How to implement SMS registration in Larav
This article mainly introduces the sample code for SMS registration using Laravel, using the Yunpian SMS platform. The content is quite good. I will share it with you now and give it as a reference.
I am working on a mall project in my company. Since there is only one backend, it is my turn to register via SMS. At the beginning, I was still a little weak in my heart. Fortunately, there is a book written by the summer
master of the Laravel-china
community. I referred to its writing method and ideas in it, and used the easy-sms
package. It only took half an afternoon to make it successfully. I will share it with everyone in the evening.
1. Determine the SMS operator
#I saw that the big guys all used Yunpian, so I did not hesitate to use it. It is recommended that companies use this text messaging platform, but others are also available.
First register an account yourself, and then find this
Click to start accessing and complete the novice guidance process.
The second signature and template must be filled in, similar to what I filled in below
It is worth noting that this template must be exactly the same as the text message content you set when using the easy-sms
package, otherwise an error will be reported.
Also remember to get APIKEY. At that time, configure it in env.
# 云片 YUNPIAN_API_KEY=9c60bdd**********
2. Installation easy-sms
package
Use this package to quickly implement the SMS sending function.
composer require "overtrue/easy-sms"
Since this component does not yet have Laravel's ServiceProvider
, for the convenience of use, we can encapsulate it ourselves.
First add the easysms.php
file in the config directory
Fill in the following content in config/easysms.php.
<?php return [ // HTTP 请求的超时时间(秒) 'timeout' => 5.0, // 默认发送配置 'default' => [ // 网关调用策略,默认:顺序调用 'strategy' => \Overtrue\EasySms\Strategies\OrderStrategy::class, // 默认可用的发送网关 'gateways' => [ 'yunpian', ], ], // 可用的网关配置 'gateways' => [ 'errorlog' => [ 'file' => '/tmp/easy-sms.log', ], 'yunpian' => [ 'api_key' => env('YUNPIAN_API_KEY'), ], ], ];
Then create a ServiceProvider
php artisan make:provider EasySmsServiceProvider
Modify the fileapp/providers/EasySmsServiceProvider.php
<?php namespace App\Providers; use Overtrue\EasySms\EasySms; use Illuminate\Support\ServiceProvider; class EasySmsServiceProvider extends ServiceProvider { /** * Bootstrap the application services. * * @return void */ public function boot() { // } /** * Register the application services. * * @return void */ public function register() { $this->app->singleton(EasySms::class, function ($app) { return new EasySms(config('easysms')); }); $this->app->alias(EasySms::class, 'easysms'); } }
Finally in config/app.php
Add the service you just created in providers
, App\Providers\EasySmsServiceProvider::class,
App\Providers\AppServiceProvider::class, App\Providers\AuthServiceProvider::class, // App\Providers\BroadcastServiceProvider::class, App\Providers\EventServiceProvider::class, App\Providers\RouteServiceProvider::class, App\Providers\EasySmsServiceProvider::class, //easy-sms
3, Create a route and corresponding controller
First create the route, we need an ajax method to request the SMS verification code, and a logical method to confirm the registration, as follows:
Route::group(['prefix' => 'verificationCodes', 'as' => 'verificationCodes.'], function() { Route::post('register', 'VerificationCodesController@register')->name('register'); Route::get('ajaxregister', 'VerificationCodesController@ajaxregister')->name('ajaxregister'); });
After the route is created, we use the command to generate the controller
php artisan make:controller Home\VerificationCodesController
and then directly write the register
and ajaxregister
methods in it
Code logic
Modify the file
app/Home/VerificationCodesController.php
<?php . . . use Overtrue\EasySms\EasySms; use App\Models\System\User; class VerificationCodesController extends Controller { // 这里验证就不写了。 public function ajaxregister(VerificationCodeRequest $request, EasySms $easySms) { //获取前端ajax传过来的手机号 $phone = $request->phone; // 生成4位随机数,左侧补0 $code = str_pad(random_int(1, 9999), 4, 0, STR_PAD_LEFT); try { $result = $easySms->send($mobile, [ 'content' => "【安拾商城】您的验证码是{$code}。如非本人操作,请忽略本短信" ]); } catch (Overtrue\EasySms\Exceptions\NoGatewayAvailableException $exception) { $response = $exception->getExceptions(); return response()->json($response); } //生成一个不重复的key 用来搭配缓存cache判断是否过期 $key = 'verificationCode_' . str_random(15); $expiredAt = now()->addMinutes(10); // 缓存验证码 10 分钟过期。 \Cache::put($key, ['mobile' => $mobile, 'code'=> $code], $expiredAt); return response()->json([ 'key' => $key, 'expired_at' => $expiredAt->toDateTimeString(), ], 201); }
In this way, the user can receive the text message, and The front end should save this key
and pass it to the backend when submitting the registration form to determine whether it has expired. The following is to determine whether it has expired and whether the verification code is wrong.
