PHP commonly used array functions
* Functions should mainly understand the following points?
* 1. Function: What can it do?
* 2. Scenario: Where is it used?
* 3. Parameters: required and optional
* 4. Return value: type and quantity
* 1. Key and value operations (6)
* 1. in_array(value, array): Whether val is in array, return Boolean value
* 2. array_key_exists(key, array): Whether key is in array, return Boolean value
* 3. array_values(array): Returns the value part of array in index mode
* 4. array_keys(array[,value]): Returns the key part of array in index mode, and can also return the key of the specified value
* 5. array_search(value, array): Return the key of the specified value in string format
* 6. array_filp(array): Key value swap
* 2. Inside the array Pointer (cruise) operations (8)
* 1. key(array): returns the key of the current element
* 2. current(array): the value of the current element, pos() It is a function with the same name
* 3. next(array): The pointer moves down, pointing to the next element, and returns the current value
* 4. reset(array): The pointer is reset, pointing to the first element element, and return its value
* 5. end(array): The pointer moves to the last element
* 6. prev(array): The pointer moves forward one bit, and returns The value of the current element
* 7. each(array): Returns the index and associated description of the key value of the current element, and automatically moves the pointer down
* 8. list($a ,$b,...): Assign the values in the index array to a set of variables
echo '<pre class="brush:php;toolbar:false">'; $user = ['id'=>5,'name'=>'peter','gender'=>'male','age'=>30]; print_r($user); //查看数组 echo '<hr color="red">';
//1. Commonly used operation functions for keys and values in arrays
// 1. in_array(value, array): Whether val is in the array, return a Boolean value
echo in_array('Peter Zhu',$user) ? '存在<br>' : '不存在<br>';
//2. array_key_exists(key, array): Whether the key is in the array, return a Boolean value
echo array_key_exists('name',$user) ? '存在<br>' : '不存在<br>';
//3.array_values(array): Return the value part of array in index mode
print_r(array_values($user));
//4.array_keys(array[,value]): Return the key part of array in index mode
print_r(array_keys($user));
//4-1.array_keys(array[,value]): Returns the key part of the array in index mode, and can also return the key of the specified value
print_r(array_keys($user,'male'));
//5. array_search(value, array): Return the key of the specified value in string mode
print_r(array_search('peter', $user));
//6. array_filp(array): key value swap
print_r(array_flip($user));
// 2. Array internal pointer (cruise) operation
//count(array)The number of elements in the current array
echo count($user),'<br>';
//The current pointer points to the first element
//1.key(array): Returns the key of the current element
echo key($user),'<br>';
//2.current(array): The value of the current element, pos() is the function of the same name
echo current($user), '<br>';
//3.next(array): The pointer moves down, pointing to the next Element
next($user);
//View the key value of the current element
echo key($user),'<br>'; echo current($user), '<br>';
//Next(array) can also return in addition to moving the pointer down The value of the current element
var_dump(next($user)); echo key($user),'<br>'; echo current($user), '<br>';
//Continue to traverse downwards and find that next(array) returns false, indicating that the traversal is over
var_dump(next($user));
//There is no data at the end, there will be no more output
echo key($user),'<br>'; echo current($user), '<br>';
//4.reset(array): The pointer is reset, points to the first element, and returns its value
reset ($user);
//In addition to reset, the value of the first element can also be returned
// var_dump(reset($user));
echo key($user),'<br>'; echo current($user), '
';
//5. end(array): The pointer moves to the last element
end($user);
// var_dump(end($user)); //Can also return the current The value of the element
echo key($user),'<br>'; echo current($user), '
';
//6. prev(array): The pointer moves forward one bit and returns the value of the current element
prev($user); echo key($user),'<br>'; echo current($user), '
';
//7. each(array): Returns the current The index of the element's key value and the associated description of the array, and the pointer is automatically moved down
* Returns an array of four elements:
* 2 index elements, [0] is the key, [ 1] is the value
* 2 associated elements, [key] is the key, [value] is the value
@print_r(each($user));
/ /Note: Due to execution efficiency issues, this function has been abandoned in php7
//8. list($a,$b,...): Assign the value in the index array to a group Variable
reset($user); //复位指针
* Operation performed:
* 1.each($user): Get the index part of the current element
* 2.list($key, $value ): The value of [0] is assigned to the variable $key, the value of [1] is assigned to the variable $value
* 3. The array $user pointer automatically moves down
list($key, $value) = each($user); echo $key,'=>'.$value,'<br>';
//Repeated call
list($key, $value) = each($user); echo $key,'=>'.$value,'<br>';
//Very suitable for loop implementation
echo '<hr color="blue">'; reset($user); while(list($key, $value) = each($user)) { echo $key,'=>'.$value,'<br>'; }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


