


What can the react framework do? Detailed introduction to react framework (with complete usage examples)
React is a JavaScript framework for building "predictable" and "declarative" web user interfaces, which has enabled Facebook to develop web applications faster. Let’s take a look at the content of the article
1. Features:
1. Simply describe your application at any point in time What it should look like is that React will automatically manage UI updates when the data changes.
2. Declarative When the data changes, React is conceptually the same as clicking F5. In fact, it only updates part of the change. React is about building reusable components. In fact, with React all you do is build components. Encapsulation makes component code reuse, testing, and separation of concerns easier.
In addition, there are the following points to note:
React is not an MVC framework
React does not use templates
Responsive update is very simple
2. Main principles
In traditional web applications, operating DOM is generally Direct update operation, but we know that DOM updates are usually expensive. In order to reduce operations on the DOM as much as possible, React provides a different and powerful way to update the DOM instead of direct DOM operations. It is Virtual DOM, a lightweight virtual DOM, which is an object abstracted by React, describing what the dom should look like and how it should be presented. The real DOM is updated through this Virtual DOM, and this Virtual DOM manages the update of the real DOM.
Why can it be faster through this extra layer of Virtual DOM operations? This is because React has a diff algorithm. Updating the Virtual DOM does not guarantee that it will affect the real DOM immediately. React will wait until the event loop ends, and then use this diff algorithm to calculate the smallest value by comparing the current new dom representation with the previous one. Steps to update the real DOM.
Components Components
Nodes in the DOM tree are called elements, but here it is different, Virtual DOM It is called commponent. The node of Virtual DOM is a complete abstract component, which is composed of commponents.
Note: The use of components is extremely important in React, because the existence of components makes calculating DOM diff more efficient.
State and Render
How does React present the real DOM, how to render components, when to render, and how to update synchronously. This requires a brief understanding of State and Render. Rendered. The state attribute contains some data needed to define the component. When the data changes, Render will be called to re-render. Here, the data can only be updated through the provided setState method.
Let’s take a look at the official website demo first:
<!DOCTYPE html> <html> <head> <script src="http://fb.me/react-0.12.1.js"></script> <script src="http://fb.me/JSXTransformer-0.12.1.js"></script> </head> <body> <p id="example"></p> <script type="text/jsx"> React.render( <h1 id="Hello-nbsp-world">Hello, world!</h1>, document.getElementById('example') ); </script> </body> </html>
is very simple. When accessed by a browser, you can see the words Hello, world!. JSXTransformer.js supports parsing JSX syntax. JSX is a syntax that can write html code in Javascript. If you don't like it, React also provides native Javascript methods. (If you want to see more, go to the PHP Chinese website React Reference Manual column to learn)
Another demo:
<html> <head> <title>Hello React</title> <script src="http://fb.me/react-0.12.1.js"></script> <script src="http://fb.me/JSXTransformer-0.12.1.js"></script> <script src="http://code.jquery.com/jquery-1.10.0.min.js"></script> <script src="http://cdnjs.cloudflare.com/ajax/libs/showdown/0.3.1/showdown.min.js"></script> <style> #content{ width: 800px; margin: 0 auto; padding: 5px 10px; background-color:#eee; } .commentBox h1{ background-color: #bbb; } .commentList{ border: 1px solid yellow; padding:10px; } .commentList .comment{ border: 1px solid #bbb; padding-left: 10px; margin-bottom:10px; } .commentList .commentAuthor{ font-size: 20px; } .commentForm{ margin-top: 20px; border: 1px solid red; padding:10px; } .commentForm textarea{ width:100%; height:50px; margin:10px 0 10px 2px; } </style> </head> <body> <p id="content"></p> <script type="text/jsx"> var staticData = [ {author: "张飞", text: "我在写一条评论~!"