


PHP uses bit operations to implement addition, subtraction, multiplication and division of integers and test them (code example)
The content of this article is about PHP using bit operations to implement addition, subtraction, multiplication and division of integers and testing (code examples). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you. help.
<?php /** * Created by PhpStorm. * User: Mch * Date: 8/10/18 * Time: 23:51 * 只用位运算不用算数运算实现整数的 + - * / */ class Arithmetic { const MAX_INTEGER = 2147483647; const MIN_INTEGER = -2147483648; /** * @param int $a * @param int $b * @return int $a + $b; */ public static function add(int $a, int $b) : int { $sum = $a; while ($b) { $sum = $a ^ $b; // 不考虑进位 $b = ($a & $b) << 1; // 只考虑进位 $a = $sum; } return $sum; } /** * 相反数 <= 二进制表达取反+1(补码) * @param int $n * @return int */ private static function negateNumber(int $n) : int { return self::add(~$n, 1); } /** * a-b = a + (-b) * @param int $a * @param int $b * @return int */ public static function minus(int $a, int $b) : int { return self::add($a, self::negateNumber($b)); } /** * @param int $a * @param int $b * @return int $a * $b */ public static function multiple(int $a, int $b) : int { $res = 0; while ($b) { if (($b & 1)) { $res = self::add($res, $a); } $a <<= 1; $b >>= 1; } return $res; } private static function isNegative(int $n) : bool { return $n < 0; } /** * a/b a = MIN_INTEGER, b!=MIN_INTEGER ? * @param int $a * @param int $b * @return int */ private static function p(int $a, int $b) : int { $x = self::isNegative($a) ? self::negateNumber($a) : $a; $y = self::isNegative($b) ? self::negateNumber($b) : $b; $res = 0; for ($i = 31; $i >-1; $i = self::minus($i, 1)) { if (($x >> $i)>=$y) { $res |= (1 << $i); $x = self::minus($x, $y<<$i); } } return self::isNegative($a) ^ self::isNegative($b) ? self::negateNumber($res):$res; } /** * @param int $a * @param int $b * @return int $a / $b */ public static function pide(int $a, int $b) : int { if ($b === 0) { throw new RuntimeException("pisor is 0"); } if ($a === self::MIN_INTEGER && $b === self::MIN_INTEGER) { return 1; } else if ($b === self::MIN_INTEGER) { return 0; } else if ($a === self::MIN_INTEGER) { $res = self::p(self::add($a, 1), $b); return self::add($res, self::p(self::minus($a, self::multiple($res, $b)), $b)); } else { return self::p($a, $b); } } }
TEST:
echo Arithmetic::add(1, 2).PHP_EOL; // 3 echo Arithmetic::minus(10, 3).PHP_EOL; // 7 echo Arithmetic::multiple(5, 3).PHP_EOL; // 15 echo Arithmetic::pide(-2147483648, 1).PHP_EOL; // -2147483648 echo Arithmetic::pide(-15, 3).PHP_EOL; // -5
Related recommendations:
Code for simple interaction between PHP and html forms
phpHow to generate classes for HTML files? How to generate html file class in php
The above is the detailed content of PHP uses bit operations to implement addition, subtraction, multiplication and division of integers and test them (code example). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


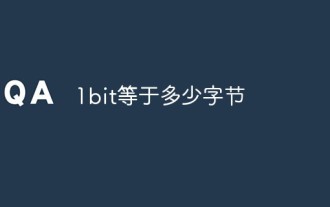
1 bit is equal to one-eighth of a byte. In the binary number system, each 0 or 1 is a bit (bit), and a bit is the smallest unit of data storage; every 8 bits (bit, abbreviated as b) constitute a byte (Byte), so "1 byte ( Byte) = 8 bits”. In most computer systems, a byte is an 8-bit (bit) long data unit. Most computers use a byte to represent a character, number, or other character.
