How to develop a custom library
This time I will show you how to develop a custom library and what are the precautions for developing a custom library. The following is a practical case, let's take a look.
Of course, this library is still in its infancy, and the function implementation is relatively simple. It is published here. Everyone is welcome to use this as a basis to improve it. Project address: Hoz.js, everyone is welcome to start, fork, and raise issues.
Features
Declarative template syntax
-
Learn from redux, flux, etc., and introduce state management
Introducing virtual dom, diff algorithm to improve performance
Declarative syntax
<p id = "app"> <p> <img src="{{moveImage}}" /> <p>{{moveName}}</p> </p> </p>
var hoz = new Hoz('app', state, changeStore) var state = { moveName: '', moveImage: '' } function changeStore (state, action) { switch (action.type) { case 'SET_NAME': { state.moveName = action.data break } case 'SET_IMAGE': { state.moveImage = action.data break } } } hoz.store.dispatch({ type: 'SET_NAME', data: '后来的我们' })
This is a hoz application, through a concise template Syntax declaratively renders data into the DOM system.
And everything is responsive.
Draw on the ideas of redux, flux, etc., and introduce state management
Draw on the ideas of redux in terms of state management, and realize the management of one-way data flow.
Mainly defines the four concepts of state, action, changeStore, and dispatch.
state
Store data
var state = { moveName: '', moveImage: '' }
changeStore
Equivalent to the reducer in redux, which stores all operations on data
function changeStore (state, action) { switch (action.type) { case 'SET_NAME': { state.moveName = action.data break } case 'SET_IMAGE': { state.moveImage = action.data break } } }
Receive the action, execute the corresponding method, and modify the data in the state. Different from redux, redux puts back a new state, and it directly operates the current state, because the data in the state has been tracked through the Object.defineProperty method, which will be discussed later.
action and dispatch
When we want to modify the data, we must modify the state in the changeStore by submitting the action
hoz.store.dispatch({ type: 'SET_NAME', data: '后来的我们' })
This is hoz State management strategy
view -> dispatch -> action -> changeStore -> state -> view
Benefits
As applications become increasingly complex and the amount of data increases, it is very difficult to manage the changing status without corresponding data management. It is difficult to observe when and for what reason the state changes.
This means that all data in the application follows the same lifecycle, making the application more predictable and easier to understand.
We can see all the changes that can occur in the state from the changeStore
The only way to modify the state is to submit an action to the changeStore, so Every change in the data will flow through one place, which facilitates our debugging and other operations.
Introducing virtual dom and diff algorithm
As we all know, due to the large size of dom elements and dom operationsit is easy to cause page rearrangement , the performance of directly operating dom is very, very poor.
So hoz introduced the virtual dom algorithm and used native JavaScript objects to map dom objects, because the operation of native JavaScript objects is faster and simpler.
How to map? For example,
class VNode { constructor (sel, tagName, id, className, el, children, data, text, key) { this.tagName = tagName // p this.sel = sel // p#id.class this.id = id // id this.className = className // [] this.children = children // [] this.el = el // node this.data = data // {} this.key = key this.text = text } } export default VNode
is just a JavaScript object, representing a dom element.
I create a virtual dom tree based on the element pointed to by the id passed in the hoz constructor as the root element. When the data changes, the dom is not directly manipulated, but virtual Perform operations and modifications on the dom tree. Then the diff algorithm is used to compare the old and new virtual DOM trees, and the real DOM is modified in the smallest unit.
Data Responsiveness Principle
How does hoz achieve data responsiveness? A publish/subscribe mode is mainly implemented here by using the Object.defineProperty method. The getter and setter properties of the data in the state are modified through Object.defineProperty. When rendering for the first time, the corresponding subscribers are added to a topic object in the getter. Go, when the data changes, call the notify method of the topic object corresponding to the data in the setter to publish a message to notify each subscriber of the update.
state -> data -> publisher 一对多的关系 | Dep | view -> {{data}} -> subscribers
I hope everyone can use this as a basis to improve it together. Project address: Hoz.js, everyone is welcome to start, fork, and raise issues.
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the php Chinese website!
