How to create Web and TCP servers in Node.js
This article mainly introduces the methods and processing skills of using Node.js to create Web servers and TCP servers. Readers who need it can learn it.
Create a Web server using the http module
Function of the Web server:
Accept HTTP requests (GET, POST, DELETE, PUT, PATCH)
Process HTTP requests (handle it yourself, or request other programs to handle it)
Respond (return pages, files, various types of data, etc.)
Common Web server architecture:
Nginx, Apache: responsible for accepting HTTP requests, determining who will handle the request, and returning the results of the request
php-fpm/php module: processing assigned to itself request, and return the processing results to the assignor
Common request types:
Request files: including static files (web pages, pictures, front-end JavaScript files, css files ...), and the files processed by the program
Complete specific operations: such as logging in, obtaining specific data, etc.
Node.js Web server:
Does not rely on other specific web server software (such as Apache, Nginx, IIS...)
Node.js code handles request logic
Node.js The code is responsible for various "configurations" of the Web server
Use Express to create a Web server
Simple Express server
Static file service
Routing
Middleware
Simple Express server:
1 2 3 4 5 6 7 8 |
|
Static file scope:
Web pages, plain text, images, front-end JavaScript code, CSS style sheet files, media files, font files
Use Express to access static files
1 |
|
Routing:
Assign different requests to the corresponding processing functions
Distinguishing: path, request method
Three routing implementation methods:
path: relatively simple
Router: more suitable for multiple sub-routes under the same route
route: More suitable for API
Middleware
Connect: Node.js middleware framework
Layered processing
Each layer Implement a function
Create TCP server
Use net module to create TCP server
Use telnet to connect to TCP server
Use net to create TCP client
Use node.js to build a simple web server JS code part:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
|
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
Error in loading path in laydate.js
How to implement route parameter passing in vue-router
How to use jQuery to operate table to merge cells
The above is the detailed content of How to create Web and TCP servers in Node.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


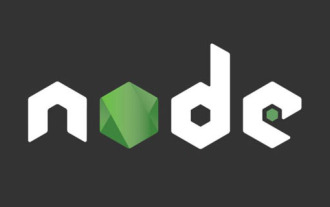
The Node service built based on non-blocking and event-driven has the advantage of low memory consumption and is very suitable for handling massive network requests. Under the premise of massive requests, issues related to "memory control" need to be considered. 1. V8’s garbage collection mechanism and memory limitations Js is controlled by the garbage collection machine
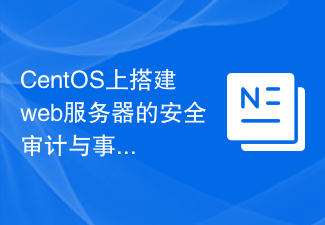
Overview of security auditing and event log management of web servers built on CentOS. With the development of the Internet, security auditing and event log management of web servers have become more and more important. After setting up a web server on the CentOS operating system, we need to pay attention to the security of the server and protect the server from malicious attacks. This article will introduce how to perform security auditing and event log management, and provide relevant code examples. Security audit Security audit refers to comprehensive monitoring and inspection of the security status of the server to promptly discover potential
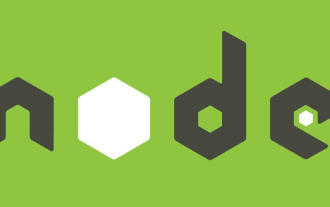
The file module is an encapsulation of underlying file operations, such as file reading/writing/opening/closing/delete adding, etc. The biggest feature of the file module is that all methods provide two versions of **synchronous** and **asynchronous**, with Methods with the sync suffix are all synchronization methods, and those without are all heterogeneous methods.
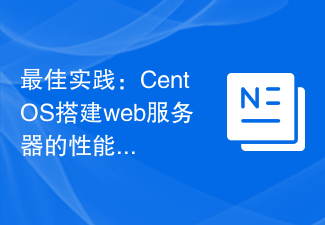
Best Practices: Performance Tuning Guide for Building Web Servers on CentOS Summary: This article aims to provide some performance tuning best practices for users building web servers on CentOS, aiming to improve the performance and response speed of the server. Some key tuning parameters and commonly used optimization methods will be introduced, and some sample codes will be provided to help readers better understand and apply these methods. 1. Turn off unnecessary services. When building a web server on CentOS, some unnecessary services will be started by default, which will occupy system resources.
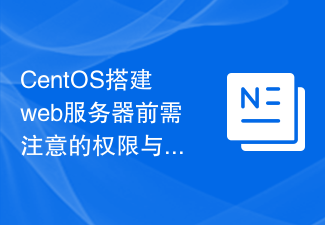
Permissions and access control strategies that you need to pay attention to before building a web server on CentOS. In the process of building a web server, permissions and access control strategies are very important. Correctly setting permissions and access control policies can protect the security of the server and prevent unauthorized users from accessing sensitive data or improperly operating the server. This article will introduce the permissions and access control strategies that need to be paid attention to when building a web server under the CentOS system, and provide corresponding code examples. User and group management First, we need to create a dedicated
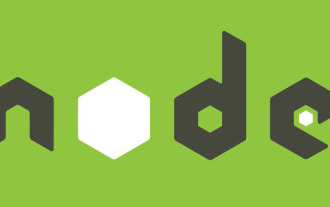
The event loop is a fundamental part of Node.js and enables asynchronous programming by ensuring that the main thread is not blocked. Understanding the event loop is crucial to building efficient applications. The following article will give you an in-depth understanding of the event loop in Node. I hope it will be helpful to you!
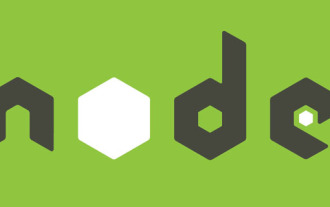
At the beginning, JS only ran on the browser side. It was easy to process Unicode-encoded strings, but it was difficult to process binary and non-Unicode-encoded strings. And binary is the lowest level data format of the computer, video/audio/program/network package
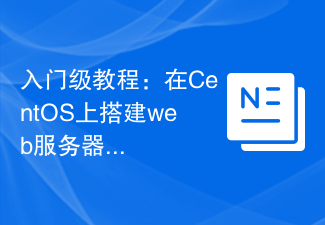
Entry-level tutorial: A quick guide to building a web server on CentOS Introduction: In today's Internet era, building your own web server has become a need for many people. This article will introduce you to how to build a web server on the CentOS operating system, and provide code examples to help readers quickly implement it. Step 1: Install and configure Apache Open the terminal and install the Apache server through the following command: sudoyuminstallhttpd After the installation is complete, start Apac
