


PHP examples of Laravel authentication principles and detailed explanation of fully customized authentication
I have been learning the laravel framework recently, so the following article mainly introduces you to the relevant information about the Laravel authentication principle and completely customized authentication. The article introduces it in detail through sample code. Friends who need it can refer to it. The following is Let’s learn together with the editor
Preface
Laravel’s default auth function is already very comprehensive, but we often encounter There are some situations that need to be customized, such as the verification fields do not match the default ones, such as the need to satisfy user name and email authentication at the same time, etc. How to create a completely custom certificate? Rather than a tutorial, I prefer to introduce the working principle, so that it will be easier for you to modify or customize it yourself.
Authenticatable interface
Illuminate\Contracts\Auth\Authenticatable
Authenticatable defines a model that can be used for authentication Or the interface that the class needs to implement. In other words, if you need to use a custom class for authentication, you need to implement the methods defined by this interface.
// 获取唯一标识的,可以用来认证的字段名,比如 id,uuid public function getAuthIdentifierName(); // 获取该标示符对应的值 public function getAuthIdentifier(); // 获取认证的密码 public function getAuthPassword(); // 获取remember token public function getRememberToken(); // 设置 remember token public function setRememberToken($value); // 获取 remember token 对应的字段名,比如默认的 'remember_token' public function getRememberTokenName();
For example, your authentication model needs to use 'token' instead of 'password' as password verification. At this time, you can modify the return of the getAuthPassword() method. The value is 'token';
Authenticatable trait
Illuminate\Auth\Authenticatable
The Authenticatable trait defined in Laravel is also Laravel auth Trait used by the default User model. This trait defines the default authentication identifier of the User model as 'id', the password field as 'password', the field corresponding to the remember token as remember_token, and so on.
Some settings can be modified by overriding these methods of the User model.
Guard interface
Illuminate\Contracts\Auth\Guard
Guard interface defines an implementation that implements Authenticatable (can Authentication) model or class authentication methods and some common interfaces.
// 判断当前用户是否登录 public function check(); // 判断当前用户是否是游客(未登录) public function guest(); // 获取当前认证的用户 public function user(); // 获取当前认证用户的 id,严格来说不一定是 id,应该是上个模型中定义的唯一的字段名 public function id(); // 根据提供的消息认证用户 public function validate(array $credentials = []); // 设置当前用户 public function setUser(Authenticatable $user);
StatefulGuard interface
Illuminate\Contracts\Auth\StatefulGuard
StatefulGuard interface inherits from Guard interface, except inside Guard In addition to some basic interfaces defined, further and stateful Guard has also been added.
The newly added interfaces include these:
// 尝试根据提供的凭证验证用户是否合法 public function attempt(array $credentials = [], $remember = false); // 一次性登录,不记录session or cookie public function once(array $credentials = []); // 登录用户,通常在验证成功后记录 session 和 cookie public function login(Authenticatable $user, $remember = false); // 使用用户 id 登录 public function loginUsingId($id, $remember = false); // 使用用户 ID 登录,但是不记录 session 和 cookie public function onceUsingId($id); // 通过 cookie 中的 remember token 自动登录 public function viaRemember(); // 登出 public function logout();
Laravel provides 3 guards by default: RequestGuard, TokenGuard, and SessionGuard.
RequestGuard
Illuminate\Auth\RequestGuard
RequestGuard is a very simple The guard.RequestGuard is authenticated by passing in a closure. You can add a custom RequestGuard by calling Auth::viaRequest.
SessionGuard
Illuminate\Auth\SessionGuard
SessionGuard is the default for Laravel web authentication guard.
TokenGuard
Illuminate\Auth\TokenGuard
TokenGuard is suitable for stateless api authentication through token authentication.
UserProvider interface
Illuminate\Contracts\Auth\UserProvider
UserProvider interface defines the method of obtaining the authentication model, such as obtaining the model based on id, Get models based on email, etc.
// 通过唯一标示符获取认证模型 public function retrieveById($identifier); // 通过唯一标示符和 remember token 获取模型 public function retrieveByToken($identifier, $token); // 通过给定的认证模型更新 remember token public function updateRememberToken(Authenticatable $user, $token); // 通过给定的凭证获取用户,比如 email 或用户名等等 public function retrieveByCredentials(array $credentials); // 认证给定的用户和给定的凭证是否符合 public function validateCredentials(Authenticatable $user, array $credentials);
There are two user providers by default in Laravel: DatabaseUserProvider & EloquentUserProvider.
DatabaseUserProvider
Illuminate\Auth\DatabaseUserProvider
Get the authentication model directly through the database table.
EloquentUserProvider
Illuminate\Auth \EloquentUserProvider
Get the authentication model through the eloquent model
AuthManager
Illuminate\Auth\AuthManager
Guard is used to authenticate a Whether the user is authenticated successfully, UserProvider is used to provide the source of the authentication model, and functions such as managing guard and customizing guard according to the project's config are implemented through AuthManager.
