A brief analysis of the use of Ajax in Asp.net MVC
In ASP.NET MVC beta, we can use Ajax.BeginForm, Ajax.ActionLink to make Ajax calls. Similarly, we can also use some Ajax-supported frameworks such as jQuery to simplify ajax calls.
1. Using System.Web.Mvc.Ajax
1.1 System.Web.Mvc.Ajax.BeginForm
1.2 System.Web.Mvc .Ajax.ActionLink
2. Manually create your own "non-intrusive" Javascript"
1. Use System.Web.Mvc.Ajax
1.1 System.Web.Mvc.Ajax.BeginForm
Step 1: Create Form## with Ajax.BeginForm
#
@using (Ajax.BeginForm( new AjaxOptions() { HttpMethod = "post", Url = @Url.Action("Index","Reviews"), InsertionMode = InsertionMode.Replace, UpdateTargetId = "restaurantList", LoadingElementId = "loding", LoadingElementDuration = 2000 })) { <input type="search" name="searchItem"/> <input type="submit" value="按名称搜索"/> }
<form id="form0" method="post" data-ajax-url="/Reviews" data-ajax-update="#restaurantList" data-ajax-mode="replace" data-ajax-method="post" data-ajax-loading-duration="2000" data-ajax-loading="#loding" data-ajax="true" action="/Reviews" novalidate="novalidate">
new AjaxOptions() { ... Url = @Url.Action("Index","Reviews") ... } public ActionResult Index(string searchKey = null) { var model = _restaurantReviews.Where(r => searchKey == null || r.Name.ToLower().Contains(searchKey.ToLower().Trim())) .OrderByDescending(r => r.Rating) .Take(100) .Select(r=>new RestaurantReview() { City = r.City, Country = r.Country, Id = r.Id, Name = r.Name, Rating = r.Rating }).ToList(); if (Request.IsAjaxRequest()) { System.Threading.Thread.Sleep(1000 * 3);//模拟处理数据需要的时间 //return View(model)会返回整个页面,所以返回部分视图。 return PartialView("_RestaurantPatialView", model); } return View(model); }
:
<p id="restaurantList">... </p>
## Step 4: (Optional) To enhance the user experience, add an AjaxOption object The ID specified by the LoadingElementid parameter is the HTML element of Loding:
CSHTML files:
r 动
#1.2 System.Web.Mvc.Ajax.ActionLink
The usage of System.Web.Mvc.Ajax.ActionLink and System.Web.Mvc.Ajax.BeginForm are basically the same
The first step: Use System.Web.Mvc.Ajax.ActionLink to create a hyperlink
new AjaxOptions() { .... LoadingElementId = "loding", LoadingElementDuration = 2000 }))
The corresponding generated final html is:
<p id="loding" hidden="hidden"> <img class="smallLoadingImg" src="@Url.Content("~/Content/images/loading.gif")" /> </p>
Step 2: Define the Action to respond to the hyperlink:
@*@Html.ActionLink(item.Name, "Details", "Reviews",new{id = item.Id},new {@class ="isStar"})*@ @*<a class="isStar" href="@Url.Action("Details","Reviews", new {id = item.Id})">@item.Name</a>*@ @*使用Ajax的超链接*@ @{ var ajaxOptions = new AjaxOptions() { HttpMethod = "post", //Url = @Url.Action(""), UpdateTargetId = "renderBody", InsertionMode = InsertionMode.Replace, LoadingElementId = "loding", LoadingElementDuration = 2000 }; @Ajax.ActionLink(item.Name, "Details", "Reviews", new { id = item.Id }, ajaxOptions, new {@class="isStar"}) }
Step 3: Define the bearer Update some html elements:
<a class="isStar" href="/Reviews/Details/1" data-ajax-update="#renderBody" data-ajax-mode="replace" data-ajax-method="post" data-ajax-loading-duration="2000" data-ajax-loading="#loding" data-ajax="true">
## Step 4: (Optional) To enhance the user experience, add the AjaxOptionsd object The Id specified by the LoadingElementId parameter is the loading html element:
/// <summary> ///关于使用System.Web.Mvc.Ajax的说明: /// Controller的Action方法: /// (1)当显式添加[HttpPost],传给System.Web.Mvc.Ajax的AjaxOptions()的HttpMethod只能为 "post", /// (2)当显式添加[HttpGet],传给System.Web.Mvc.Ajax的AjaxOptions()的HttpMethod只能为 "get", /// (3) 当都没有显式添加[HttpPost]和[HttpGet],传给System.Web.Mvc.Ajax的AjaxOptions()的HttpMethod可以为 "get"也可以为"post", /// </summary> /// <param name="id"></param> /// <returns></returns> public ActionResult Details(int id=1) { var model = (from r in _restaurantReviews where r.Id == id select r).FirstOrDefault(); if (Request.IsAjaxRequest()) { return PartialView("_RestaurantDetails", model); } return View(model); }
