


Static data members and static member functions of C++ classes
Static data members
·Declare with the keyword static
·When the data members of the declared class are static, no matter how many are created There is only one copy of static members for each class object
·Shared among all objects of the class, with static lifetime
·If there are no other initialization statements, the first one will be created after Object, all static data members are initialized to zero
·Defined and initialized outside the class, use the range resolution operator (::) to indicate the class to which it belongs
Example :
#include <iostream> using namespace std; class Box { public: static int count; //若该静态数据成员在private部分声明,则只能通过静态成员函数处理 Box(double l = 2.0, double b = 2.0, double h = 2.0) { cout << "One constructor was called." << endl; length = l, width = b, height = h; count++; //每创建一个对象时加1 } double Volume() { return length * width * height; } ~Box() { count--; } private: double length, width, height; }; //初始化类Box的静态成员 int Box::count = 0; int main(void) { Box Box1(3.3, 1.2, 1.5); Box Box2(8.5, 6.0, 2.0); cout << "Total objects: " << Box::count << endl; //输出对象的总数 return 0; }
Static member function
Declaring the member function as static can separate the function from any specific object of the class
·It can also be called when the class object does not exist. Use the class name plus the range resolution operator :: to access it
·Static member functions can only access static Member data, other static member functions and other functions outside the class
·Static member functions have a class scope and cannot access the this pointer of the class. You can use static member functions to determine whether some objects of the class have been Create
·To use static member functions to access non-static members, you need to pass the object
Example:
#include <iostream> using namespace std; class Box { public: static int count; Box(double l = 2.0, double b = 2.0, double h = 2.0) { cout <<"One constructor was called." << endl; length = l, width = b, height = h; count++; } double Volume() { return length * width * height; } static int getCount() { //静态成员函数 return count; } private: double length, width, height; }; int Box::count = 0; int main(void) { //在创建对象之前输出对象的总数 cout << "Inital Stage Count: " << Box::getCount() << endl; Box Box1(3.3, 1.2, 1.5); Box Box2(8.5, 6.0, 2.0); //在创建对象之后输出对象的总数 cout << "Final Stage Count: " << Box::getCount() << endl; return 0; }
Note:
The difference between static member functions and ordinary member functions:
Static member functions do not have this pointers and can only access static members (including static member variables and static Member function)
·Ordinary member functions have this pointer and can access any member in the class; while static member functions do not have this pointer
Use static members to understand the calling of constructors and destructors
#include <iostream> using namespace std; class A { friend class B; //类B是类A的友元 public: static int value; static int num; A(int x, int y) { xp = x, yp = y; value++; cout << "调用构造:" << value << endl; } void displayA() { cout << xp << "," << yp << endl; } ~A() { num++; cout << "调用析构:" << num << endl; } private: int xp, yp; }; class B { public: B(int x1, int x2) : mpt1(x1 + 2, x2 - 2), mpt2(x1, x2) { cout << "调用构造\n"; //mpt是类A的对象,有几个mpt,有关类A的操作便执行几次 } void set(int m, int n); void displayB(); ~B() { cout << "调用析构\n"; //析构函数在类结束前调用,类结束的时候释放类申请的空间 } private: A mpt1, mpt2; //将A类的对象声明为B类的私有数据成员 }; int A::value = 0; int A::num = 0; void B::set(int m, int n) { mpt1.xp = m * 2, mpt1.yp = n / 2; } void B::displayB() { mpt1.displayA(); } int main() { B p(10, 20); cout << "Hello world!" << endl; B displayB(); //通过友元,使类B输出类A的私有数据成员 return 0; }
Related articles:
C The use of static members and constant members
C Review Key Points Summary 5 Static Member Variables and Member Functions
The above is the detailed content of Static data members and static member functions of C++ classes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




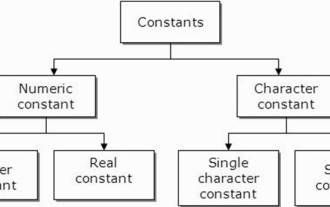
A constant is also called a variable and once defined, its value does not change during the execution of the program. Therefore, we can declare a variable as a constant referencing a fixed value. It is also called text. Constants must be defined using the Const keyword. Syntax The syntax of constants used in C programming language is as follows - consttypeVariableName; (or) consttype*VariableName; Different types of constants The different types of constants used in C programming language are as follows: Integer constants - For example: 1,0,34, 4567 Floating point constants - Example: 0.0, 156.89, 23.456 Octal and Hexadecimal constants - Example: Hex: 0x2a, 0xaa.. Octal
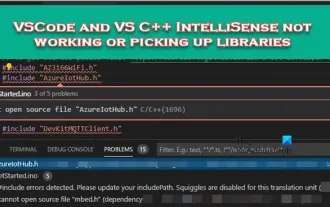