AuthManager should be a bit like the Context class in the strategy pattern and the factory in the factory method. On the one hand, it manages the Guard, and on the other hand, it calls the specific strategy (Guard) method through the __call magic method.
The corresponding implementation class of Auth facade is AuthManager. AuthManager is registered as a singleton in the container and is used to manage all guard, user provider and guard proxy work.
Custom authentication
Based on the above knowledge, you can know that it is very simple to customize a authentication.
Create authentication model
Create a custom authentication model and implement the Authenticatable interface;
Create a custom UserProvider
Create a custom UserProvider, implement the UserProvider interface, and return the above custom authentication model;
Create a custom Guard
Create a custom Guard and implement the Guard or StatefulGuard interface
添加 guard creator 和 user provider creator 到 AuthManager 中
在 AppServiceProvider 的 boot 方法添加如下代码:
Auth::extend('myguard', function(){ ... return new MyGuard(); //返回自定义 guard 实例 ... }); Auth::provider('myuserprovider', function(){ return new MyUserProvider(); // 返回自定义的 user provider });
在 config\auth.php的 guards 数组中添加自定义 guard,一个自定义 guard 包括两部分: driver 和 provider.
'oustn' => [ 'driver' => 'myguard', 'provider' => 'myusers', ],
在 config\auth.php的 providers 数组中添加自定义 user provider.
'myusers' => [ 'driver' => 'myuserprovider' // 里面具体的字段可以根据你创建 user provider 需要的信息自由添加,可以通过 Auth::createUserProvider('myuserprovider') 创建 ],
设置 config\auth.php 的 defaults.guard 为 oustn.
The above is the detailed content of PHP examples of Laravel authentication principles and detailed explanation of fully customized authentication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
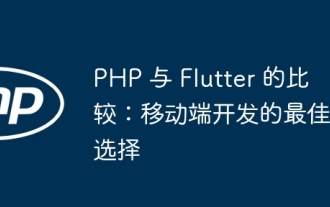
PHP and Flutter are popular technologies for mobile development. Flutter excels in cross-platform capabilities, performance and user interface, and is suitable for applications that require high performance, cross-platform and customized UI. PHP is suitable for server-side applications with lower performance and not cross-platform.
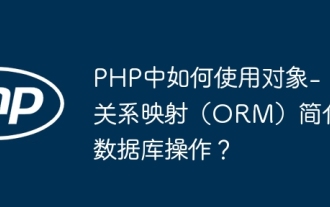
Database operations in PHP are simplified using ORM, which maps objects into relational databases. EloquentORM in Laravel allows you to interact with the database using object-oriented syntax. You can use ORM by defining model classes, using Eloquent methods, or building a blog system in practice.

PHP unit testing tool analysis: PHPUnit: suitable for large projects, provides comprehensive functionality and is easy to install, but may be verbose and slow. PHPUnitWrapper: suitable for small projects, easy to use, optimized for Lumen/Laravel, but has limited functionality, does not provide code coverage analysis, and has limited community support.
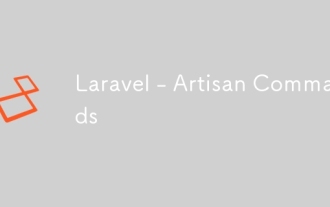
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
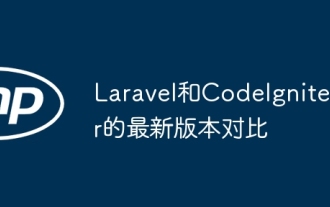
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
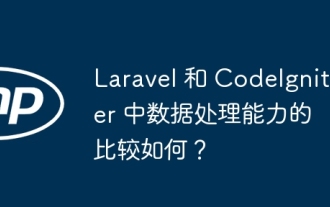
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
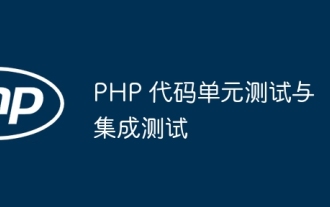
PHP Unit and Integration Testing Guide Unit Testing: Focus on a single unit of code or function and use PHPUnit to create test case classes for verification. Integration testing: Pay attention to how multiple code units work together, and use PHPUnit's setUp() and tearDown() methods to set up and clean up the test environment. Practical case: Use PHPUnit to perform unit and integration testing in Laravel applications, including creating databases, starting servers, and writing test code.
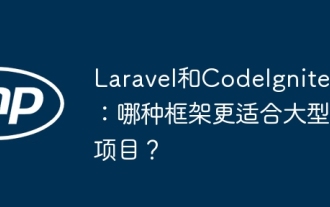
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