<p id="renderBody"> .... </p>
@* --------------------------------------------------------- 需要手工为Form添加些属性标签,用于锚点 模仿MVC框架的构建自己的“非介入式Javascript”模式 -------------------------------------------------------*@ <form method="post" action="@Url.Action("Index")" data-otf-ajax="true" data-otf-ajax-updatetarget="#restaurantList"> <input type="search" name="searchItem" /> <input type="submit" value="按名称搜索" /> </form>
JSONP for AJAX cross-domain request to obtain JSON data
Realize mobile phone positioning based on h5 ajax
Use the H5 feature FormData to upload files without refreshing
The above is the detailed content of A brief analysis of the use of Ajax in Asp.net MVC. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
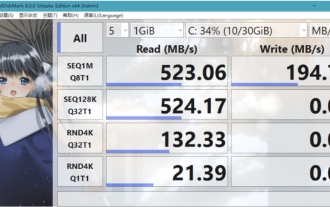
CrystalDiskMark is a small HDD benchmark tool for hard drives that quickly measures sequential and random read/write speeds. Next, let the editor introduce CrystalDiskMark to you and how to use crystaldiskmark~ 1. Introduction to CrystalDiskMark CrystalDiskMark is a widely used disk performance testing tool used to evaluate the read and write speed and performance of mechanical hard drives and solid-state drives (SSD). Random I/O performance. It is a free Windows application and provides a user-friendly interface and various test modes to evaluate different aspects of hard drive performance and is widely used in hardware reviews
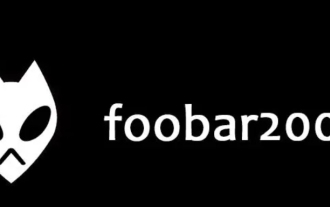
foobar2000 is a software that can listen to music resources at any time. It brings you all kinds of music with lossless sound quality. The enhanced version of the music player allows you to get a more comprehensive and comfortable music experience. Its design concept is to play the advanced audio on the computer The device is transplanted to mobile phones to provide a more convenient and efficient music playback experience. The interface design is simple, clear and easy to use. It adopts a minimalist design style without too many decorations and cumbersome operations to get started quickly. It also supports a variety of skins and Theme, personalize settings according to your own preferences, and create an exclusive music player that supports the playback of multiple audio formats. It also supports the audio gain function to adjust the volume according to your own hearing conditions to avoid hearing damage caused by excessive volume. Next, let me help you
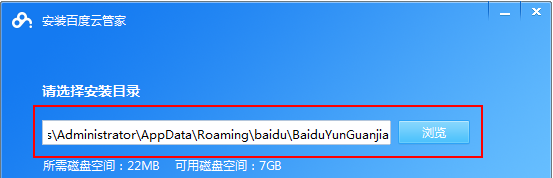
Cloud storage has become an indispensable part of our daily life and work nowadays. As one of the leading cloud storage services in China, Baidu Netdisk has won the favor of a large number of users with its powerful storage functions, efficient transmission speed and convenient operation experience. And whether you want to back up important files, share information, watch videos online, or listen to music, Baidu Cloud Disk can meet your needs. However, many users may not understand the specific use method of Baidu Netdisk app, so this tutorial will introduce in detail how to use Baidu Netdisk app. Users who are still confused can follow this article to learn more. ! How to use Baidu Cloud Network Disk: 1. Installation First, when downloading and installing Baidu Cloud software, please select the custom installation option.
