Common magic method functions and usage in PHP
This article mainly introduces the functions and usage of common magic methods in PHP. It has certain reference value. Now I share it with everyone. Friends in need can refer to it
Overview
In object-oriented programming, PHP provides a series of magic methods, which provide a lot of convenience for programming. Magic methods in PHP usually start with __ (two underscores) and do not require explicit calls but are triggered by certain conditions.
Before starting
Before summarizing PHP’s magic methods, let’s define two classes for use in later examples:
<?php class Device{ public $name,$battery,$data = [],$connection; protected function connect(){ $this->connection = 'resource'; echo $this->name.'connected'.PHP_EOL; } protected function disconnect(){ $this->connection = null; echo $this->name.'disconnected'.PHP_EOL; } }
The Device class has four member attributes and two member methods.
<?php class Battery{ private $charge = 0; public function setCharge($charge){ $charge = (int)$charge; if($charge < 0){ $charge = 0; }else if($charge > 100){ $charge = 100; } $this->charge = $charge; } }
The Battery class has one member property and one member method.
Constructor and destructor
Constructor and destructor are called when the object is created and destroyed respectively. When an object is "destroyed", it means that there is no reference to the object. For example, if the variable that refers to the object is deleted (unset), reassigned, or the script execution ends, the destructor will be called.
__construct()
__construct()The constructor is by far the most commonly used function. When creating an object, you can do some initialization work in the constructor. You can define any number of parameters for the constructor, as long as the corresponding number of parameters is passed in when instantiating. Any exception that occurs in the constructor prevents the object from being created.
<?php class Device{ public $name,$battery,$data = [],$connection; public function __construct(Battery $battery,$name){ $this->battery = $battery; $this->name = $name; $this->connect(); } protected function connect(){ $this->connection = 'resource'; echo $this->name.'connected'.PHP_EOL; } protected function disconnect(){ $this->connection = null; echo $this->name.'disconnected'.PHP_EOL; } }
In the above sample code, the constructor of the Device class assigns values to the member properties and calls the connect() method.
Declaring the constructor as a private method prevents objects from being created outside the class, which is often used in the simplex pattern.
__desctruct()
The destructor is usually called when the object is destroyed. The destructor does not receive any parameters. Some cleanup work is often performed in the destructor, such as closing the database connection, etc.
__get()
The magic method __get() is called when we try to access a property that does not exist. It receives a parameter that represents the name of the accessed attribute and returns the value of the attribute. In the Device class above, there is a data attribute, which plays a role here, as shown in the following code:
<?php class Device{ public $name,$battery,$data = [],$connection; public function __construct(Battery $battery,$name){ $this->battery = $battery; $this->name = $name; $this->connect(); } protected function connect(){ $this->connection = 'resource'; echo $this->name.'connected'.PHP_EOL; } protected function disconnect(){ $this->connection = null; echo $this->name.'disconnected'.PHP_EOL; } } $battery = new Battery(); $device = new Device($battery,'mac'); echo $device->aaa; //Notice: Undefined property: Device::$aaa