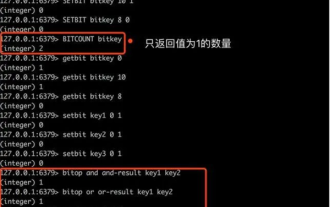
The redis test code in this article is based on the following environment: Operating system: MacOS 64-bit version: Redis5.0.764bit Operating mode: standalonemode Redis bit operation reids bit operation is also called bit array operation and bitmap. It provides four commands: SETBIT, GETBIT, BITCOUNT, and BITTOP. For manipulating binary bit arrays. Let’s first look at a wave of basic operation examples SETBIT syntax: SETBITkeyoffsetvalue is: command key offset 0/1 The setbit command is used to write the binary bit setting value of the specified offset in the bit array. The offset starts counting from 0 and is only allowed Write 1 or 0,
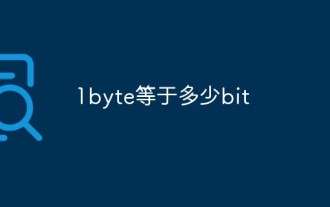
1byte equals 8bit. Data storage is in "byte" (Byte) as the unit, and data transmission is mostly in "bit" (bit) as the unit. One bit represents a 0 or 1 (that is, binary), and every 8 bits (bit) form a Byte is the smallest unit of information; therefore, "1Byte=8bit".
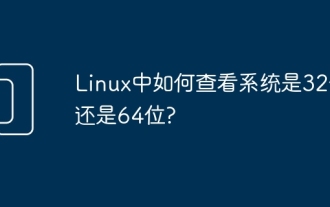
CentOS is a Linux distribution that originated from RHEL and is compiled in accordance with the regulations of open source code. Moreover, it maintains functional compatibility with RHEL and is a free, open source operating system that users can use and modify without paying copyright fees. So does CentOS distinguish between 32-bit and 64-bit in Linux? Please see below for details. CentOS distinguishes between 32-bit and 64-bit! The main differences: CentOS32bit system is mainly released for PC; CentOS64bit system is mainly aimed at large-scale scientific computing; 64bitLinux system is mainly installed on 64bit hardware system; 32bit
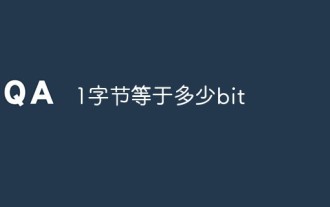
1 byte is equal to 8 bits. In the binary number system, each 0 or 1 is a bit (bit), and a bit is the smallest unit of data storage; every 8 bits (bit, abbreviated as b) constitute a byte (Byte), so "1 byte ( Byte) = 8 bits”. In most computer systems, a byte is an 8-bit unit of data. Most computers use a byte to represent a character, number, or other character.
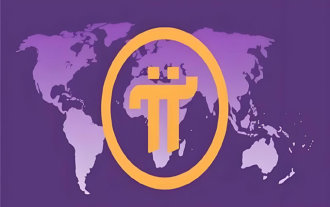
Pi Coin In-depth Analysis: Pi Coin (π), a cryptocurrency that coexists with opportunities and challenges, has attracted more than 47 million users worldwide since its birth in 2018 with its unique "mobile mining" mechanism. This article will explore the basic information, ecosystem, application scenarios and the controversy surrounding Picoin, helping you to fully understand this controversial digital asset. Pi Coin Core Information Chinese Name: Pai Coin English Name: Pi Coin, π Coin Common Abbreviation: π Official Website: https://minepi.com/ Founder: Nicolas Kokkalis (Technical Head, Ph.D., Stanford University) and Chengdiao
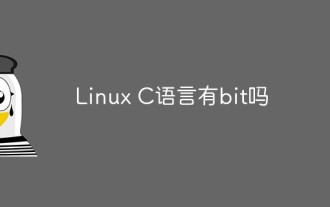
Linux C language has bit; in the microcontroller C language, bit is a new keyword, often used to define a "bit variable"; the method of defining bit type data in C language is: 1. Define through sbit or bit; 2. Defined through bit fields (in structures); 3. Defined through combined bit operators.
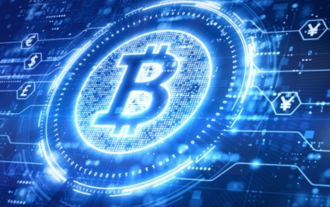
Top ten virtual currency trading platforms in 2025: 1. Binance; 2. OKX; 3. gateio; 4. bitget, etc. When choosing an exchange, it is recommended that you comprehensively consider security, transaction fees, and Choose the platform that suits you best for user experience and other factors.
