9 steps to operate mongodb with Python
This article shares with you the detailed steps and example codes for operating mongodb in Python. Friends in need can refer to it.
1 Import pymongo
from pymongo import MongoClient
2 Connect to the server port number 27017
Connect to MongoDB
To connect to MongoDB we need to use the MongoClient in the PyMongo library. Generally speaking, just pass in the IP and port of MongoDB. The first parameter is the address host, and the second parameter is the port. If the port is not passed, the default is 27017.
conn = MongoClient("localhost") MongoClient(host='127.0.0.1',port=27017)
Three connection database
db = conn.Database name
Connection collection
collection = db[collection_name] or collection = db.collection_name
View all collection names
db.collection_names()
Four Insert Data
(1) Insert one piece of data
db.user.insert({"name":"夏利刚","age":18,"hobby":"学习"})
(2) Insert multiple pieces of data
db.user.insert([{"name":"夏利刚","age":18,"hobby":"学习"},{"name":"xxxoo","age":48,"hobby":"学习"}]
(3) It is recommended to use
insert_one 插入一条数据 insert_many() 插入多条数据
data.inserted_id data.inserted_ids
(1) Query all
#带条件的查询 # data = db.user.find({"name":"周日"}) # print(data) #返回result类似一个迭代器 可以使用 next方法 一个一个 的取出来 # print(next(data)) #取出一条数据
db.user.find_one()
db.user.find({"name":"张三"})
from bson.objectid import ObjectId*#用于ID查询 data = db.user.find({"_id":ObjectId("59a2d304b961661b209f8da1")})
(1){"name":{'$regex':"张"}} (2)import re {'xxx':re.compile('xxx')}
(1) sort sort
data = db.user.find({"age":{"$gt":10}}).sort("age",-1) #年龄 升序 查询 pymongo.ASCENDING --升序 data = db.user.find({"age":{"$gt":10}}).sort("age",1) #年龄 降序 查询 pymongo.DESCENDING --降序
db.user.find().limit(3) data = db.user.find({"age":{"$gt":10}}).sort("age",-1).limit(3)
The update() method is actually a method that is not officially recommended. Here it is also divided into update_one() method and update_many() method. The usage is more strict,
db.user.update({"name":"张三"},{"$set":{"age":25}}) db.user.update({"name":"张三"},{"$inc":{"age":25}})
db.user.update_one({"name":"张三"},{"$set":{"age":99}})
db.user.update_many({"name":"张三"},{"$set":{"age":91}})
collection.remove({"name":"lilei"})
collection.remove()
delete_one()即删除第一条符合条件的数据 collection.delete_one({“name”:“ Kevin”}) delete_many()即删除所有符合条件的数据,返回结果是DeleteResult类型 collection.delete_many({“age”: {$lt:25}})
result.deleted_count
conn.close()
How to operate MongoDB with PHP and simple analysis
The above is the detailed content of 9 steps to operate mongodb with Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


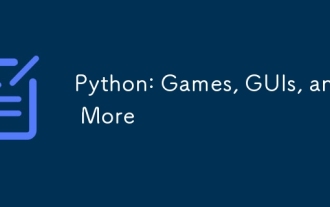
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
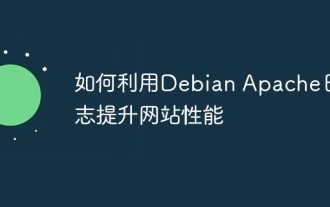
This article will explain how to improve website performance by analyzing Apache logs under the Debian system. 1. Log Analysis Basics Apache log records the detailed information of all HTTP requests, including IP address, timestamp, request URL, HTTP method and response code. In Debian systems, these logs are usually located in the /var/log/apache2/access.log and /var/log/apache2/error.log directories. Understanding the log structure is the first step in effective analysis. 2. Log analysis tool You can use a variety of tools to analyze Apache logs: Command line tools: grep, awk, sed and other command line tools.
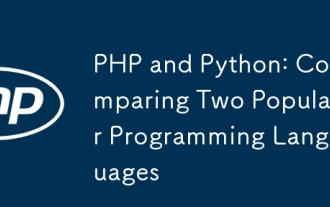
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
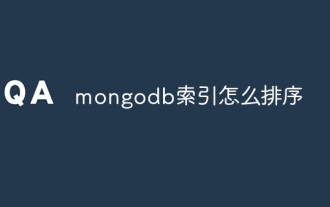
Sorting index is a type of MongoDB index that allows sorting documents in a collection by specific fields. Creating a sort index allows you to quickly sort query results without additional sorting operations. Advantages include quick sorting, override queries, and on-demand sorting. The syntax is db.collection.createIndex({ field: <sort order> }), where <sort order> is 1 (ascending order) or -1 (descending order). You can also create multi-field sorting indexes that sort multiple fields.
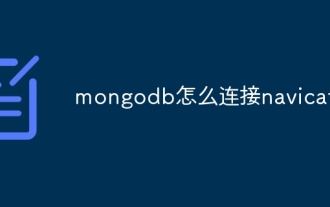
To connect to MongoDB with Navicat: Install Navicat and create a MongoDB connection; enter the server address in the host, enter the port number in the port, and enter the MongoDB authentication information in the user name and password; test the connection and save; Navicat will connect to the MongoDB server.
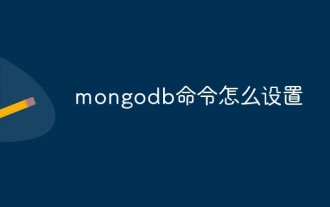
To set up a MongoDB database, you can use the command line (use and db.createCollection()) or the mongo shell (mongo, use and db.createCollection()). Other setting options include viewing database (show dbs), viewing collections (show collections), deleting database (db.dropDatabase()), deleting collections (db.&lt;collection_name&gt;.drop()), inserting documents (db.&lt;collecti
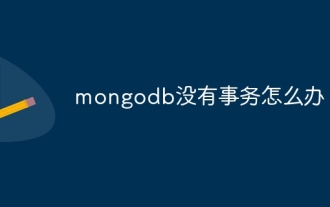
MongoDB lacks transaction mechanisms, which makes it unable to guarantee the atomicity, consistency, isolation and durability of database operations. Alternative solutions include verification and locking mechanisms, distributed transaction coordinators, and transaction engines. When choosing an alternative solution, its complexity, performance, and data consistency requirements should be considered.
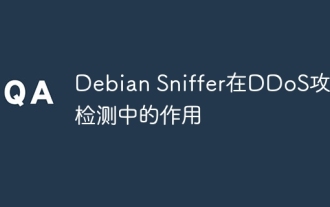
This article discusses the DDoS attack detection method. Although no direct application case of "DebianSniffer" was found, the following methods can be used for DDoS attack detection: Effective DDoS attack detection technology: Detection based on traffic analysis: identifying DDoS attacks by monitoring abnormal patterns of network traffic, such as sudden traffic growth, surge in connections on specific ports, etc. This can be achieved using a variety of tools, including but not limited to professional network monitoring systems and custom scripts. For example, Python scripts combined with pyshark and colorama libraries can monitor network traffic in real time and issue alerts. Detection based on statistical analysis: By analyzing statistical characteristics of network traffic, such as data
