Copying and cloning objects in php
* Copying and cloning of objects
* 1. By default, objects are passed by reference (actually a copy of the object identifier, which will be discussed in detail later)
* 2. In other words, the two object variables actually refer to the same object
* 3. If you want to create a new object, you must use the clone keyword to clone the current object
* 4 .When using the clone keyword, if there is __clone() in the class, it will be automatically called
* 5. The __clone() method runs on the newly created object
* 6.__clone( ) method can control what we copy and the basic operations to be completed when cloning
class Member { //声明三个私有属性 private $name; //会员名 private $email; //会员邮箱 private $score; //会员积分 //构造方法 public function __construct($name='',$email='',$score=0) { $this->name = $name; $this->email = $email; $this->score = $score; } //查询器(暂时省略访问控制) public function __get($name) { return $this->$name; } //设置器(暂时省略访问控制) public function __set($name,$value) { $this->$name = $value; } //克隆魔术方法在对象克隆时自动调用,针对新对象进行初始化操作 public function __clone() { $this->score = 0; } }
//Instantiate the member class Member and create the member object $member
$member = new Member('peter','peter@php.cn',1000);
//Access test
echo $member->score;
//Copy the member object
$member1 = $member;
//Use the new variable name $member1 to update the object information
$member1->score = 2000;
//Use the new object variable name $member1 to access
echo $member1->score; echo '<hr>';
//Use the original object name $member to access
echo $member->score;
* Conclusion:
* 1. The result of the new variable modification is reflected in the original object variable
* 2 .Explain that these two variables actually point to the same object
* 3. That is, the object is passed by reference by default
* 4. In other words, the second variable name $member1 It is just an alias of the original variable, and no new object is created
* 5. It can be understood that it is just a new name for the identifier of the original object
var_dump($member1); //对象id=1 var_dump($member); //对象id=1
//Explanation$ member1 and $member are two identical objects, just with different names
//What should you do if you want to completely create a brand new object? You need to use the keyword: clone
$member2 = clone $member;
//Let’s first check the score attribute value of the object variable $member2
echo $member2->score; //目前是原始值2000
//Modify the score value
$member2->score = 5000; echo '<hr>';
//Check the score in $member2 again
echo $member2->score; //新值5000 echo '<hr>';
//Think about it, will my modification affect the score attribute value of the original $member variable?
echo $member->score; //发现原对象的score属性值仍为2000,未发生变化
//Why is this? Because we used cloning technology to create Two completely different object variables
//Verification below
var_dump($member); //变量id是1 var_dump($member2); //变量id是2
//The IDs of these two object variables are different, indicating that they are two completely different objects
//The following is a task to complete: When creating a new member object, clear the member's points to zero? What should be done?
//Create a __clone() clone in the Member class Magic method, preprocess the cloned new object
//Demonstrate it again
//View the current points
echo $member->score;
//Clone to create a brand new object $member3
$member3 = clone $member;
//Check the score value of the new object $member3 to verify whether __clone() in the class is effective?
echo '<hr>'; echo $member3->score; //0,说明克隆魔术方法已经生效
The above is the detailed content of Copying and cloning objects in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
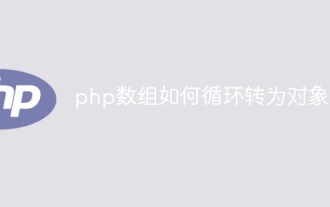
There are two ways to convert a PHP array into an object in a loop: 1. Use forced type conversion to convert the array into an object, and the keys of the array must be valid object attribute names; 2. Create a new object and add the elements of the array Copied into the object, independent of whether the array keys are valid as property names of the object.
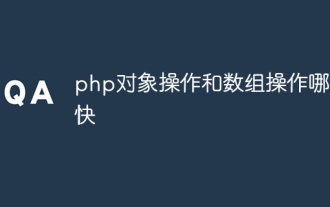
PHP array operations are faster than PHP object operations. The reasons are: 1. Object operations involve steps such as creating objects, calling methods and accessing properties, which may be slower in performance; 2. Array operations are a special type of variables. Can hold multiple values, use different methods and functions on arrays, and can perform fast and efficient operations on arrays.
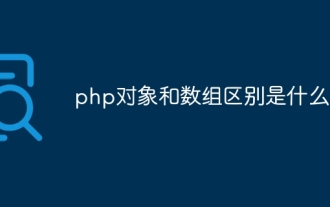
The difference between PHP objects and arrays is: 1. The object is a composite data type, while the array is a simple data type; 2. The properties and methods of the object can be accessed through the instance of the object, while the elements of the array can be accessed through the index; 3. An object is an entity that encapsulates properties and methods, while an array is an ordered collection of elements; 4. Objects are passed by reference in PHP, while arrays are passed by value in PHP; 5. Objects are suitable for describing entities with state and behavior, while arrays are suitable for storing and processing large amounts of similar data.
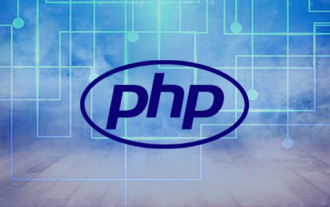
PHP arrays are not objects. In PHP, arrays and objects are two different data types. An array is a collection of ordered data; and an object is the result of instantiation of a class, which contains not only properties but also methods. Objects can encapsulate operations on data, but arrays cannot.
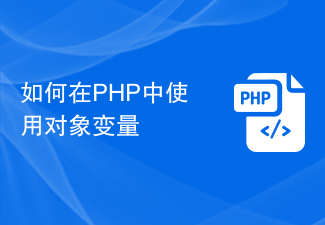
How to use object variables in PHP requires specific code examples. In PHP, using object variables makes it easier to manage and manipulate objects. An object variable is a data type that stores object instances. The object can be manipulated by calling methods of the class and accessing the properties of the class. The following will introduce in detail how to use object variables in PHP and provide corresponding code examples. Creating objects In PHP, you can use the new keyword to create objects. An example is as follows: classCar{public$colo
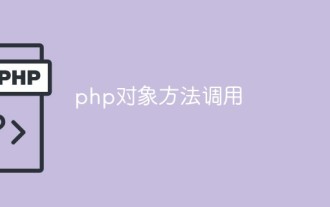
PHP is a very popular programming language that can be used to develop various applications, especially web applications. In PHP, object-oriented programming is one of its important features. This article will explore how to call object methods in PHP.
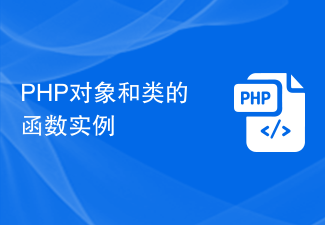
PHP is an object-oriented programming language that supports the concepts of objects and classes. In PHP, an object is an instance of a class, which can store data and functions, which are called methods. By using functions of PHP objects and classes, we can easily organize our code and improve code reusability. In this article, we will introduce examples of functions on PHP objects and classes and their functionality. Constructor (__construct) A constructor is a function that is automatically called when an object is created. It is used to initialize the properties of the object and perform

In PHP, it is very simple to obtain all the methods in an object, which can be achieved by using the ReflectionClass class in the PHP standard library. The ReflectionClass class provides a method to reflect all information of a class in PHP, including class name, attributes, methods, etc. Below we introduce in detail how to use the ReflectionClass class to obtain all methods in an object.
