Introduction to PureComponent in React
This article mainly introduces the introduction of PureComponent in React, which has certain reference value. Now I share it with everyone. Friends in need can refer to it
React avoids repeated rendering
React builds and maintains an inner implementation within the rendered UI, which includes React elements returned from components. This implementation allows React to avoid unnecessary creation and association of DOM nodes, because doing so may be slower than directly manipulating JavaScript objects. It is called a "virtual DOM".
When a component's props or state change, React determines whether it is necessary to update the actual DOM by comparing the newly returned element with the previously rendered element. When they are not equal, React updates the DOM.
In some cases, your component can improve speed by overriding the shouldComponentUpdate lifecycle function, which is triggered before the re-rendering process begins. This function returns true by default, which allows React to perform updates:
shouldComponentUpdate(nextProps, nextState) { return true; }
Example
If you want the component to be only in props.color
or state.count## To re-render when the value of # changes, you can set
shouldComponentUpdate like this:
class CounterButton extends React.Component { constructor(props) { super(props); this.state = {count: 1}; } shouldComponentUpdate(nextProps, nextState) { if (this.props.color !== nextProps.color) { return true; } if (this.state.count !== nextState.count) { return true; } return false; } render() { return ( <button color={this.props.color} onClick={() => this.setState(state => ({count: state.count + 1}))}> Count: {this.state.count} </button> ); } }
shouldComponentUpdate only checks
props.color Changes in and
state.count. If these values do not change, the component will not be updated. As your components become more complex, you can use a similar pattern to do a "shallow comparison" of properties and values to determine whether the component needs to be updated. This pattern is so common that React provides a helper object to implement this logic - inherited from
React.PureComponent. The following code can more easily achieve the same operation:
class CounterButton extends React.PureComponent { constructor(props) { super(props); this.state = {count: 1}; } render() { return ( <button color={this.props.color} onClick={() => this.setState(state => ({count: state.count + 1}))}> Count: {this.state.count} </button> ); } }
if (this._compositeType === CompositeTypes.PureClass) { shouldUpdate = !shallowEqual(prevProps, nextProps) || !shallowEqual(inst.state, nextState); }
mutations, you cannot use it at this time.
class ListOfWords extends React.PureComponent { render() { return <p>{this.props.words.join(',')}</p>; } } class WordAdder extends React.Component { constructor(props) { super(props); this.state = { words: ['marklar'] }; this.handleClick = this.handleClick.bind(this); } handleClick() { // This section is bad style and causes a bug const words = this.state.words; words.push('marklar'); this.setState({words: words}); } render() { return ( <p> <button onClick={this.handleClick} /> <ListOfWords words={this.state.words} /> </p> ); } }
handleClick() { this.setState(prevState => ({ words: prevState.words.concat(['marklar']) })); } 或者 handleClick() { this.setState(prevState => ({ words: [...prevState.words, 'marklar'], })); }; 或者针对对象结构: function updateColorMap(oldObj) { return Object.assign({}, oldObj, {key: new value}); }
- Immutable: Once created, a collection cannot be changed at another point in time.
- Persistence: New collections can be created using the original collection and a mutation. The original collection remains available after the new collection is created.
- Structure sharing: The new collection is created using as much of the structure of the original collection as possible to minimize copy operations and improve performance.
// 常见的js处理 const x = { foo: 'bar' }; const y = x; y.foo = 'baz'; x === y; // true // 使用 immutable.js const SomeRecord = Immutable.Record({ foo: null }); const x = new SomeRecord({ foo: 'bar' }); const y = x.set('foo', 'baz'); x === y; // false
PureComponent What really works is only on some pure display components. If complex components are used
shallowEqual It’s basically impossible to pass that level. In addition, in order to ensure correct rendering during use, remember that
props and
state cannot use the same reference.
The difference between AngularJs and Angular’s commonly used instruction writing methods
The above is the detailed content of Introduction to PureComponent in React. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


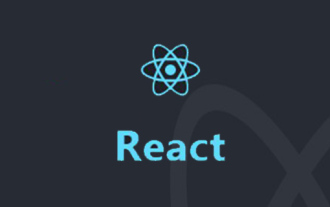
Calling method: 1. Calls in class components can be implemented by using React.createRef(), functional declaration of ref or props custom onRef attribute; 2. Calls in function components and Hook components can be implemented by using useImperativeHandle or forwardRef to throw a child Component ref is implemented.
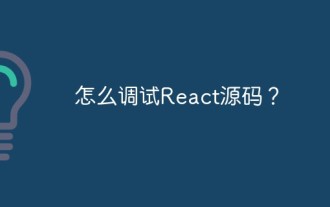
How to debug React source code? The following article will talk about how to debug React source code under various tools, and introduce how to debug the real source code of React in contributors, create-react-app, and vite projects. I hope it will be helpful to everyone!
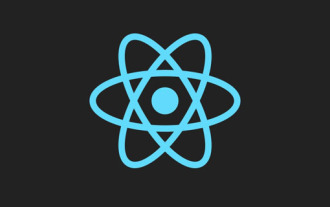
React custom Hooks are a way to encapsulate component logic in reusable functions. They provide a way to reuse state logic without writing classes. This article will introduce in detail how to customize encapsulation hooks.
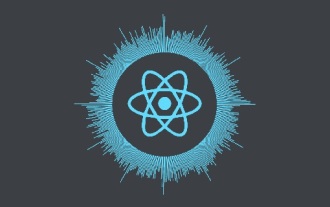
Why doesn’t React use Vite as the first choice for building applications? The following article will talk to you about the reasons why React does not recommend Vite as the default recommendation. I hope it will be helpful to everyone!
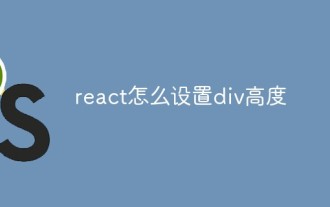
How to set the div height in react: 1. Implement the div height through CSS; 2. Declare an object C in the state and store the style of the change button in the object, then get A and reset the "marginTop" in C. That is Can.
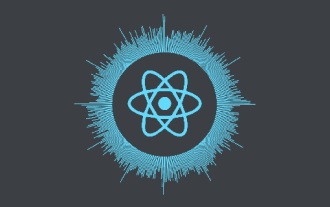
This article will share with you 7 great and practical React component libraries that are often used in daily development. Come and collect them and try them out!

This article will share with you 10 practical tips for writing simpler React code. I hope it will be helpful to you!
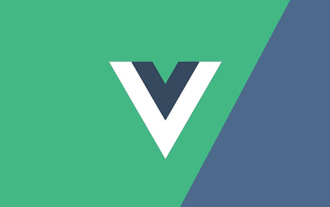
When developing front-end projects, state management is always an unavoidable topic. The Vue and React frameworks themselves provide some capabilities to solve this problem. However, there are often other considerations when developing large-scale applications, such as the need for more standardized and complete operation logs, time travel capabilities integrated in developer tools, server-side rendering, etc. This article takes the Vue framework as an example to introduce the differences in the design and implementation of the two state management tools, Vuex and Pinia.
