Complete the writing of Message component using angular
This article mainly introduces how to write an angular version of the Message component. The editor thinks it is quite good, so I will share it with you now and give it as a reference. Let’s follow the editor and take a look.
The best way to learn a framework or library is to read the official documentation and start writing examples. I have been using my free time to learn Angular recently, so today I will try to write a message component and dynamically load the message component through the message service.
The projects I participate in are basically completed with jquery. Previously, I wrote a simple message plug-in myself in the project, as shown below.
Now use angular (version 5.0.0) to implement the message component.
message component
The message component should be displayed according to the incoming type, message and duration. Create three files: message.component.ts, message.component.html, message.component.css, the code is as follows.
//message.component.ts import {Component,Input,OnInit,ChangeDetectionStrategy} from '@angular/core'; import { trigger, state, style, transition, animate } from '@angular/animations'; const mapping={ success:'glyphicon-ok-sign', warning:'glyphicon-exclamation-sign', error:'glyphicon-exclamation-sign', info:'glyphicon-ok-circle' } @Component({ selector:'upc-ng-message', templateUrl:'./message.component.html', styleUrls:['./message.component.css'], changeDetection:ChangeDetectionStrategy.OnPush }) export class MessageComponent implements OnInit{ ngOnInit(): void { this.typeClass=['upc-message-' + this.msgType]; this.typeIconClass=[mapping[this.msgType]]; } @Input() msgType:'success' | 'info' | 'warning' | 'error'='info' @Input() payload:string = '' private typeClass private typeIconClass }
<!--*message.component.html--> <p class="upc-message"> <p class="upc-message-content" [ngClass]="typeClass"> <i class="glyphicon" [ngClass]="typeIconClass"></i> {{payload}} </p> </p>
.upc-message { position: fixed; z-index: 1999; width: 100%; top: 36px; left: 0; pointer-events: none; padding: 8px; text-align: center; } .upc-message i { margin-right: 8px; font-size: 14px; top: 1px; position: relative; } .upc-message-success i { color: green; } .upc-message-warning i { color: yellow; } .upc-message-error i { color: red; } .upc-message-content { padding: 8px 16px; -ms-border-radius: 4px; border-radius: 4px; -webkit-box-shadow: 0 2px 8px #000000; -ms-box-shadow: 0 2px 8px #000000; box-shadow: 0 2px 8px #000000; box-shadow: 0 2px 8px rgba(0,0,0,.2); background: #fff; display: inline-block; pointer-events: all; }
ComponentLoader
Through the dynamic components section of the official document, you can understand that dynamically creating components needs to be completed through ComponentFactoryResolver. Use ComponentFactoryResolver to create a ComponentFactory, and then create components through the create method of ComponentFactory. Looking at the API description in the official document, the create method of ComponentFactory requires at least one injector parameter, and the creation of the injector is also mentioned in the document, where the parameter providers is the class that needs to be injected. Let’s sort out the whole process:
Provide providers
Create Injector instance
Create ComponentFactory
Use ComponentFactory to create ComponentRef
//ComponentFactory的create方法 create(injector: Injector, projectableNodes?: any[][], rootSelectorOrNode?: string|any, ngModule?: NgModuleRef<any>): ComponentRef<C> //使用Injector的create创建injector实例 static create(providers: StaticProvider[], parent?: Injector): Injector
For code reuse, a general loader class is created here to complete the dynamic creation of components. Among them, the attch method is used to initialize the ComponentFactory (the parameter is the component type); the to method is used to identify the parent container of the component; the provider method is used to initialize the injectable class; the create method is used to create components and manual change detection; the remove method is used to Remove components.
import { ComponentFactoryResolver, ComponentFactory, ComponentRef, Type, Injector, Provider, ElementRef } from '@angular/core'; export class ComponentLoader<T>{ constructor(private _cfr: ComponentFactoryResolver, private _injector: Injector) { } private _componentFactory: ComponentFactory<T> attch(componentType: Type<T>): ComponentLoader<T> { this._componentFactory = this._cfr.resolveComponentFactory<T>(componentType); return this; } private _parent: Element to(parent: string | ElementRef): ComponentLoader<T> { if (parent instanceof ElementRef) { this._parent = parent.nativeElement; } else { this._parent = document.querySelector(parent); } return this; } private _providers: Provider[] = []; provider(provider: Provider) { this._providers.push(provider); } create(opts: {}): ComponentRef<T> { const injector = Injector.create(this._providers as any[], this._injector); const componentRef = this._componentFactory.create(injector); Object.assign(componentRef.instance, opts); if (this._parent) { this._parent.appendChild(componentRef.location.nativeElement); } componentRef.changeDetectorRef.markForCheck(); componentRef.changeDetectorRef.detectChanges(); return componentRef; } remove(ref:ComponentRef<T>){ if(this._parent){ this._parent.removeChild(ref.location.nativeElement) } ref=null; } }
At the same time, in order to facilitate the creation of loader, create the LoaderFactory class, the code is as follows:
import { ComponentFactoryResolver, Injector, Injectable, ElementRef } from '@angular/core'; import { ComponentLoader } from './component-loader.class'; @Injectable() export class ComponentLoaderFactory { constructor(private _injector: Injector, private _cfr: ComponentFactoryResolver) { } create<T>(): ComponentLoader<T> { return new ComponentLoader(this._cfr, this._injector); } }
message service
message service provides display of message API, the code is as follows:
import {Injectable,Injector} from '@angular/core'; import { ComponentLoaderFactory } from '../component-loader/component-loader.factory'; import {MessageComponent} from './message.component'; import {ComponentLoader} from '../component-loader/component-loader.class'; @Injectable() export class MessageService{ constructor(private _clf:ComponentLoaderFactory,private _injector:Injector){ this.loader=this._clf.create<MessageComponent>(); } private loader:ComponentLoader<MessageComponent> private createMessage(t,c,duration=2000){ this.loader.attch(MessageComponent).to('body'); const opts = { msgType: t, payload:c }; const ref = this.loader.create(opts); ref.changeDetectorRef.markForCheck(); ref.changeDetectorRef.detectChanges(); let self=this; let st = setTimeout(() => { self.loader.remove(ref); }, duration); } public info(payload,duration?) { this.createMessage('info',payload,duration); } public success(payload,duration?) { this.createMessage('success',payload,duration); } public error(payload,duration?) { this.createMessage('error',payload,duration); } public warning(payload,duration?) { this.createMessage('warning',payload,duration); } }
message.module
Finally, add message.module.ts. Remember to add dynamically created components to the entryComponents array.
import {NgModule} from '@angular/core'; import { CommonModule } from '@angular/common'; import {MessageComponent} from './message.component'; import {MessageService} from './message.service'; import {ComponentLoaderFactory} from '../component-loader/component-loader.factory'; @NgModule({ imports:[CommonModule], declarations:[MessageComponent], providers:[MessageService,ComponentLoaderFactory], entryComponents:[MessageComponent], exports:[MessageComponent] }) export class MessageModule{ }
Use method
Inject MessageService and call the API to use the Message component.
this._msgService.success('成功了!');
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
How to achieve the display box effect in the Vue component Toast
About rules parameter processing in webpack
How to implement simple calculations in AngularJS
The above is the detailed content of Complete the writing of Message component using angular. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


