


Using Elememt-UI to build the management backend in Vue (detailed tutorial)
This article shares with you in detail the process of building a management backend with Vue Elememt-UI and related code examples, please refer to it and learn together.
Installation
I am using vue-cli to initialize the project. The command is as follows:
npm i -g vue-cli mkdir my-project && cd my-project vue init webpack
Modify the package.json file:
... "dependencies": { "vue": "^2.5.2", "vue-router": "^3.0.1", "element-ui": "^2.0.7", // element-ui "axios": "^0.17.1" // http 请求库 } ...
Then execute npm install to install the dependencies. If the installation speed is a bit slow, you can try cnpm and find out the specific installation and usage by yourself.
Briefly introduce the directory structure of the project:
├─build // 构建配置 ├─config // 配置文件 ├─src // vue 开发源文件目录 ├────assets // css/js 文件 ├────components // vue 组件 ├────router // 路由 ├────App.vue // 启动组件 ├────main.js // 入口文件 ├─static // 静态文件目录 ├─test // 测试目录
Then execute npm run dev in the project root directory, open the browser and enter http://localhost:8080 to view it.
Goal
Login page, login, exit function
Home page, call interface rendering list
Routing
Routing uses vue-router. For specific usage, please refer to the official documentation
We are here Two routes are required:
src/router/index.js
import Vue from 'vue' import Router from 'vue-router' import Index from '@/components/Index' import Login from '@/components/Login' Vue.use(Router) const routers = new Router({ routes: [ { path: '/index', name: 'index', component: Index }, { path: '/login', name: 'login', component: Login } ] }) routers.beforeEach((to, from, next) => { if (to.name !== 'login' && !localStorage.getItem('token')) { next({path: 'login'}) } else { next() } }) export default routers
Login page
src/components/Login .vue
<template> <p class="login"> <el-form name="aa" :inline="true" label-position="right" label-width="80px"> <el-form-item label="用户名"> <el-input v-model="user.name"></el-input> </el-form-item> <el-form-item label="密码"> <el-input type="password" v-model="user.password"></el-input> </el-form-item> <el-form-item label=" "> <el-button type="primary" @click="login()">登录</el-button> </el-form-item> </el-form> </p> </template> <script> import $http from '@/api/' import config from '@/config' export default { data () { return { user: { name: '', password: '' } } }, mounted: function () { var token = localStorage.getItem('token') if (token) { this.$router.push('/index') } }, methods: { login: function () { var data = { grant_type: 'password', client_id: config.oauth_client_id, client_secret: config.oauth_secret, username: this.user.name, password: this.user.password } var _this = this $http.login(data).then(function (res) { if (res.status === 200) { $http.setToken(res.data.access_token) _this.$message({ showClose: false, message: '登录成功', type: 'success' }) _this.$router.push('/index') } else { _this.$message({ showClose: false, message: '登录失败', type: 'error' }) } }) } } } </script> <style> .login{ width: 300px; margin: 100px auto; background-color: #ffffff; padding: 30px 30px 5px; border-radius: 5px; } </style>
首页
src/components/Index.vue
<template> <p class="main"> <el-table stripe v-loading="loading" element-loading-background="#dddddd" :data="tableData" style="width: 100%"> <el-table-column prop="id" label="ID"> </el-table-column> <el-table-column prop="name" label="名称"> </el-table-column> </el-table> <el-pagination background layout="prev, pager, next" :total="total" class="page" @current-change="pageList"> </el-pagination> </p> </template> <script> import $http from '@/api/' export default { data () { return { tableData: [], total: 0, loading: false } }, mounted: function () { this.getList() }, methods: { pageList: function (page) { this.search.page = page this.getList() }, getList: function () { var _this = this _this.loading = true $http.index().then(function (res) { if (res.status === 200) { _this.tableData = res.data.data.lists _this.total = res.data.data.total } _this.loading = false }) } } } </script>
App
src/App.vue