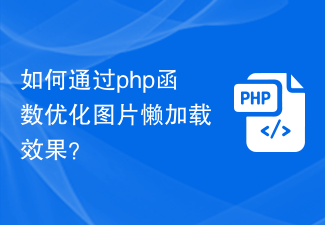
How to optimize the lazy loading effect of images through PHP functions? With the development of the Internet, the number of images in web pages is increasing, which puts pressure on page loading speed. In order to improve user experience and reduce loading time, we can use image lazy loading technology. Lazy loading of images can delay the loading of images. Images are only loaded when the user scrolls to the visible area, which can reduce the loading time of the page and improve the user experience. When writing PHP web pages, we can optimize the lazy loading effect of images by writing some functions. Details below
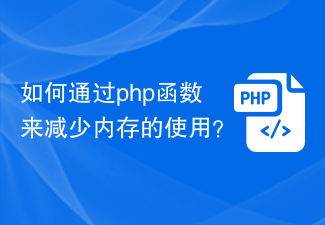
How to reduce memory usage through PHP functions. In development, memory usage is a very important consideration. If a large amount of memory is used in a program, it may cause slowdowns or even program crashes. Therefore, reasonably managing and reducing memory usage is an issue that every PHP developer should pay attention to. This article will introduce some methods to reduce memory usage through PHP functions, and provide specific code examples for readers' reference. Use the unset() function to release variables in PHP. When a variable is no longer needed, use
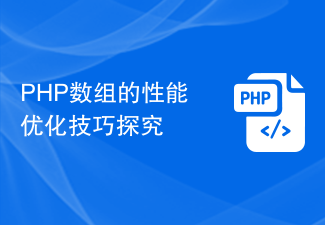
PHP array is a very common data structure that is often used during the development process. However, as the amount of data increases, array performance can become an issue. This article will explore some performance optimization techniques for PHP arrays and provide specific code examples. 1. Use appropriate data structures In PHP, in addition to ordinary arrays, there are some other data structures, such as SplFixedArray, SplDoublyLinkedList, etc., which may perform better than ordinary arrays in certain situations.
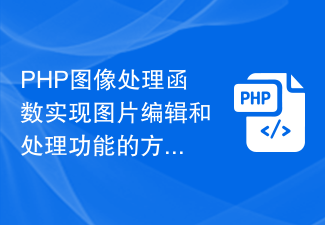
PHP image processing functions are a set of functions specifically used to process and edit images. They provide developers with rich image processing functions. Through these functions, developers can implement operations such as cropping, scaling, rotating, and adding watermarks to images to meet different image processing needs. First, I will introduce how to use PHP image processing functions to achieve image cropping function. PHP provides the imagecrop() function, which can be used to crop images. By passing the coordinates and size of the cropping area, we can crop the image

PHP function introduction: strtr() function In PHP programming, the strtr() function is a very useful string replacement function. It is used to replace specified characters or strings in a string with other characters or strings. This article will introduce the usage of strtr() function and give some specific code examples. The basic syntax of the strtr() function is as follows: strtr(string$str, array$replace) where $str is the original word to be replaced.
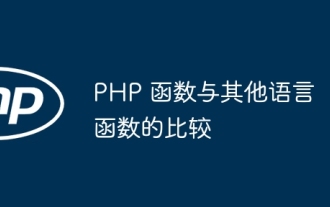
PHP functions have similarities with functions in other languages, but also have some unique features. Syntactically, PHP functions are declared with function, JavaScript is declared with function, and Python is declared with def. In terms of parameters and return values, PHP functions accept parameters and return a value. JavaScript and Python also have similar functions, but the syntax is different. In terms of scope, functions in PHP, JavaScript and Python all have global or local scope. Global functions can be accessed from anywhere, and local functions can only be accessed within their declaration scope.
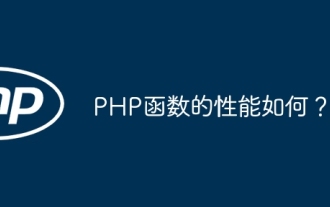
The performance of different PHP functions is crucial to application efficiency. Functions with better performance include echo and print, while functions such as str_replace, array_merge, and file_get_contents have slower performance. For example, the str_replace function is used to replace strings and has moderate performance, while the sprintf function is used to format strings. Performance analysis shows that it only takes 0.05 milliseconds to execute one example, proving that the function performs well. Therefore, using functions wisely can lead to faster and more efficient applications.

The main differences between PHP and Flutter functions are declaration, syntax and return type. PHP functions use implicit return type conversion, while Flutter functions explicitly specify return types; PHP functions can specify optional parameters through ?, while Flutter functions use required and [] to specify required and optional parameters; PHP functions use = to pass naming Parameters, while Flutter functions use {} to specify named parameters.