public function register(VerificationCodeRequest $request) { //获取刚刚缓存的验证码和key $verifyData = \Cache::get($request->verification_key); //如果数据不存在,说明验证码已经失效。 if(!$verifyData) { return response()->json(['status' =>0, 'message'=> '短信验证码已失效'], 422); } // 检验前端传过来的验证码是否和缓存中的一致 if (!hash_equals($verifyData['code'], $request->verification_code) { return redirect()->back()->with('warning', '短信验证码错误'); } $user = User::create([ 'mobile' => $verifyData['mobile'], 'password' => bcrypt($request->password), ]); // 清除验证码缓存 \Cache::forget($request->verification_key); return redirect()->route('login')->with('success', '注册成功!'); }
The above hash_equals
is a string comparison that can prevent timing attacks~
The above is my entire process.
The above is the entire content of this article. I hope it will be helpful to everyone's study. For more related content, please pay attention to the PHP Chinese website!
Related recommendations:
Use Laravel to generate Gravatar avatar address
How to create a custom artisan make command through laravel Create a new class file
The above is the detailed content of How to implement SMS registration in Larav. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
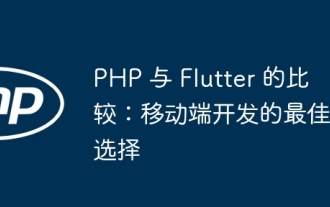
PHP and Flutter are popular technologies for mobile development. Flutter excels in cross-platform capabilities, performance and user interface, and is suitable for applications that require high performance, cross-platform and customized UI. PHP is suitable for server-side applications with lower performance and not cross-platform.
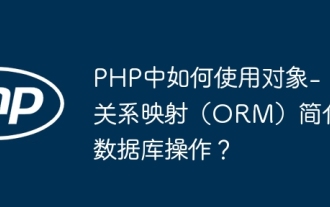
Database operations in PHP are simplified using ORM, which maps objects into relational databases. EloquentORM in Laravel allows you to interact with the database using object-oriented syntax. You can use ORM by defining model classes, using Eloquent methods, or building a blog system in practice.

PHP unit testing tool analysis: PHPUnit: suitable for large projects, provides comprehensive functionality and is easy to install, but may be verbose and slow. PHPUnitWrapper: suitable for small projects, easy to use, optimized for Lumen/Laravel, but has limited functionality, does not provide code coverage analysis, and has limited community support.
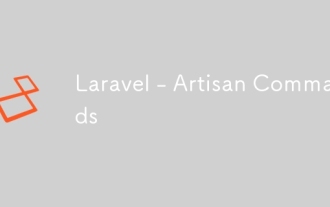
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
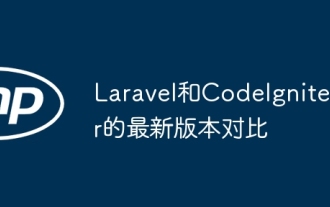
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
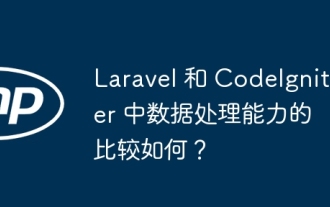
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
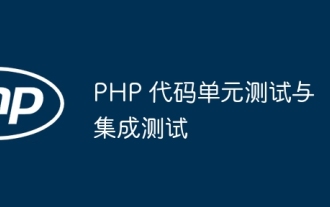
PHP Unit and Integration Testing Guide Unit Testing: Focus on a single unit of code or function and use PHPUnit to create test case classes for verification. Integration testing: Pay attention to how multiple code units work together, and use PHPUnit's setUp() and tearDown() methods to set up and clean up the test environment. Practical case: Use PHPUnit to perform unit and integration testing in Laravel applications, including creating databases, starting servers, and writing test code.
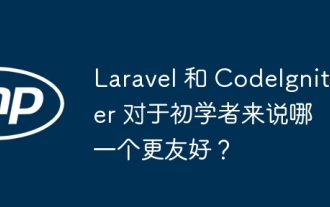
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