<template> <p id="app"> <el-row v-if="token"> <menus class="left-menu"> <h3 class="logo"><a href="/" rel="external nofollow" >Admin</a></h3> </menus> <el-col :span="21" :gutter="0" :offset="3"> <el-breadcrumb separator-class="el-icon-arrow-right" class="breadcrumb"> <el-breadcrumb-item :to="{ path: '/' }">首页</el-breadcrumb-item> <el-breadcrumb-item class="active">列表</el-breadcrumb-item> </el-breadcrumb> <el-dropdown @command="operate" class="header"> <img src="/static/image/head.jpg" /> <el-dropdown-menu slot="dropdown" :click="true"> <el-dropdown-item command="/user/profile">基本资料</el-dropdown-item> <el-dropdown-item command="/logout">安全退出</el-dropdown-item> </el-dropdown-menu> </el-dropdown> <router-view/> </el-col> <el-col :span="21" :gutter="0" :offset="3" class="footer">Copyright © 2017 Flyerboy All Rights Reserved</el-col> </el-row> <router-view v-if="!token" /> </p> </template> <script> import Menus from '@/components/Menu' export default { name: 'App', data () { return { token: false } }, mounted: function () { this.token = localStorage.getItem('token') ? true : false }, watch: { '$route.path': function ($newVal, $oldVal) { this.token = localStorage.getItem('token') ? true : false } }, methods: { operate: function (command) { if (command === '/logout') { localStorage.removeItem('token') this.$router.push('login') } else { this.$router.push(command) } } }, components: { Menus } } </script> <style> body{ margin: 0; padding: 0; background-color: #eeeeee; } .header{ position: absolute; top: 5px; right: 20px; } .header img{ width: 38px; height: 38px; border-radius: 20px; border: 1px solid #aaaaaa; } #app { font-family: 'Avenir', Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; } .main{ padding: 20px; min-height: 600px; margin-bottom: 20px; } .main table{ background: #ffffff; } .left-menu{ background-color: #33374B; } .logo{ padding: 20px 0 15px 20px; font-size: 24px; border-bottom: 2px solid #3a8ee6; } .logo a{ color: #ffffff; text-decoration: none; } .left-menu .el-menu{ border-right: 0; } .breadcrumb{ line-height: 40px; padding: 5px 20px; background: #ffffff; } .breadcrumb span{ color: #069; font-weight: normal; } .breadcrumb .active{ color: #aaaaaa; } .page{ margin: 20px 0 0; margin-left: -10px; } .page .el-pager li.number{ background-color: #ffffff; } .el-submenu .el-menu-item{ padding-left: 60px !important; } .footer{ position: fixed; bottom: 0; right: 0; font-size: 12px; color: #888888; padding: 15px 20px; text-align: center; background-color: #ffffff; margin-top: 40px; } </style>
Call API
import axios from 'axios' axios.defaults.baseURL = 'http://localhost:8000/' axios.defaults.headers.post['Content-Type'] = 'application/x-www-form-urlencoded' axios.defaults.headers.common['Authorization'] = 'Bearer ' + localStorage.getItem('token') export default { setToken: function (token) { localStorage.setItem('token', token) axios.defaults.headers.common['Authorization'] = 'Bearer ' + token }, login: function (param) { return axios.post('oauth/token', param) }, index: function (params) { return axios.get('api/user/list', { params: params }) } }
config
export default { oauth_client_id: 2, oauth_secret: '' }
main.js
import Vue from 'vue' import App from './App' import router from './router' import ElementUI from 'element-ui' import 'element-ui/lib/theme-chalk/index.css' Vue.use(ElementUI) Vue.config.productionTip = false new Vue({ el: '#app', router, components: { App }, template: '<App/>' })
How to use vue to convert timestamps into custom time formats
Using vue and element -UIHow to implement table content paging
Detailed explanation of FastClick source code (detailed tutorial)
The above is the detailed content of Using Elememt-UI to build the management backend in Vue (detailed tutorial). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
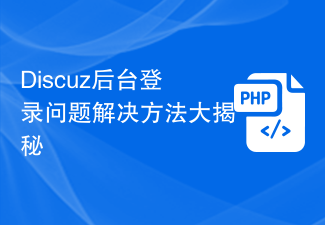
The solution to the Discuz background login problem is revealed. Specific code examples are needed. With the rapid development of the Internet, website construction has become more and more common, and Discuz, as a commonly used forum website building system, has been favored by many webmasters. However, precisely because of its powerful functions, sometimes we encounter some problems when using Discuz, such as background login problems. Today, we will reveal the solution to the Discuz background login problem and provide specific code examples. We hope to help those in need.