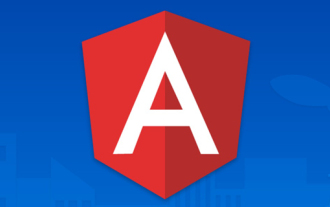
This article continues the learning of Angular, takes you to understand the metadata and decorators in Angular, and briefly understands their usage. I hope it will be helpful to everyone!
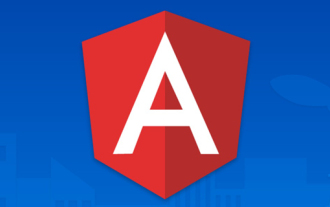
This article will give you an in-depth understanding of Angular's state manager NgRx and introduce how to use NgRx. I hope it will be helpful to you!

Angular.js is a freely accessible JavaScript platform for creating dynamic applications. It allows you to express various aspects of your application quickly and clearly by extending the syntax of HTML as a template language. Angular.js provides a range of tools to help you write, update and test your code. Additionally, it provides many features such as routing and form management. This guide will discuss how to install Angular on Ubuntu24. First, you need to install Node.js. Node.js is a JavaScript running environment based on the ChromeV8 engine that allows you to run JavaScript code on the server side. To be in Ub
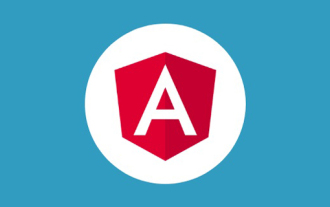
Do you know Angular Universal? It can help the website provide better SEO support!

With the rapid development of the Internet, front-end development technology is also constantly improving and iterating. PHP and Angular are two technologies widely used in front-end development. PHP is a server-side scripting language that can handle tasks such as processing forms, generating dynamic pages, and managing access permissions. Angular is a JavaScript framework that can be used to develop single-page applications and build componentized web applications. This article will introduce how to use PHP and Angular for front-end development, and how to combine them
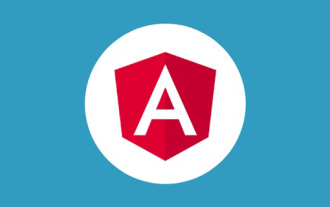
This article will share with you an Angular practical experience and learn how to quickly develop a backend system using angualr combined with ng-zorro. I hope it will be helpful to everyone!
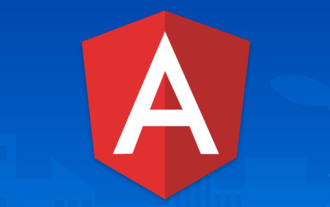
How to use monaco-editor in angular? The following article records the use of monaco-editor in angular that was used in a recent business. I hope it will be helpful to everyone!
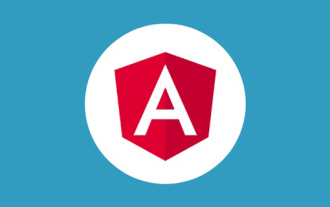
This article will take you through the independent components in Angular, how to create an independent component in Angular, and how to import existing modules into the independent component. I hope it will be helpful to you!
