


[js]: Detect user input, text box default style setting, design table style to achieve select all and invert selection
Topic 1: Detecting user input:
##Topic requirements: Write a user registration page Check whether the username is less than 6 characters and whether the password is more than 8 characters. If the requirements are not met, highlight the text box; The code is as follows:<!doctype html> <html> <head> <meta charset="utf-8"> <title>用户注册页面</title> <style> .bg {background-color: red; } </style> </head> <body> <!-----id适用于js中的---> <input type="text" name="name" id="name" /><br> <input type="text" name="pwd" id="pwd" /><br> <input type="submit" value="注册" id="submit" /> <script> // 编写一个用户注册页面 // 检测用户名是否是6位以下,密码是否是8位以上,如果不满足要求高亮显示文本框 ; var nameText = document.getElementById('name'); var pwdText = document.getElementById('pwd'); var submit = document.getElementById('submit'); //给submit按钮注册事件 submit.onclick = function () { if(nameText.value.length < 6 && nameText.value.length > 0) { nameText.className = ''; } else { nameText.className = 'bg'; } if(pwdText.value.length > 8 && pwdText.value.length < 16) { pwdText.className = ''; } else { pwdText.className = 'bg'; } //取消submit的默认行为的执行 if里面不需要这句了 如果加了肯能会影响后续代码的执行 return false; } </script> </body> </html>
Topic 2: Setting the default style of the text box:
<!doctype html> <html> <head> <meta charset="utf-8"> <title>设置文本框中的默认样式</title> <style> .bg {color: gray; } </style> </head> <body> <input type="text" id="textSearch" class="bg" value="请输入关键字" > <input type="button" value="搜索"> <script> //注册事件 //如果文本框获得焦点 当内容是请输入关键字 清空内容 文字颜色恢复默认的黑色 var textSearch = document.getElementById('textSearch'); textSearch.onfocus = function () { if(textSearch.value === '请输入关键字') { this.value = ''; //把this的属性恢复为默认值 这里作用是把文字颜色变为黑色 this.className = ''; } } // 当失去焦点的时候onblur。如果文本框中的内容为空 设置文本框中内容为 请输入关键字 设置字体颜色为gray textSearch.onblur = function () { // if (textSearch.value === '') {} // 这一句不太好的 这个要是用户第一个输入的是空格的话那么就会误判 我们可以用它的长度来判断 if (textSearch.value.length === 0) { this.className = 'bg'; this.value = '请输入关键字'; } } </script> </body> </html>
Topic 3: : Design a table style to realize the function of selecting all and inverting the selection
Function to be realized:1 When clicking the select all button (parent's checkbox), keep the selected state of the child's checkbox consistent with the parent's checkbox
2 Register click events for all child's checkboxes, Click the child's checkbox. If one of the child's checkboxes is not selected, the parent's checkbox is also not selected
3 Inverse selection
Code example:
<!doctype html> <html> <head> <meta charset="utf-8"> <title>全选反选</title> <style> * { padding: 0; margin: 0; } .wrap { width: 300px; margin: 100px auto 0; } table { border-collapse: collapse; border-spacing: 0; border: 1px solid #c0c0c0; width: 300px; } th, td { border: 1px solid #d0d0d0; color: #404060; padding: 10px; } th { background-color: #09c; font: bold 16px "微软雅黑"; color: #fff; } td { font: 14px "微软雅黑"; } tbody tr { background-color: #f0f0f0; } tbody