JS inheritance method--case description
1. Prototype chain: Use prototypes to let one reference type inherit the properties and methods of another reference type
Each constructor has a prototype object, and the prototype object contains a pointer to the constructor pointer, and instances contain an internal pointer to the prototype object.
Basic pattern for implementing originality chain:
function SuperType(){ this.colors =[“red”, “blue”, “green”]; } function SubType(){ } SubType.prototype = new SuperType(); var instance1 = new SubType(); instance1.colors.push(“black”); alert(instance1.colors); //red, blue, green, black var instance2 = new SubType(); alert(instance2.colors); //red, blue, green, black
The final result: intance points to the prototype of SubType, and the prototype of SubType points to the prototype of SuperType, and SuperType inherits Object
The default prototype of all functions is an instance of Object
Problem: There will be problems with reference type values
For example, if an instance of a subclass is created, if If the attributes of a subclass instance are modified, then other subclasses will be affected when creating them. The code is as follows:
function SuperType(){ this.colors =[“red”, “blue”, “green”]; } function SubType(){ } SubType.prototype = new SuperType(); var instance1 = new SubType(); instance1.colors.push(“black”); alert(instance1.colors); //red, blue, green, black var instance2 = new SubType(); alert(instance2.colors); //red, blue, green, black
The above results indicate that the attribute values of other instances will be affected
2. Borrowing the constructor: calling the supertype constructor inside the subtype constructor
function SuperType(){ this.colors =[“red”, “blue”, “green”]; } function SubType{}( SuperType.call(this); //继承了SuperType。通过调用SuperType的构造函数,借用其构造结构 } var instance1 = new SubType(); instance1.colors.push(“black”); alert(intance1.colors); //red,blue,green,black var instance2 = new SubType(); alert(instance2.colors); //red,blue,green
Use this method to pass parameters to the supertype constructor in the subclass constructor, as follows:
function SuperType(name){ this.name = name; } function SubType(){ SuperType.call(this,“Nicholas”); //传入参数,利用这个参数初始化父类构造函数中的name this.age = 29; } var instance = new SubType(); alert(instance.name); //Nicholas alert(instance.age); //29
Problem: Inconvenient to reuse
3. Combined inheritance: Use the prototype chain to inherit the prototype properties and methods, and borrow the constructor to implement the instance Inheritance of attributes
Sample code:
function SuperType(name){ this.name = name; this.colors = [“red”, “blue”,“green”]; } SuperType.prototype.sayName = function(){ //定义了一个方法,该方法在继承的子类中也可以用 alert(this.name); } function SubType(name, age){ SuperType.call(this, name); //继承SuperType的一部分,this指SubType, this.age = age; //自己新定义的属性age也可以进行赋值 } SubType.prototype = new SuperType(); //利用原型继承,可以使用父类的方法(见原型链继承) SubType.prototype.sayAge = function(){ //定义SubType特有的新方法 alert(this.age); } var instance1 = new SubType(“Martin”, 10); instance1.colors.push(“black”); alert(instance1.colors); //red,blue,green,black instance1.sayName(); //Martin instance1.sayAge(); //10 var instance2 = new SubType(“Greg”, 27); alert(instance2.colors); //red,blue,green instance2.sayName(); //Greg instance2.sayAge(); //27
Related recommendations:
Detailed examples of inheritance methods in JS
Several ways to implement inheritance in JS
The above is the detailed content of JS inheritance method--case description. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




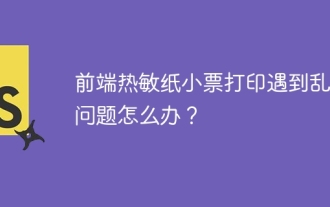
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
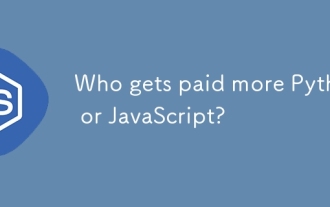
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
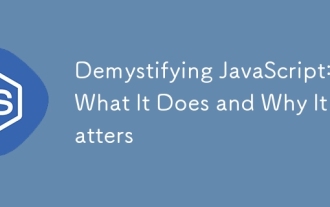
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
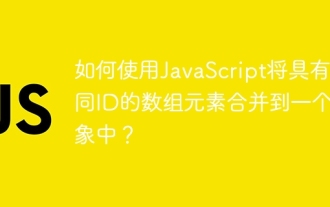
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
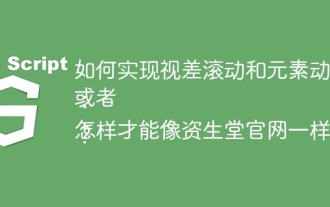
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
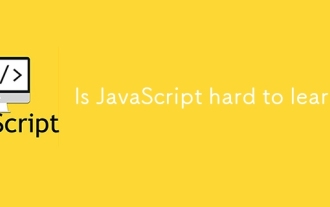
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
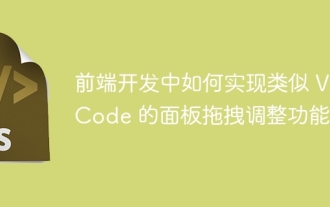
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
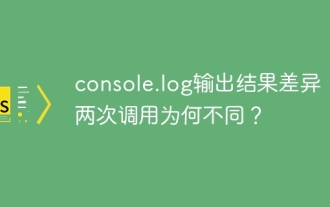
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
