Commonly used system functions in php projects
String function
strlen: Get the string length, byte length
substr: String interception, get the string (intercept according to bytes)
strchr: Similar to substr, intercept from the specified position until the end
strrchr (get the file suffix name): Same as strchr, just search for characters starting from the right
strtolower : All characters are lowercase (for English letters)
strtoupper: All characters are uppercase
strrev: String reverse (only English can be reversed: English storage has only one byte) , reverse by bytes
strpos: Find the position (numeric subscript) where the corresponding character appears in the string, starting from the leftmost
strrpos: Same as strpos, except from Start looking for the right side of the string
trim: remove the characters on both sides of the function, the default is a space
Time and date function
time: get the current time Timestamp (integer: starting from 0:00:00 on January 1, 1970, Greenwich Mean Time) Number of seconds
date: time serialization function, converts the specified timestamp into the specified time and date Display format (arbitrary string: professional formatting regulations), if no timestamp is specified, the system defaults to the timestamp of the current time
strtotime: Convert a string in time and date format into the corresponding time Stamp (as long as it is a correct English time expression, it can be converted)
microtime: microsecond timestamp, returns different results according to different requirements. Mixed microtime (Boolean type), can return a floating point number. Time, can also return an array (timestamp and microseconds)
Mathematical related functions
abs: absolute value
floor: down The result of rounding floor(3.2) is equal to 3
ceil: Round up
round: Rounding
rand: Get a random integer within the specified range
mt_rand: Get a random integer within the specified range (more efficient)
Array related functions
key: Get the next element pointed to by the current pointer of the current array Standard
current: Get the value of the element pointed to by the current pointer
next: Get the value of the next element and move the pointer down
prev: Get the value of the previous element value, and move the pointer up
end: Move the pointer to the last element of the array, and return the value of the final pointer position
reset: Move the pointer to the first element of the array , returns the value of the final pointer position
array_keys: Gets all the key names of an array, returns an index array
array_values: Gets all the values of an array, returns an index array
explode: Explode, divide a string into multiple segments according to a specified rule (usually special characters), each segment is treated as an element of the array, and an index array is returned
implode : Gluing, splicing all the elements in an array into a string according to a specified rule (special characters)
array_merge: Merging, refers to combining the elements in two arrays Elements are accumulated. If the subsequent array has the same subscript (key name: association) as the previous array, then the value of the subsequent element will overwrite the previous one; if it is the same subscript of the index, the subscript will be automatically modified and superimposed on the previous array. inside.
Data structure simulation function
array_shift: Pop elements from the front of the array and get the value of the element
array_pop: Pop elements from the back of the array, Get the value of the element
array_unshift: Push elements from the front of the array to get the number of current array elements
array_push: Push elements from the back of the array to get the number of current array elements
Determine the variable
is_bool: Determine whether it is a Boolean type
is_float: Determine the floating point type
is_integer: Determine the integer type
is_object: Judgment object
is_array: Judgment array
is_string: Judgment string
is_resource: Judgment resource
is_scalar: scalar It is scalar, and the basic data types are judged: integer, floating point, Boolean and string.
is_null: Whether it is empty.
is_numeric: Judgment of characters composed of numbers or pure numbers. String
gettype: Get the data type
settype: Change the data type
File operation function
opendir (path): open A path resource (read all the data inside the path into memory)
readdir (path resource): Read the name of the file pointed to by the current resource pointer from the folder resource, and the pointer will move downward by one Bit
closedir (resource): Release the corresponding file resource
scandir (path): Read all the file names inside a path and return an array. Each element of the array is a file name.
file_exists: Determine whether a file exists (file is a broad sense: path and file)
is_dir: Determine whether a specified path exists (folder)
is_file: Determine a Specify whether the path is a file (file)
mkdir: Create a path, if the path exists, an error will be reported
rmdir: Remove the folder
file_get_contents: From a specified Read the data content in the file.
file_put_contents: Write the specified string to the corresponding file
fopen: Open a file resource
fgetc: c represents character, read one character at a time
fgets: s represents string, which means multiple characters can be read, depending on the specified Read the length or encounter a newline (only one line of data can be read at most)
Both functions operate on the current resource pointer, and will move the pointer down after reading
fread : Get the data of the specified length until the end of the file
fwrite: Write data to the location of the file resource pointer. Writing will not move the existing things back at the current location, but will overwrite
fseek: Specify the pointer to the corresponding location
fclose: Use the corresponding file resource
copy: Copy
unlink: Delete the file
rename: Rename the file
filemtime: m represents modify, the time when the file was last modified
filesize: file size (bytes)
fileperms: file permissions (under Linux octal)

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


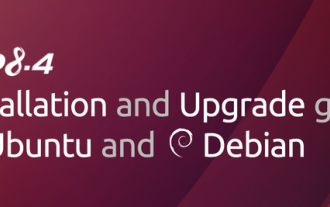
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
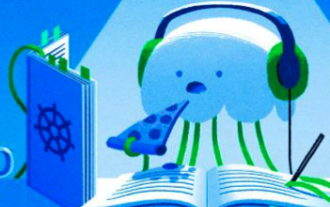
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
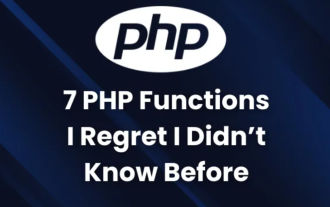
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
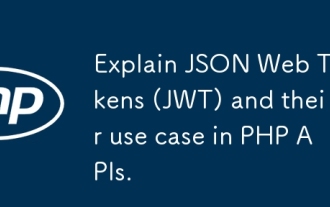
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
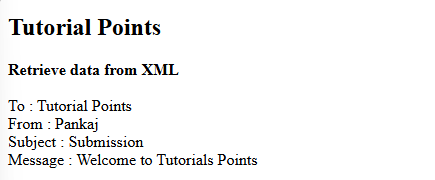
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
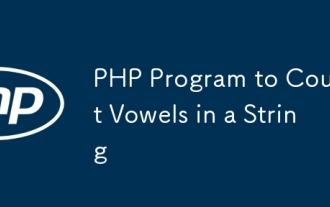
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
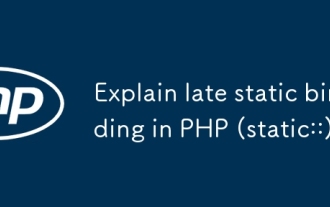
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.