How to create a webSocket web chat room in Java (with code)
The content of this article is about how to create a webSocket web chat room in Java (with code). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
1. What is webSocket
WebSocket is a network communication protocol and a persistence protocol. RFC6455 defines its communication standard.
WebSocket is a protocol for full-duplex communication on a single TCP connection that HTML5 began to provide.
2. Why use webSocket
Traditional web communication uses http technology. The http protocol is a stateless, connectionless, one-way application layer protocol. A request can only correspond to one response. Communication requests can only be issued by the client, and the server responds to the request. Therefore, the server is very passive in sending responses. This passive response is doomed to the fact that the server cannot proactively push responses to the client in a timely manner. If the server has continuous status changes, it will be very difficult for the client to obtain it. By frequently using asynchronous ajax to continuously obtain requests to implement long polling, this is particularly performance consuming and inefficient. (Keep shaking hands, or keep living for a long time).
And webSocket allows full-duplex communication between the server and the client. As long as the connection is established once, the connection can be maintained. Once a connection is established, both parties can communicate with each other without the need for multiple handshakes.
3. Implement WEB chat room
Add pom.xml and introduce jar package
<dependency> <groupId>javax</groupId> <artifactId>javaee-api</artifactId> <version>7.0</version> <scope>provided</scope> </dependency>
2. Create html and js file
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>在线聊天室</title> <script type="text/javascript" src="./static/jquery-3.2.0.min.js"></script> <!-- 最新版本的 Bootstrap 核心 CSS 文件 --> <link rel="stylesheet" href="https://cdn.bootcss.com/bootstrap/3.3.7/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous"> <!-- 最新的 Bootstrap 核心 JavaScript 文件 --> <script src="https://cdn.bootcss.com/bootstrap/3.3.7/js/bootstrap.min.js" integrity="sha384-Tc5IQib027qvyjSMfHjOMaLkfuWVxZxUPnCJA7l2mCWNIpG9mGCD8wGNIcPD7Txa" crossorigin="anonymous"></script> </head> <body> <div> <div><span>聊天室</span> <button class="btn btn-warning" onclick="doClose();">退出聊天</button> </div> <div><textarea class="form-control" style="width: 40%;" rows="3" id="contentInp"></textarea></div><hr/> <div><button class="btn btn-danger" onclick="doClear();">清空会话框</button></div> <div id="content">开始聊天<br/></div> </div> </body> <script type="text/javascript"> var ws = new WebSocket("ws://localhost:8080/ws/websocket"); //controller层url $(function(){ $("#contentInp").keyup(function(evt){ if(evt.which == 13){ //enter键发送消息 var htm = $("#contentInp").val(); doSend(htm); $("textarea").empty(); } }); }) ws.onopen = function(){ appendHtm("连接成功!"); } // 从服务端接收到消息,将消息回显到聊天记录区 ws.onmessage = function(evt){ appendHtm(evt.data); } ws.onerror = function(){ appendHtm("连接失败!"); } ws.onclose = function(){ appendHtm("连接关闭!"); } function appendHtm(htm){ ($("#content")[0]).innerHTML += htm +"<br/>" } // 注销登录 function doClose(){ ws.close(); } // 发送消息 function doSend(htm){ // ($("#content")[0]).innerHTML += htm +"<br/>" ws.send(htm); } function doClear(){ $("#content").empty(); } </script> </html>
3.Backend java code
package controller; import java.io.IOException; import java.util.concurrent.CopyOnWriteArraySet; import javax.net.ssl.SSLSession; import javax.websocket.OnClose; import javax.websocket.OnError; import javax.websocket.OnMessage; import javax.websocket.OnOpen; import javax.websocket.Session; import javax.websocket.server.ServerEndpoint; @ServerEndpoint("/websocket") public class WebScoketServer { private static Integer onlineNum = 0; //当前在线人数,线程必须设计成安全的 private static CopyOnWriteArraySet<WebScoketServer> arraySet = new CopyOnWriteArraySet<WebScoketServer>(); //存放每一个客户的的WebScoketServer对象,线程安全 private Session session; /** * 连接成功 * @param session 会话信息 */ @OnOpen public void onOpen(Session session) { this.session =session; arraySet.add(this); this.addOnlineNum(); System.out.println("有一个新连接加入,当前在线 "+this.getOnLineNum()+" 人"); } /** * 连接关闭 */ @OnClose public void onClose() { arraySet.remove(this); this.subOnlineNum(); System.out.println("有一个连接断开,当前在线 "+this.getOnLineNum()+" 人"); } /** * 连接错误 * @param session * @param error */ @OnError public void onError(Session session, Throwable error) { System.err.println("发生错误!"); error.printStackTrace(); } /** * 发送消息,不加注解,自己选择实现 * @param msg * @throws IOException */ public void onSend(String msg) throws IOException { this.session.getBasicRemote().sendText(msg); } /** * 收到客户端消息回调方法 * @param session * @param msg */ @OnMessage public void onMessage(Session session, String msg) { System.out.println("消息监控:"+msg); for (WebScoketServer webScoketServer : arraySet) { try { webScoketServer.onSend(msg); } catch (IOException e) { e.printStackTrace(); continue; } } } /** * 增加一个在线人数 */ private synchronized void addOnlineNum() { onlineNum++; } /** * 减少一个在线人数 */ private synchronized void subOnlineNum() { onlineNum--; } private Integer getOnLineNum() { return onlineNum; } }
