Detailed explanation of using jest to test react native components
This article mainly introduces in detail how to use jest to test react native components in the project. Now I will share it with you and give you a reference.
There are currently many Javascript testing tools, but for the React testing strategy, the standard testing tool for ReactJs launched by Facebook is Jest.Jest’s official website address: https://facebook.github.io/jest/. We can see that the Jest official website claims: Painless JavaScript Testing. Is Facebook's JavaScript unit testing framework for testing services and React applications.
The so-called unit test is to test each unit. In popular terms, it generally targets functions, classes or individual components, and does not involve systems and integration. Unit testing is the basic test of software testing. Jest mainly has the following features:
Adaptability: Jest is modular, extensible and configurable.
Sandbox and fast: Jest virtualizes the JavaScript environment, can simulate the browser, and execute in parallel
Snapshot test: Jest can Quickly write tests on snapshots or other serialized values of the React tree to provide a quickly updated user experience.
Support asynchronous code testing: support promises and async/await
Automatically generate static analysis results: not only display test case execution results, but also Statement, branch, function, etc. coverage.
Why use unit testing tools
During the development process, we can still write our own code for unit testing without using testing tools, but Our codes have a mutual calling relationship. During the testing process, we want to make the unit relatively independent and run normally. We need to mock the dependent functions and environment of the function under test, and perform test data input, test execution and There are many similarities in the inspection of test results, and testing tools provide us with convenience in these aspects.
Preparation stage
Requires an rn project, what is demonstrated here is my personal project ReactNative-ReduxSaga-TODO
Installation jest
If you created the rn project using the react-native init command line, and your rn version is above 0.38, there is no need to install it. If you are not sure, check whether the
package.json file contains the following code:
// package.json "scripts": { "test": "jest" }, "jest": { "preset": "react-native" }
If not, install npm i jest --save-dev and add the above code to The corresponding location of the package.json file.
After completing the above steps, simply run npm run test to test whether jest is configured successfully. But we did not write a test case, and the terminal will print no tests found. The configuration is now complete.
Snapshot test
Write a component
import React from 'react'; import { Text, View, } from 'react-native'; import PropTypes from 'prop-types'; const PostArea = ({ title, text, color }) => ( <View style={{ backgroundColor: '#ddd', height: 100 }}> <Text style={{ fontSize: 30 }}>{title}</Text> <Text style={{ fontSize: 15, color }}>{text}</Text> </View> ); export default PostArea;
Find the __test__ folder in the project root directory. Now, let’s use React’s test Renderer and Jest's snapshot functionality to interact with the component and capture the rendered output and create a snapshot file.
// PostArea_test.js import 'react-native'; import React from 'react'; import PostArea from '../js/Twitter/PostArea'; import renderer from 'react-test-renderer'; test('renders correctly', () => { const tree = renderer.create(<PostArea title="title" text="text" color="red" />).toJSON(); expect(tree).toMatchSnapshot(); });
Then run npm run test or jest in the terminal. Will output:
PASS __tests__\PostArea_test.js (6.657s)
√ renders correctly (5553ms)
› 1 snapshot written.
Snapshot Summary
› 1 snapshot written in 1 test suite.
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 1 added, 1 total
Time: 8.198s
Ran all test suites.
At the same time, a file will be output in the test folder, which is the generated snapshot.
// Jest Snapshot v1, https://goo.gl/fbAQLP exports[`renders correctly 1`] = ` <View style={ Object { "backgroundColor": "#ddd", "height": 100, } } > <Text accessible={true} allowFontScaling={true} disabled={false} ellipsizeMode="tail" style={ Object { "fontSize": 30, } } > title </Text> <Text accessible={true} allowFontScaling={true} disabled={false} ellipsizeMode="tail" style={ Object { "color": "red", "fontSize": 15, } } > text </Text> </View> `;
Modify source files
The next time the test is run, the rendered output will be compared to the previously created snapshot. Snapshots should be submitted together with the code. When a snapshot test fails, it needs to be checked for intentional or unintentional changes. If the changes are as expected, call jest -u to overwrite the current snapshot.
Let’s change the original code: change the font size of the second line
<Text style={{ fontSize: 14, color }}>{text}</Text>
At this time, we run jest again. At this time, the terminal will throw an error and point out the error location
#Because this code was intentionally changed by us, when we run jest -u, the snapshot will be overwritten. If you execute jest again, no error will be reported~
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
How to use parent component to pass value to child component in vue-prop
In Node.js Use cheerio to make a simple web crawler (detailed tutorial)
How to implement parent components to pass multiple data to child components in vue
The above is the detailed content of Detailed explanation of using jest to test react native components. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
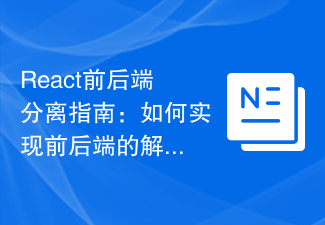
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
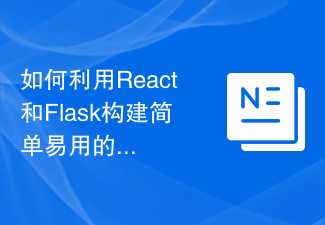
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
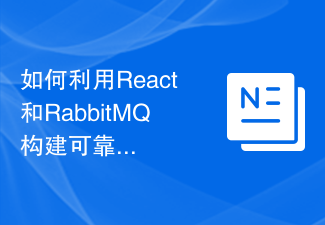
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
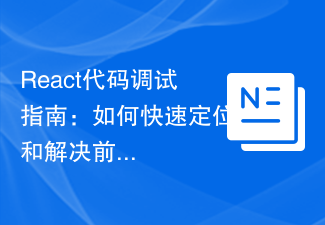
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
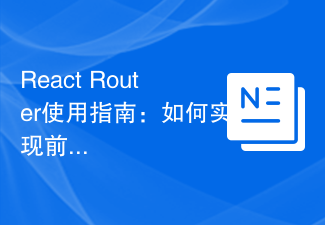
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
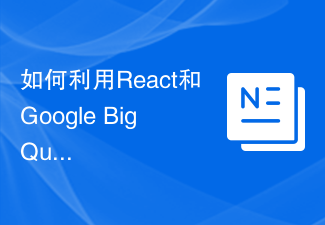
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
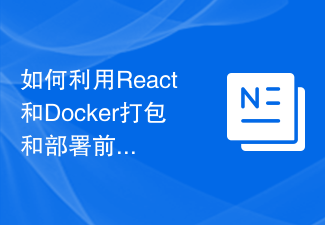
How to use React and Docker to package and deploy front-end applications. Packaging and deployment of front-end applications is a very important part of project development. With the rapid development of modern front-end frameworks, React has become the first choice for many front-end developers. As a containerization solution, Docker can greatly simplify the application deployment process. This article will introduce how to use React and Docker to package and deploy front-end applications, and provide specific code examples. 1. Preparation Before starting, we need to install