}, {author: "关羽", text: "2货,都知道你在写的是一条评论。。"}, {author: "刘备", text: "哎,咋跟这俩逗逼结拜了!"} ]; var converter = new Showdown.converter();//markdown /** 组件结构: <CommentBox> <CommentList> <Comment /> </CommentList> <CommentForm /> </CommentBox> */ //评论内容组件 var Comment = React.createClass({ render: function (){ var rawMarkup = converter.makeHtml(this.props.children.toString()); return ( <p className="comment"> <h2 className="commentAuthor"> {this.props.author}: </h2> <span dangerouslySetInnerHTML={{__html: rawMarkup}} /> </p> ); } }); //评论列表组件 var CommentList = React.createClass({ render: function (){ var commentNodes = this.props.data.map(function (comment){ return ( <Comment author={comment.author}> {comment.text} </Comment> ); }); return ( <p className="commentList"> {commentNodes} </p> ); } }); //评论表单组件 var CommentForm = React.createClass({ handleSubmit: function (e){ e.preventDefault(); var author = this.refs.author.getDOMNode().value.trim(); var text = this.refs.text.getDOMNode().value.trim(); if(!author || !text){ return; } this.props.onCommentSubmit({author: author, text: text}); this.refs.author.getDOMNode().value = ''; this.refs.text.getDOMNode().value = ''; return; }, render: function (){ return ( <form className="commentForm" onSubmit={this.handleSubmit}> <input type="text" placeholder="Your name" ref="author" /><br/> <textarea type="text" placeholder="Say something..." ref="text" ></textarea><br/> <input type="submit" value="Post" /> </form> ); } }); //评论块组件 var CommentBox = React.createClass({ loadCommentsFromServer: function (){ this.setState({data: staticData}); /*
For the sake of convenience, I will not go here Server-side, you can try it yourself
$.ajax({ url: this.props.url + "?_t=" + new Date().valueOf(), dataType: 'json', success: function (data){ this.setState({data: data}); }.bind(this), error: function (xhr, status, err){ console.error(this.props.url, status, err.toString()); }.bind(this) }); */ }, handleCommentSubmit: function (comment){ //TODO: submit to the server and refresh the list var comments = this.state.data; var newComments = comments.concat([comment]); //这里也不向后端提交了 staticData = newComments; this.setState({data: newComments}); }, //初始化 相当于构造函数 getInitialState: function (){ return {data: []}; }, //组件添加的时候运行 componentDidMount: function (){ this.loadCommentsFromServer(); this.interval = setInterval(this.loadCommentsFromServer, this.props.pollInterval); }, //组件删除的时候运行 componentWillUnmount: function() { clearInterval(this.interval); }, //调用setState或者父级组件重新渲染不同的props时才会重新调用 render: function (){ return ( <p className="commentBox"> <h1 id="Comments">Comments</h1> <CommentList data={this.state.data}/> <CommentForm onCommentSubmit={this.handleCommentSubmit} /> </p> ); } }); //当前目录需要有comments.json文件 //这里定义属性,如url、pollInterval,包含在props属性中 React.render( <CommentBox url="comments.json" pollInterval="2000" />, document.getElementById("content") ); </script> </body> </html>
Effect:
This article ends here (if you want to see more, go to the PHP Chinese websiteReact User Manual column), if you have any questions, you can leave a message below.
The above is the detailed content of What can the react framework do? Detailed introduction to react framework (with complete usage examples). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
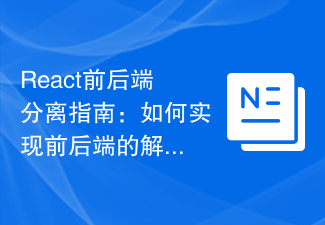
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
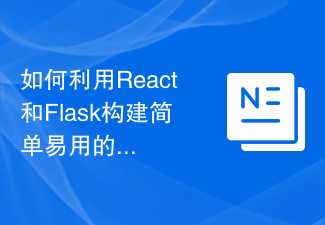
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
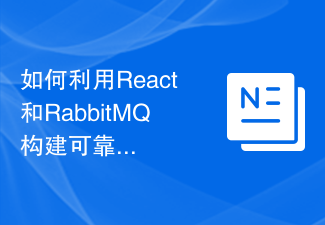
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
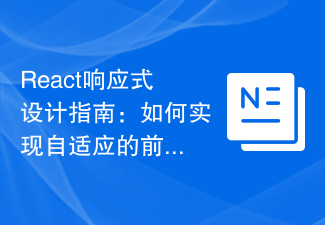
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
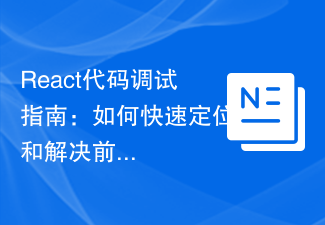
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
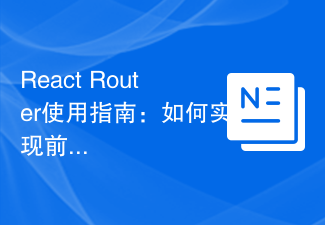
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
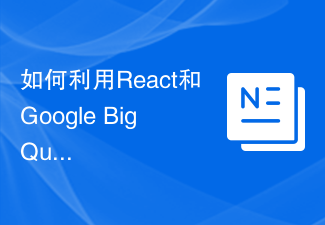
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