Recommended reading:
Front-end, HTT, computer and network
Detailed explanation of webkit-font-smoothing font anti-aliasing rendering use cases
The above is the detailed content of How to develop a custom library. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
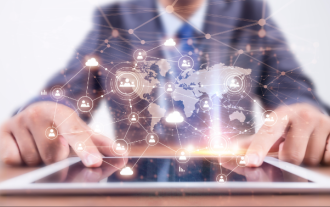
This AI-assisted programming tool has unearthed a large number of useful AI-assisted programming tools in this stage of rapid AI development. AI-assisted programming tools can improve development efficiency, improve code quality, and reduce bug rates. They are important assistants in the modern software development process. Today Dayao will share with you 4 AI-assisted programming tools (and all support C# language). I hope it will be helpful to everyone. https://github.com/YSGStudyHards/DotNetGuide1.GitHubCopilotGitHubCopilot is an AI coding assistant that helps you write code faster and with less effort, so you can focus more on problem solving and collaboration. Git
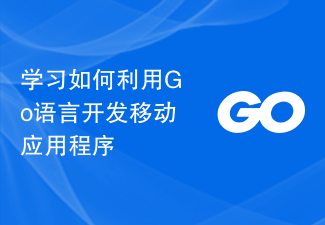
Go language development mobile application tutorial As the mobile application market continues to boom, more and more developers are beginning to explore how to use Go language to develop mobile applications. As a simple and efficient programming language, Go language has also shown strong potential in mobile application development. This article will introduce in detail how to use Go language to develop mobile applications, and attach specific code examples to help readers get started quickly and start developing their own mobile applications. 1. Preparation Before starting, we need to prepare the development environment and tools. head
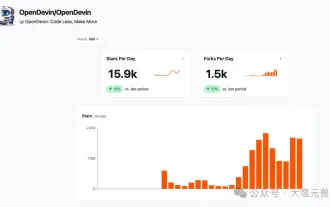
On March 3, 2022, less than a month after the birth of the world's first AI programmer Devin, the NLP team of Princeton University developed an open source AI programmer SWE-agent. It leverages the GPT-4 model to automatically resolve issues in GitHub repositories. SWE-agent's performance on the SWE-bench test set is similar to Devin, taking an average of 93 seconds and solving 12.29% of the problems. By interacting with a dedicated terminal, SWE-agent can open and search file contents, use automatic syntax checking, edit specific lines, and write and execute tests. (Note: The above content is a slight adjustment of the original content, but the key information in the original text is retained and does not exceed the specified word limit.) SWE-A
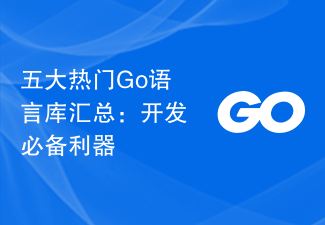
Summary of the five most popular Go language libraries: essential tools for development, requiring specific code examples. Since its birth, the Go language has received widespread attention and application. As an emerging efficient and concise programming language, Go's rapid development is inseparable from the support of rich open source libraries. This article will introduce the five most popular Go language libraries. These libraries play a vital role in Go development and provide developers with powerful functions and a convenient development experience. At the same time, in order to better understand the uses and functions of these libraries, we will explain them with specific code examples.
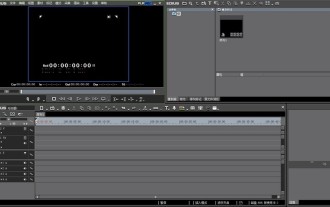
1. The picture below is the default screen layout of edius. The default EDIUS window layout is a horizontal layout. Therefore, in a single-monitor environment, many windows overlap and the preview window is in single-window mode. 2. You can enable [Dual Window Mode] through the [View] menu bar to make the preview window display the playback window and recording window at the same time. 3. You can restore the default screen layout through [View menu bar>Window Layout>General]. In addition, you can also customize the layout that suits you and save it as a commonly used screen layout: drag the window to a layout that suits you, then click [View > Window Layout > Save Current Layout > New], and in the pop-up [Save Current Layout] Layout] enter the layout name in the small window and click OK
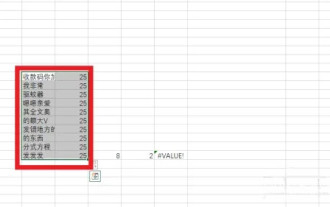
In an excel table, sometimes you may need to insert coordinate axes to see the changing trend of the data more intuitively. Some friends still don’t know how to insert coordinate axes in the table. Next, I will share with you how to customize the coordinate axis scale in Excel. Coordinate axis insertion method: 1. In the excel interface, select the data. 2. In the insertion interface, click to insert a column chart or bar chart. 3. In the expanded interface, select the graphic type. 4. In the right-click interface of the table, click Select Data. 5. In the expanded interface, you can customize it.
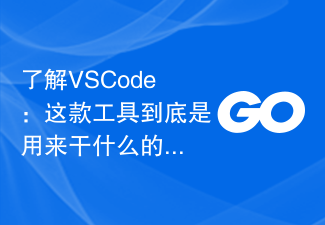
"Understanding VSCode: What is this tool used for?" 》As a programmer, whether you are a beginner or an experienced developer, you cannot do without the use of code editing tools. Among many editing tools, Visual Studio Code (VSCode for short) is very popular among developers as an open source, lightweight, and powerful code editor. So, what exactly is VSCode used for? This article will delve into the functions and uses of VSCode and provide specific code examples to help readers
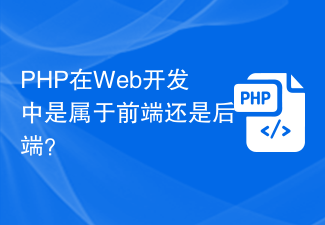
PHP belongs to the backend in web development. PHP is a server-side scripting language, mainly used to process server-side logic and generate dynamic web content. Compared with front-end technology, PHP is more used for back-end operations such as interacting with databases, processing user requests, and generating page content. Next, specific code examples will be used to illustrate the application of PHP in back-end development. First, let's look at a simple PHP code example for connecting to a database and querying data:
