php session management and control
This article will introduce the relevant content of PHP session management and control.
php session management and control
<?php setcookie('name'); setcookie('mycookie'); setcookie('mycookie',''); setcookie("mycookie",false); setcookie('mycookie','',time()-3600); echo ($HTTP_COOKIE_VARS['mycookie']); print_r($_COOKIE); ?> <?php if (($_POST['username'] != null) && ($_POST['password'] != null)) { $userName = $_POST['username']; $passWord =md5($_POST['password']); $conn = mysqli_connect('localhost', 'root', 'root'); mysqli_select_db($conn, 'test'); $sql = "select * from user where 'username = '$userName'"; $res = mysqli_query($conn, $sql); $row = mysqli_fetch_assoc($res); if ($row['password'] == $passWord) { setcookie('username',$userName,time()+60*60*24*30); setcookie('password',$passWord,time()+60*60*24*30); header('Location: welcome.php'."?username=$userName"); } } if (($_COOKIE['username'] != null) && ($_COOKIE['password'] != null)) { $userName = $_COOKIE['username']; $passWord = $_COOKIE['password']; $conn = mysqli_connect('localhost', 'root', 'root', 'test'); $res = mysqli_query($conn, "select * from user where username = '$userName'"); $row = mysqli_fetch_assoc($res); if ($row['password'] == $passWord) { header('location:welcome.php'."?username=$userName"); } } ?> <html> <head> </head> <body> <form action="" method="post"> <p> 用户名:<input type="text" name="username" /> 密码:<input type="text" name="password" /> <input type="submit" value="登录"/> </p> </form> </body> </html> <?php /* * session使用: * 1.开启session * 2.添加session * 3.读取session数据 * 4.销毁session数据 * 5.session的扩展:默认session存储位置 */ session_start(); $_SESSION=array('name' => '小明'); $_SESSION = array();//销毁 echo $_SESSION['name']; // $_SESSION['name']='小明'; // $_SESSION['name']='小明1'; // echo $_SESSION['name']; // unset($_SESSION['name']);销毁且不可逆 // echo $_SESSION['name']; ?> <?php session_start(); if (($_POST['username'] != null) && ($_POST['password'] != null)) { $userName = $_POST['username']; $passWord = $_POST['password']; $conn = mysqli_connect('localhost', 'root', 'root', 'test'); $res = mysqli_query($conn, "select * from user where username='$userName'"); $row = mysqli_fetch_assoc($res); if ($row['password'] == $passWord) { $_SESSION['username'] = $userName; $_SESSION['password'] = $passWord; header('Location:welcome.php'); } } ?> <?php session_start(); $userName = $_SESSION['username']; ?> <?php $goods = array(); $i = 0; $conn = mysqli_connect('localhost', 'root', 'root', 'test'); $res = mysqli_query($conn, 'select * from shop'); while ($row = mysqli_fetch_assoc($res)){ $goods[$i]['id'] = $row['id']; $goods[$i]['name'] = $row['name']; $goods[$i]['price'] = $row['price']; $i++; } ?> <html> <head> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <body> <?php foreach ($goods as $value){ echo '商品名'.$value['name'].'价格'.$value['price']; echo "<a href=buy.php?name=".$value['name'].'&price='. $value['price'].">购买</a>"; echo '<br />'; } ?> <a href="shoppingCart.php">查看购物车</a> </body> </html> <html> <head> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <body> <?php session_start(); $name = $_GET['name']; $price = $_GET['price']; $goods = $_SESSION['goods']; if ($name == $goods[$name]['name']) { $_SESSION['totalPrice'] += $price; $goods[$name]['number'] += 1; }else { $goods[$name]['name'] = $name; $goods[$name]['price'] = $price; $goods[$name]['number'] += 1; $_SESSION['totalPrice'] += $price; } $_SESSION['goods'] = $goods; header('location: goodsList.php'); ?> </body> </html> <html> <head> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <body> <?php session_start(); $goods = $_SESSION['goods']; echo '您购买了:<br />'; foreach ($goods as $value){ echo $value['name'].'价格'.$value['price'].'数量'.$value['number'].'<br />'; } echo '总数:'.$_SESSION['totalPrice'].'<br />'; ?> <a href="goodsList.php">返回商品列表</a> </body> </html>
This article introduces php session management and control. For more related knowledge, please pay attention to the php Chinese website.
Related recommendations:
php basic learning six: error handling
##Comparative introduction to SESSION and COOKIE under PHP
Learn how to get the client IP in php
The above is the detailed content of php session management and control. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


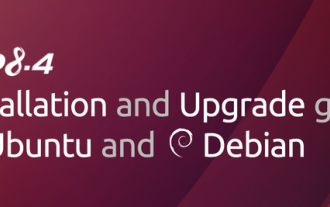
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
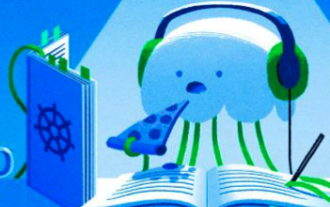
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
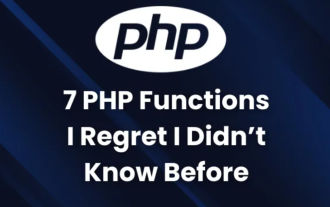
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
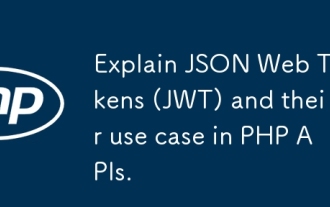
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
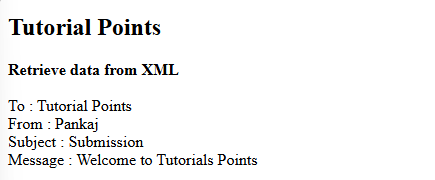
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
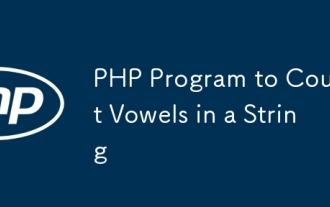
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
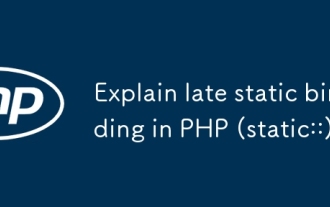
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