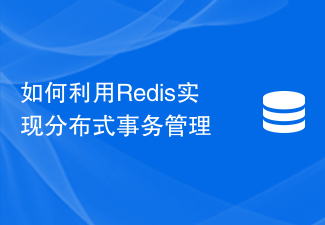
How to use Redis to implement distributed transaction management Introduction: With the rapid development of the Internet, the use of distributed systems is becoming more and more widespread. In distributed systems, transaction management is an important challenge. Traditional transaction management methods are difficult to implement in distributed systems and are inefficient. Using the characteristics of Redis, we can easily implement distributed transaction management and improve the performance and reliability of the system. 1. Introduction to Redis Redis is a memory-based data storage system with efficient read and write performance and rich data
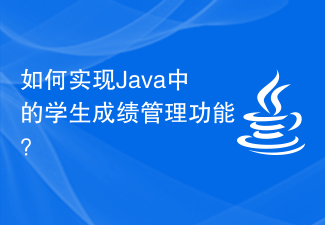
How to implement student performance management function in Java? In the modern education system, student performance management is a very important task. By managing student performance, schools can better monitor students' learning progress, understand their weaknesses and strengths, and make more targeted teaching plans based on this information. In this article, we will discuss how to use Java programming language to implement student performance management functions. First, we need to determine the data structure of student grades. Typically, student grades can be represented as a
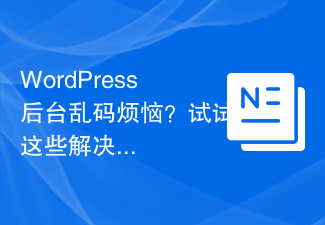
Are you worried about WordPress backend garbled code? Try these solutions, specific code examples are required. With the widespread application of WordPress in website construction, many users may encounter the problem of garbled code in the WordPress backend. This kind of problem will cause the background management interface to display garbled characters, causing great trouble to users. This article will introduce some common solutions to help users solve the trouble of garbled characters in the WordPress backend. Modify the wp-config.php file and open wp-config.

Laravel extension package management: Easily integrate third-party code and functions Introduction: In Laravel development, we often use third-party code and functions to improve the efficiency and stability of the project. The Laravel extension package management system allows us to easily integrate these third-party codes and functions, making our development work more convenient and efficient. This article will introduce the basic concepts and usage of Laravel extension package management, and use some practical code examples to help readers better understand and apply it. What is Lara
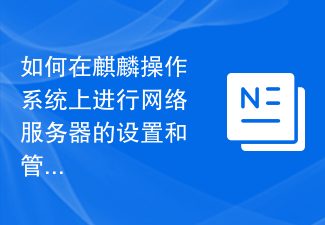
How to set up and manage the network server on Kirin operating system? Kirin operating system is a Linux-based operating system independently developed in China. It has the characteristics of open source, security and stability, and has been widely used in China. This article will introduce how to set up and manage network servers on Kirin operating system, helping readers better build and manage their own network servers. 1. Install related software Before starting to set up and manage the network server, we need to install some necessary software. On Kirin OS, you can
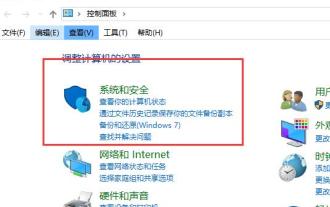
When we use the win10 system, when we use the mouse to right-click the desktop or the right-click menu, we find that the menu cannot be opened and we cannot use the computer normally. At this time, we need to restore the system to solve the problem. Win10 right-click menu management cannot be opened: 1. First open our control panel, and then click. 2. Then click under Security and Maintenance. 3. Click on the right to restore the system. 4. If it still cannot be used, check whether there is something wrong with the mouse itself. 5. If you are sure there is no problem with the mouse, press + and enter. 6. After the execution is completed, restart the computer.
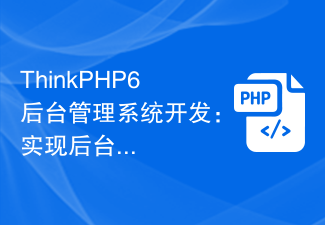
ThinkPHP6 backend management system development: Implementing backend functions Introduction: With the continuous development of Internet technology and market demand, more and more enterprises and organizations need an efficient, safe, and flexible backend management system to manage business data and conduct operational management. This article will use the ThinkPHP6 framework to demonstrate through examples how to develop a simple but practical backend management system, including basic functions such as permission control, data addition, deletion, modification and query. Environment preparation Before starting, we need to install PHP, MySQL, Com
