Detailed analysis of String class in Java
Strings are widely used in Java programming. Strings are objects in Java. Java provides the String class to create and operate strings. This article will introduce the contents of the String class in detail.
Create a string
The simplest way to create a string is as follows:
String greeting = "php Chinese website";
Encountered in the code When it is a string constant, the value here is "php中文网"", and the compiler will use this value to create a String object.
Like other objects, you can use keywords and constructs Method to create a String object.
The String class has 11 construction methods, which provide different parameters to initialize the string, such as providing a character array parameter:
StringDemo.java file code:
public class StringDemo{ public static void main(String args[]){ char[] helloArray = { 'p', 'h', 'p'}; String helloString = new String(helloArray); System.out.println( helloString ); } }
The compilation and running results of the above example are as follows:
php
Note: String class is immutable, so once you create a String object, its value cannot be changed Changed (see the notes section for details).
If you need to make a lot of modifications to the string, you should choose to use the StringBuffer & StringBuilder class.
String length
Use Methods for obtaining information about an object are called accessor methods.
One accessor method of the String class is the length() method, which returns the number of characters contained in the string object.
The following After the code is executed, the len variable is equal to 14:
StringDemo.java file code:
public class StringDemo { public static void main(String args[]) { String site = "www.php.cn"; int len = site.length(); System.out.println( "php中文网网址长度 : " + len ); } }
The compilation and running results of the above example are as follows:
php中文网网址 : 14
Connection string
String class provides a method to concatenate two strings:
string1.concat(string2);
Return string2 to connect string1 to a new string. You can also use concat for string constants () method, such as:
"我的名字是 ".concat("php");
More commonly used is to use the ' ' operator to connect strings, such as:
"Hello," + " php" + "!"
The result is as follows:
"Hello, runoob!"
The following is an example :
StringDemo.java file code:
public class StringDemo { public static void main(String args[]) { String string1 = "php中文网网址:"; System.out.println("1、" + string1 + "www.php.cn"); } }
The compilation and running results of the above example are as follows:
1、php中文网网址:www.php.cn
Create formatted string
We know the output formatting Numbers can use the printf() and format() methods.
The String class uses the static method format() to return a String object instead of a PrintStream object.
The static method format() of the String class can Used to create reusable formatted strings, not just for one-time printout.
As shown below:
System.out.printf("浮点型变量的值为 " + "%f, 整型变量的值为 " + " %d, 字符串变量的值为 " + "is %s", floatVar, intVar, stringVar);
You can also write like this
String fs; fs = String.format("浮点型变量的值为 " + "%f, 整型变量的值为 " + " %d, 字符串变量的值为 " + " %s", floatVar, intVar, stringVar)
String method
The following are the methods supported by the String class. For more details, see Java String API Documentation:
SN(serial number) | Method description |
---|---|
char charAt(int index) |
Returns the char value at the specified index. |
int compareTo(Object o) |
Compare this string to another object. |
int compareTo(String anotherString) |
Compares two strings lexicographically. |
int compareToIgnoreCase(String str) |
Compares two strings lexicographically, regardless of case. |
String concat(String str) |
Concatenates the specified string to the end of this string. |
boolean contentEquals(StringBuffer sb) |
Returns true if and only if the string has the same order of characters as the specified StringBuffer. |
static String copyValueOf(char[] data) |
Returns a String representing this sequence of characters in the specified array. |
static String copyValueOf(char[] data, int offset, int count) |
Returns a String representing this sequence of characters in the specified array. |
boolean endsWith(String suffix) |
Tests whether this string ends with the specified suffix. |
boolean equals(Object anObject) |
Compares this string to the specified object. |
boolean equalsIgnoreCase(String anotherString) |
Compares this String to another String, regardless of case. |
byte[] getBytes() |
Encode this String into a sequence of bytes using the platform's default character set, and store the result in a new byte array. |
byte[] getBytes(String charsetName) |
Encodes this String into a sequence of bytes using the specified character set and stores the result in a new byte array. |
void getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin) |