tr:hover { cursor: pointer; background-color: #fafafa; } </style> </head> <body> <p class="wrap"> <table> <thead> <tr> <th> <input type="checkbox" id="father" /> </th> <th>商品</th> <th>价钱</th> </tr> </thead> <tbody id="son"> <tr> <td> <input type="checkbox" /> </td> <td>iPhone8</td> <td>8000</td> </tr> <tr> <td> <input type="checkbox" /> </td> <td>iPad Pro</td> <td>5000</td> </tr> <tr> <td> <input type="checkbox" /> </td> <td>iPad Air</td> <td>2000</td> </tr> <tr> <td> <input type="checkbox" /> </td> <td>Apple Watch</td> <td>2000</td> </tr> </tbody> </table> <input type="button" value=" 反 选 " id="btn"> <script> // 1 点击全选按钮(父的checkbox)的时候,让子的checkbox的选中状态跟父的checkbox保持一致 //1.1 给父级的check注册事件 //获取父级checkbox var father = document.getElementById('father'); //获取所有的子级checkbox //注意这一句用选择器的获得元素的写法 var sons = document.querySelectorAll('#son input[type=checkbox]'); var len = sons.length; father.onclick = function () { //1.2遍历这个容器中的所有元素 让所有的子级checkbox的状态都等于父级的状态 for(var i = 0;i < len; i++) { //注意这一句话是核心 让子级的checkbox的状态等于父级 sons[i].checked = this.checked; } } // 2 给所有的子的checkbox注册点击事件,点击子的checkbox 如果有一个子的checkbox没有选中,父的checkbox也不选中 // 2.1 给所有的子的checkbox注册点击事件 // 核心代码封装成方法 便于使用 function step2 () { //2.2 只要子级有一个是false那么父级也就是false //2.2 定义一个变量用于父级的状态 这个fatherIsTrue必须在点击事件这里面 father的赋值也是 因为每个点击事件需要判断所有的子级的状态 在外面的话是实现不了的 var fatherIsTrue = true; for (var j = 0; j < len; j++) { if (!sons[j].checked) { fatherIsTrue = false; break; } } father.checked = fatherIsTrue; } for(var i = 0;i < len; i++) { sons[i].onclick = function () { step2(); } } //3 反选 // 给反选按钮注册一个事件 var btn = document.getElementById('btn'); btn.onclick = function () { for(var i = 0; i < len; i++) { sons[i].checked = !sons[i].checked; } //写到这里我门会有一个问题就是反选不能控制父级 但是呢我们的第二步已经完成这个问题了 所以我们把第二步 //的核心代码分装成一个方法 直接调用即可 直接粘贴复制过来不太好 step2(); } </script> </p> </body> </html>
Note: This sentence is the core. Let the status of the child's checkbox be equal to the parent's sons [i].checked = this.checked;
How to select and invert all js checkboxes
js Implementation code for setting the style of the selected row
The above is the detailed content of [js]: Detect user input, text box default style setting, design table style to achieve select all and invert selection. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


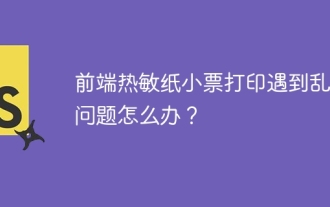
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
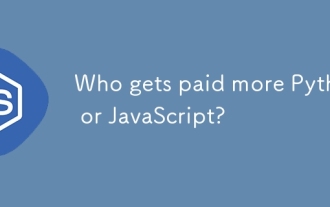
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
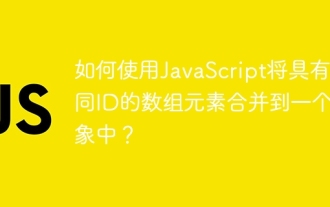
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
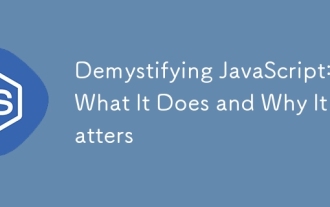
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
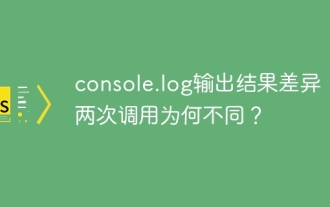
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
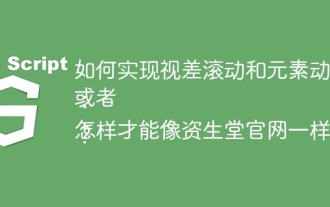
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
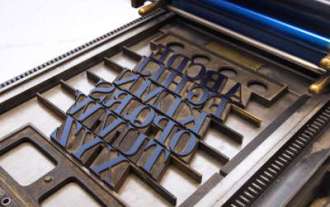
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
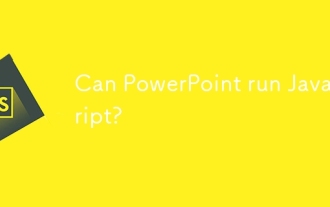
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