The above is the detailed content of How to create a webSocket web chat room in Java (with code). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


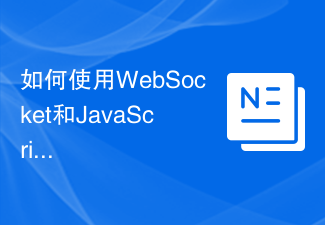
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
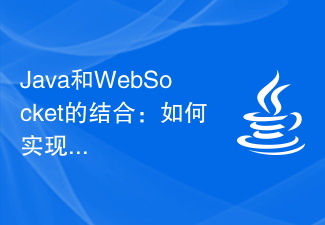
With the continuous development of Internet technology, real-time video streaming has become an important application in the Internet field. To achieve real-time video streaming, the key technologies include WebSocket and Java. This article will introduce how to use WebSocket and Java to implement real-time video streaming playback, and provide relevant code examples. 1. What is WebSocket? WebSocket is a protocol for full-duplex communication on a single TCP connection. It is used on the Web
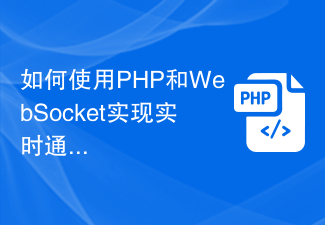
With the continuous development of Internet technology, real-time communication has become an indispensable part of daily life. Efficient, low-latency real-time communication can be achieved using WebSockets technology, and PHP, as one of the most widely used development languages in the Internet field, also provides corresponding WebSocket support. This article will introduce how to use PHP and WebSocket to achieve real-time communication, and provide specific code examples. 1. What is WebSocket? WebSocket is a single
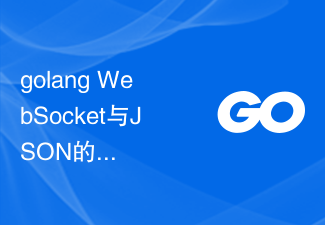
The combination of golangWebSocket and JSON: realizing data transmission and parsing In modern Web development, real-time data transmission is becoming more and more important. WebSocket is a protocol used to achieve two-way communication. Unlike the traditional HTTP request-response model, WebSocket allows the server to actively push data to the client. JSON (JavaScriptObjectNotation) is a lightweight format for data exchange that is concise and easy to read.
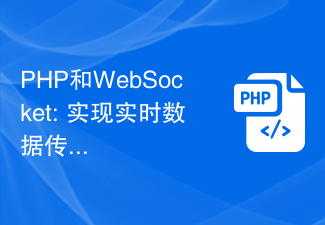
PHP and WebSocket: Best Practice Methods for Real-Time Data Transfer Introduction: In web application development, real-time data transfer is a very important technical requirement. The traditional HTTP protocol is a request-response model protocol and cannot effectively achieve real-time data transmission. In order to meet the needs of real-time data transmission, the WebSocket protocol came into being. WebSocket is a full-duplex communication protocol that provides a way to communicate full-duplex over a single TCP connection. Compared to H
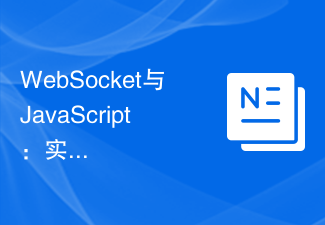
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
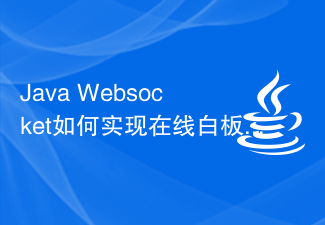
How does JavaWebsocket implement online whiteboard function? In the modern Internet era, people are paying more and more attention to the experience of real-time collaboration and interaction. Online whiteboard is a function implemented based on Websocket. It enables multiple users to collaborate in real-time to edit the same drawing board and complete operations such as drawing and annotation. It provides a convenient solution for online education, remote meetings, team collaboration and other scenarios. 1. Technical background WebSocket is a new protocol provided by HTML5. It implements
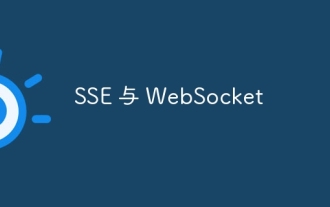
In this article, we will compare Server Sent Events (SSE) and WebSockets, both of which are reliable methods for delivering data. We will analyze them in eight aspects, including communication direction, underlying protocol, security, ease of use, performance, message structure, ease of use, and testing tools. A comparison of these aspects is summarized as follows: Category Server Sent Event (SSE) WebSocket Communication Direction Unidirectional Bidirectional Underlying Protocol HTTP WebSocket Protocol Security Same as HTTP Existing security vulnerabilities Ease of use Setup Simple setup Complex performance Fast message sending speed Affected by message processing and connection management Message structure Plain text or binary Ease of use Widely available Helpful for WebSocket integration