<?phpheader("Content-type: text/html; charset=utf-8"); class Device{ public $name,$battery,$data = [],$connection; public function __construct(Battery $battery,$name){ $this->battery = $battery; $this->name = $name; $this->connect(); } public function __get($name){ if(array_key_exists($name,$this->data)){ return $this->data[$name]; } return '属性不存在'; } protected function connect(){ $this->connection = 'resource'; echo $this->name.'connected'.PHP_EOL; } protected function disconnect(){ $this->connection = null; echo $this->name.'disconnected'.PHP_EOL; } }$battery = new Battery(); $device = new Device($battery,'mac'); echo $device->aaa; //macconnected 属性不存在
The most commonly used place for this magic method is to create a "read-only" Properties to extend access control. In the above Battery class, there is a private property $charge, which we can extend through the __get() magic method to be readable but not modifyable outside the class. The code is as follows:
<?php class Battery { private $charge = 0; public function __get($name) { if(isset($this->$name)) { return $this->$name; } return null; } }
__set()
__set() magic method will be called when we try to modify an inaccessible property. It receives two parameters, One represents the name of the attribute, and one represents the value of the attribute. The sample code is as follows:
<?php header("Content-type: text/html; charset=utf-8"); class Device{ public $name,$battery,$data = [],$connection; public function __construct(Battery $battery,$name){ $this->battery = $battery; $this->name = $name; $this->connect(); } public function __get($name){ if(array_key_exists($name,$this->data)){ return $this->data[$name]; } return '属性不存在'; } public function __set($name,$value){ $this->data[$name] = $value; } protected function connect(){ $this->connection = 'resource'; echo $this->name.'connected'.PHP_EOL; } protected function disconnect(){ $this->connection = null; echo $this->name.'disconnected'.PHP_EOL; } }$battery = new Battery(); $device = new Device($battery,'mac'); $device->aaa = '哈哈'; echo $device->aaa; //macconnected 哈哈
__isset()
__isset() magic method will be called when the isset() method is called on an inaccessible property. It receives A parameter indicating the name of the attribute. It should return a Boolean value indicating whether the property exists. The code is as follows:
<?php class Device{ private function __isset($name){ return array_key_exists($name,$this->data); }
If the members in the object are public, you can use the isset() function directly. If it is a private member attribute, you need to add an __isset() method to the class
__unset()
__unset() magic method before calling unset The () function is called when destroying an inaccessible property. It receives a parameter expressing the name of the property.
The above is the entire content of this article. I hope it will be helpful to everyone's study. For more related content, please pay attention to the PHP Chinese website!
Related recommendations:
The above is the detailed content of Common magic method functions and usage in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


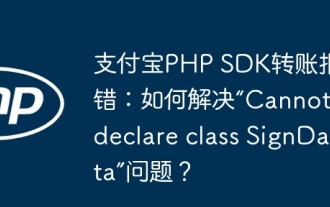
Alipay PHP...
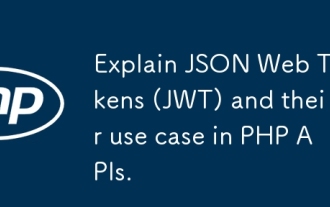
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
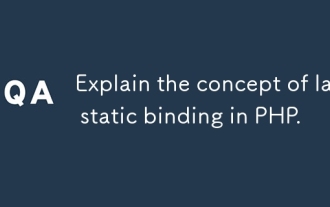
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
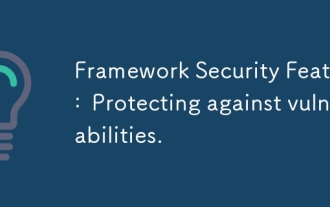
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
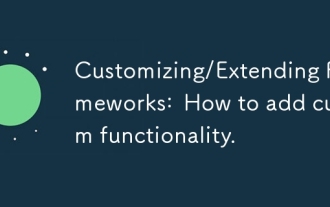
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
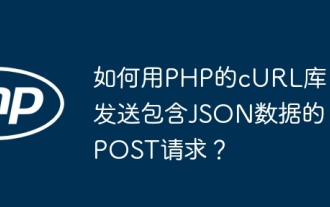
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
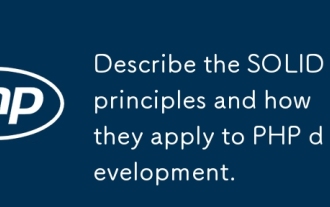
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
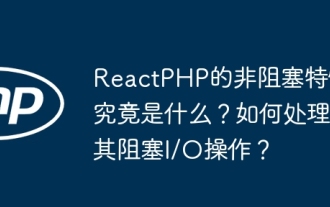
An official introduction to the non-blocking feature of ReactPHP in-depth interpretation of ReactPHP's non-blocking feature has aroused many developers' questions: "ReactPHPisnon-blockingbydefault...