VS Code and Visual Studio C++ IntelliSense may not be able to pick up libraries, especially when working on large projects. When we hover over #Include<wx/wx.h>, we see the error message "CannotOpen source file 'string.h'" (depends on "wx/wx.h") and sometimes, autocomplete Function is unresponsive. In this article we will see what you can do if VSCode and VSC++ IntelliSense are not working or extracting libraries. Why doesn't my Intellisense work in C++? When working with large files, IntelliSense sometimes
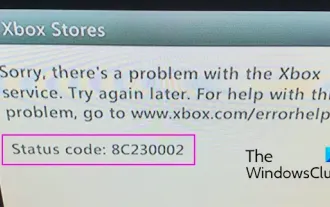
Are you unable to purchase or watch content on your Xbox due to error code 8C230002? Some users keep getting this error when trying to purchase or watch content on their console. Sorry, there's a problem with the Xbox service. Try again later. For help with this issue, visit www.xbox.com/errorhelp. Status Code: 8C230002 This error code is usually caused by temporary server or network problems. However, there may be other reasons, such as your account's privacy settings or parental controls, that may prevent you from purchasing or viewing specific content. Fix Xbox Error Code 8C230002 If you receive error code 8C when trying to watch or purchase content on your Xbox console
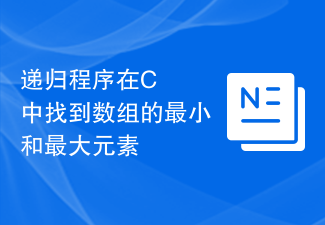
We take the integer array Arr[] as input. The goal is to find the largest and smallest elements in an array using a recursive method. Since we are using recursion, we will iterate through the entire array until we reach length = 1 and then return A[0], which forms the base case. Otherwise, the current element is compared to the current minimum or maximum value and its value is updated recursively for subsequent elements. Let’s look at various input and output scenarios for this −Input −Arr={12,67,99,76,32}; Output −Maximum value in the array: 99 Explanation &mi
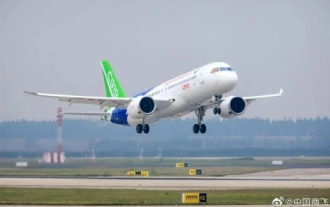
According to news on May 25, China Eastern Airlines disclosed the latest progress on the C919 passenger aircraft at the performance briefing meeting. According to the company, the C919 purchase agreement signed with COMAC has officially come into effect in March 2021, and the first C919 aircraft has been delivered by the end of 2022. It is expected that the aircraft will be officially put into actual operation soon. China Eastern Airlines will use Shanghai as its main base for commercial operations of the C919, and plans to introduce a total of five C919 passenger aircraft in 2022 and 2023. The company stated that future introduction plans will be determined based on actual operating conditions and route network planning. According to the editor's understanding, the C919 is China's new generation of single-aisle mainline passenger aircraft with completely independent intellectual property rights in the world, and it complies with internationally accepted airworthiness standards. Should
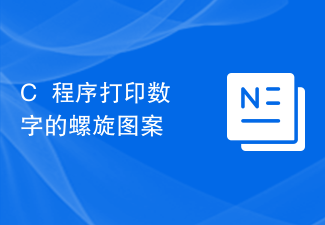
Displaying numbers in different formats is one of the basic coding problems of learning. Different coding concepts like conditional statements and loop statements. There are different programs in which we use special characters like asterisks to print triangles or squares. In this article, we will print numbers in spiral form, just like squares in C++. We take the number of rows n as input and start from the top left corner and move to the right, then down, then left, then up, then right again, and so on and so on. Spiral pattern with numbers 123456724252627282982340414243309223948494431102138474645321120373635343312191817161514
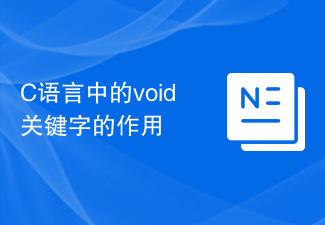
void in C is a special keyword used to represent an empty type, which means data without a specific type. In C language, void is usually used in the following three aspects. The function return type is void. In C language, functions can have different return types, such as int, float, char, etc. However, if the function does not return any value, the return type can be set to void. This means that after the function is executed, it does not return a specific value. For example: voidhelloWorld()
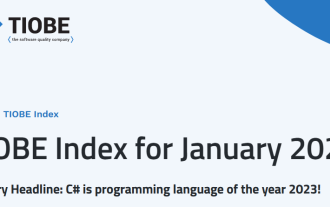
According to the TIOBE Programming Community Index, one of the benchmarks for measuring the popularity of programming languages, it is evaluated by collecting data from engineers, courses, vendors and search engines around the world. The TIOBE Index in January 2024 was released recently, and the official programming language rankings for 2023 were announced. C# won the TIOBE 2023 Programming Language of the Year. This is the first time that C# has won this honor in 23 years. TIOBE's official press release stated that C# has been in the top 10 for more than 20 years. Now it is catching up with the four major languages and has become the programming language with the largest growth in one year (+1.43%). It well-deserved to win this award. Ranked second are Scratch (+0.83%) and Fortran (+0