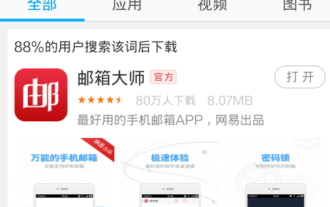
NetEase Mailbox, as an email address widely used by Chinese netizens, has always won the trust of users with its stable and efficient services. NetEase Mailbox Master is an email software specially created for mobile phone users. It greatly simplifies the process of sending and receiving emails and makes our email processing more convenient. So how to use NetEase Mailbox Master, and what specific functions it has. Below, the editor of this site will give you a detailed introduction, hoping to help you! First, you can search and download the NetEase Mailbox Master app in the mobile app store. Search for "NetEase Mailbox Master" in App Store or Baidu Mobile Assistant, and then follow the prompts to install it. After the download and installation is completed, we open the NetEase email account and log in. The login interface is as shown below
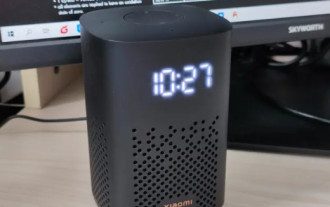
After long pressing the play button of the speaker, connect to wifi in the software and you can use it. Tutorial Applicable Model: Xiaomi 12 System: EMUI11.0 Version: Xiaoai Classmate 2.4.21 Analysis 1 First find the play button of the speaker, and press and hold to enter the network distribution mode. 2 Log in to your Xiaomi account in the Xiaoai Speaker software on your phone and click to add a new Xiaoai Speaker. 3. After entering the name and password of the wifi, you can call Xiao Ai to use it. Supplement: What functions does Xiaoai Speaker have? 1 Xiaoai Speaker has system functions, social functions, entertainment functions, knowledge functions, life functions, smart home, and training plans. Summary/Notes: The Xiao Ai App must be installed on your mobile phone in advance for easy connection and use.
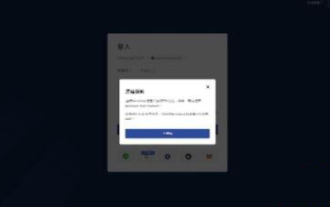
MetaMask (also called Little Fox Wallet in Chinese) is a free and well-received encryption wallet software. Currently, BTCC supports binding to the MetaMask wallet. After binding, you can use the MetaMask wallet to quickly log in, store value, buy coins, etc., and you can also get 20 USDT trial bonus for the first time binding. In the BTCCMetaMask wallet tutorial, we will introduce in detail how to register and use MetaMask, and how to bind and use the Little Fox wallet in BTCC. What is MetaMask wallet? With over 30 million users, MetaMask Little Fox Wallet is one of the most popular cryptocurrency wallets today. It is free to use and can be installed on the network as an extension
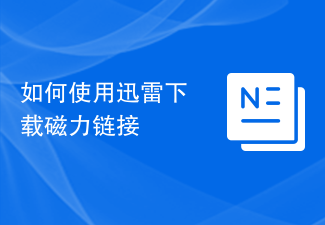
With the rapid development of network technology, our lives have also been greatly facilitated, one of which is the ability to download and share various resources through the network. In the process of downloading resources, magnet links have become a very common and convenient download method. So, how to use Thunder magnet links? Below, I will give you a detailed introduction. Xunlei is a very popular download tool that supports a variety of download methods, including magnet links. A magnet link can be understood as a download address through which we can obtain relevant information about resources.
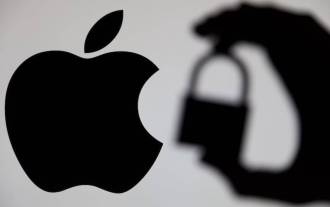
Apple rolled out the iOS 17.4 update on Tuesday, bringing a slew of new features and fixes to iPhones. The update includes new emojis, and EU users will also be able to download them from other app stores. In addition, the update also strengthens the control of iPhone security and introduces more "Stolen Device Protection" setting options to provide users with more choices and protection. "iOS17.3 introduces the "Stolen Device Protection" function for the first time, adding extra security to users' sensitive information. When the user is away from home and other familiar places, this function requires the user to enter biometric information for the first time, and after one hour You must enter information again to access and change certain data, such as changing your Apple ID password or turning off stolen device protection.
