Summary and answers to JS concept questions
This time I will bring you a summary and Q&A of JS concept questions. What are the precautions when using JS concept questions? The following is a practical case, let’s take a look.
Q: Describe inheritance and prototype chain in JavaScript and give examples.
JavaScript is a prototype-based object-oriented language and does not have a traditional class-based inheritance system.
In JS, each object internally refers to an object called prototype, and this prototype object itself also refers to its own prototype object, and so on. This forms a prototype reference chain, and the end of this chain is an object with null as the prototype. JS implements inheritance through the prototype chain. When an object references a property that does not belong to itself, the prototype chain will be traversed until the referenced property is found (or directly found at the end of the chain, in which case the property is not definition).
A simple example:
function Animal() { this.eatsVeggies = true; this.eatsMeat = false; }function Herbivore() {} Herbivore.prototype = new Animal();function Carnivore() { this.eatsMeat = true; } Carnivore.prototype = new Animal();var rabbit = new Herbivore();var bear = new Carnivore();console.log(rabbit.eatsMeat); // logs "false"console.log(bear.eatsMeat); // logs "true"
Q: In the following code snippet, what will alert display? Please explain your answer.
var foo = new Object();var bar = new Object();var map = new Object(); map[foo] = "foo"; map[bar] = "bar"; alert(map[foo]); // what will this display??
Here alert will pop up bar. The JS object is essentially a key-value hash table, where the key is always a string. In fact, when an object other than a string is used as a key, no error occurs. JS will implicitly convert it to a string and use that value as the key.
So, when the map object in the above code uses the foo object as the key, it will automatically call the toString() method of the foo object, and its default implementation will be called here. You will get the string "[object Object]". Then look at the above code and explain it as follows:
var foo = new Object(); var bar = new Object(); var map = new Object(); map[foo] = "foo"; // --> map["[Object object]"] = "foo"; map[bar] = "bar"; // --> map["[Object object]"] = "bar"; // NOTE: second mapping REPLACES first mapping! alert(map[foo]); // --> alert(map["[Object object]"]); // and since map["[Object object]"] = "bar", // this will alert "bar", not "foo"!! // SURPRISE! ;-)
Q: Please explain closures in JavaScript. What is a closure? What unique properties do they have? How and why do you use them? Please give an example.
A closure is a function that contains all variables or other functions that are in scope when the closure is created. In JavaScript, closures are implemented in the form of "inner functions", which are functions defined within the body of another function. Here is a simple example:
(function outerFunc(outerArg) { var outerVar = 3; (function middleFunc(middleArg) { var middleVar = 4; (function innerFunc(innerArg) { var innerVar = 5; // EXAMPLE OF SCOPE IN CLOSURE: // Variables from innerFunc, middleFunc, and outerFunc, // as well as the global namespace, are ALL in scope here. console.log("outerArg="+outerArg+ " middleArg="+middleArg+ " innerArg="+innerArg+"\n"+ " outerVar="+outerVar+ " middleVar="+middleVar+ " innerVar="+innerVar); // --------------- THIS WILL LOG: --------------- // outerArg=123 middleArg=456 innerArg=789 // outerVar=3 middleVar=4 innerVar=5 })(789); })(456); })(123);
An important feature of closures is that the inner function can still access the variables of the outer function even after the outer function returns. This is because, in JavaScript, when functions are executed, they still use the scope that was in effect when the function was created.
However, confusion can result if the inner function accesses the value of the outer function variable when it is called (rather than when it is created). To test the candidate's understanding of this nuance, use the following code snippet, which will dynamically create five buttons and ask the candidate what will be displayed when the user clicks the third button:
function addButtons(numButtons) { for (var i = 0; i < numButtons; i++) { var button = document.createElement('input'); button.type = 'button'; button.value = 'Button ' + (i + 1); button.onclick = function() { alert('Button ' + (i + 1) + ' clicked'); }; document.body.appendChild(button); document.body.appendChild(document.createElement('br')); } }window.onload = function() { addButtons(5); };
Many people will incorrectly answer that when the user clicks the third button, it will show "Button 3 clicked". In fact, the above code contains a bug (based on a misunderstanding of closure) that will display "Button 6 clicked" when the user clicks any of the five buttons. This is because, by the time the onclick method is called (for any button), the for loop has completed and the value of variable i is already 5.
You can next ask the candidate how to fix the error in the above code so that it produces the expected behavior (i.e. clicking button n will display "Button n clicked"). If the candidate can give the correct answer, it means that they know how to use closures correctly, as shown below:
function addButtons(numButtons) { for (var i = 0; i < numButtons; i++) { var button = document.createElement('input'); button.type = 'button'; button.value = 'Button ' + (i + 1); // HERE'S THE FIX: // Employ the Immediately-Invoked Function Expression (IIFE) // pattern to achieve the desired behavior: button.onclick = function(buttonIndex) { return function() { alert('Button ' + (buttonIndex + 1) + ' clicked'); }; }(i); document.body.appendChild(button); document.body.appendChild(document.createElement('br')); } }window.onload = function() { addButtons(5); };
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related topics on the PHP Chinese website article!
Recommended reading:
How to use li for horizontal arrangement
How to operate the page, visual area, and screen widths High attributes
The above is the detailed content of Summary and answers to JS concept questions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


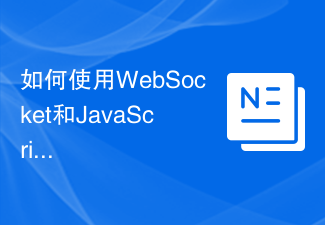
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
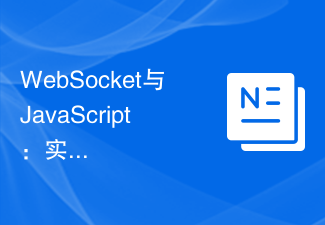
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
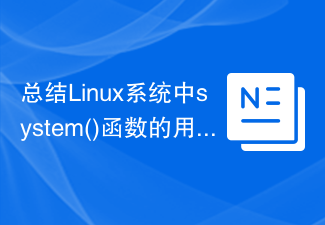
Summary of the system() function under Linux In the Linux system, the system() function is a very commonly used function, which can be used to execute command line commands. This article will introduce the system() function in detail and provide some specific code examples. 1. Basic usage of the system() function. The declaration of the system() function is as follows: intsystem(constchar*command); where the command parameter is a character.
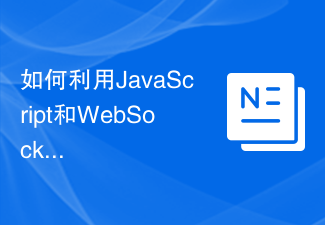
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
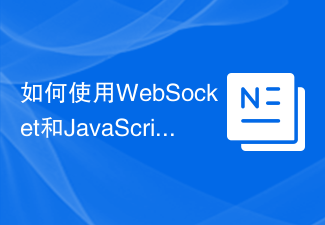
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
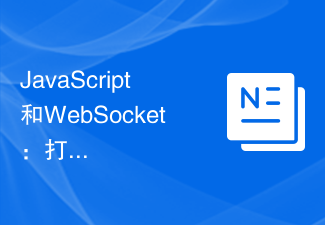
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
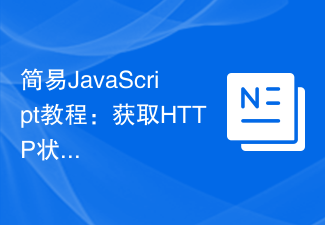
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