Copies characters from this string to a target character array. |
int hashCode() |
Returns the hash code of this string. |
int indexOf(int ch) |
Returns the index of the first occurrence of the specified character in this string. |
int indexOf(int ch, int fromIndex) |
Returns the index of the first occurrence of the specified character in this string, starting the search at the specified index. |
int indexOf(String str) |
Returns the index of the first occurrence of the specified substring in this string. |
int indexOf(String str, int fromIndex) |
Returns the index of the first occurrence of the specified substring in this string, starting at the specified index. |
String intern() |
Returns the canonical representation of a string object. |
int lastIndexOf(int ch) |
Returns the index of the last occurrence of the specified character in this string. |
int lastIndexOf(int ch, int fromIndex) |
Returns the index of the last occurrence of the specified character in this string, starting from the specified index and performing a reverse search. |
int lastIndexOf(String str) |
Returns the index of the rightmost occurrence of the specified substring in this string. |
int lastIndexOf(String str, int fromIndex) |
Returns the index of the last occurrence of the specified substring in this string, starting the reverse search from the specified index. |
int length() |
Returns the length of this string. |
boolean matches(String regex) |
Tells whether this string matches the given regular expression. |
boolean regionMatches(boolean ignoreCase, int toffset, String other, int ooffset, int len) |
Tests whether two string ranges are equal. |
boolean regionMatches(int toffset, String other, int ooffset, int len) |
Tests whether two string ranges are equal. |
String replace(char oldChar, char newChar) |
Returns a new string obtained by replacing all occurrences of oldChar with newChar. |
String replaceAll(String regex, String replacement) |
Replaces all substrings of this string that match the given regular expression with the given replacement. |
String replaceFirst(String regex, String replacement) |
Replaces the first substring of this string matching the given regular expression with the given replacement. |
String[] split(String regex) |
Splits this string based on matches of the given regular expression. |
String[] split(String regex, int limit) |
Split this string based on matching the given regular expression. |
boolean startsWith(String prefix) | Tests whether this string starts with the specified prefix. |
35 | boolean startsWith(String prefix, int toffset) Tests whether the substring of this string starting at the specified index begins with the specified prefix. |
36 | CharSequence subSequence(int beginIndex, int endIndex) Returns a new character sequence that is a subsequence of this sequence. |
37 | String substring(int beginIndex) Returns a new string that is a substring of this string. |
38 | String substring(int beginIndex, int endIndex) Returns a new string that is a substring of this string. |
39 | char[] toCharArray() Convert this string to a new character array. |
40 | String toLowerCase() Converts all characters in this String to lowercase using the rules of the default locale. |
41 | String toLowerCase(Locale locale) Converts all characters in this String to lowercase using the rules of the given Locale. |
42 | String toString() Returns this object itself (it's already a string!). |
43 | String toUpperCase() Converts all characters in this String to uppercase using the rules of the default locale. |
44 | String toUpperCase(Locale locale) Converts all characters in this String to uppercase using the rules of the given Locale. |
45 | String trim() Returns a copy of the string, ignoring leading and trailing whitespace. |
46 | static String valueOf(primitive data type x) Returns the string representation of the x parameter of the given data type. |
Related recommendations:
Detailed analysis of the performance of String, StringBuffer, and StringBuilder classes in Java
Detailed explanation of Math and String format class instances in java
The above is the detailed content of Detailed analysis of String class in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


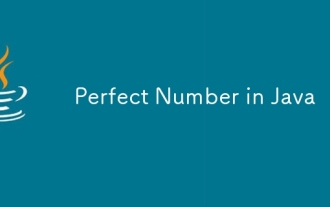
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
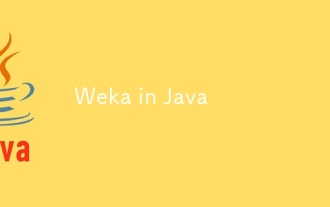
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
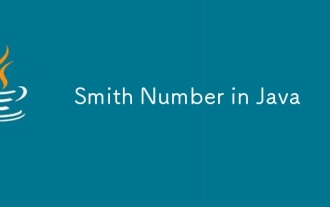
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
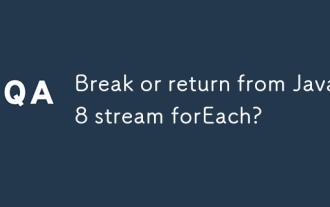
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
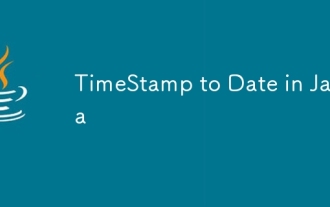
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
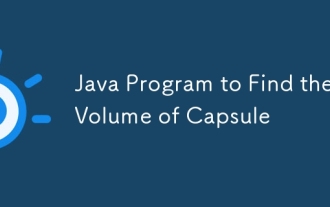
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
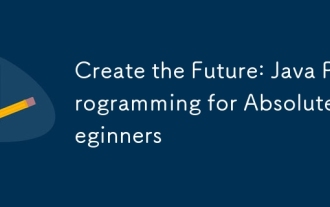
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
