Python error and exception handling
The content of this article is about error and exception handling in Python. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
1: Syntax errors syntax errors
Familiar with grammar!
2: Exception
①When printing error information, the exception type is displayed as the built-in name of the exception, and the specific information is displayed in the form of a call stack
②Common exceptions :
NameError,
ZeropisionError,
TypeError
SyntaxError
IndexError Index exceeds sequence range
KeyError Requesting a dictionary key that does not exist
IOError use using using using using using using out out out out out out out out out out Out out out of ’ Try to access unknown object properties
Three: Exception handling
while True: try: x = int(input("Please enter a number: ")) break except ValueError: print("Oops! That was no valid number. Try again ")
①First, execute the try clause (between the keyword try and the keyword except Statements between)
② If no exception occurs, ignore the except clause and end after the try clause is executed.
③If an exception occurs during the execution of the try clause, the rest of the try clause will be ignored.
④If the exception type matches the name after except, the corresponding except clause will be executed. Finally execute the code after the try statement.
⑤If an exception does not match any except, then the exception will be passed to the upper try.
⑥A try statement may contain multiple except clauses to handle different specific exceptions. At most one branch will be executed.
⑦An except clause can handle multiple exceptions at the same time. These exceptions will be placed in parentheses to become a tuple.
except (RuntimeError, TypeError, NameError): pass
⑨The try except statement also has an optional else clause. If this clause is used, it must be placed after all except clauses. This clause will be executed when no exception occurs in the try clause.
raise the only one The parameter specifies the exception to be thrown.
It must be an exception instance or an exception class (that is, a subclass of Exception)
The exception class inherits from the Exception class and can be inherited directly or indirectly.
When creating a module that may throw a variety of different exceptions,
A common approach is to create a basic exception class for this package,
try: raise KeyboardInterrupt finanlly: print("dooo")
If an exception is thrown in the try clause (or in the except and else clauses),
but not If any except intercepts it, then the exception will be thrown again after the finally clause is executed.
Purpose: Defensive program
Requires logic checking during runtime
Reference: "The best time to use assertions in Python 》
# ---------------------------------------------------------------------# # 异常处理 # ---------------------------------------------------------------------# while True: try: x = int(input("Please enter a number: ")) break except ValueError: print("Oops! That was no valid number. Try again ") # ---------------------------------------------------------------------# # 抛出异常 # ---------------------------------------------------------------------# """ try: raise NameError('HiThere') except NameError: print('An exception flew by!') raise """ # ---------------------------------------------------------------------# # 用户自定义异常 # ---------------------------------------------------------------------# class MyError(Exception): # 继承自Exception def __init__(self, value): # 构造函数重写 self.value = value def __str__(self): return repr(self.value) try: raise MyError(2*2) # 抛出异常 except MyError as e: print('My exception occurred, value:', e.value) # 当创建一个模块有可能抛出多种不同的异常时, # 一种通常的做法是为这个包建立一个基础异常类, # 然后基于这个基础类为不同的错误情况创建不同的子类 class Error(Exception): """Base class for exceptions in this module.""" pass class InputError(Error): """Exception raised for errors in the input. Attributes: expression -- input expression in which the error occurred message -- explanation of the error """ def __init__(self, expression, message): self.expression = expression self.message = message class TransitionError(Error): """Raised when an operation attempts a state transition that's not allowed. Attributes: previous -- state at beginning of transition next -- attempted new state message -- explanation of why the specific transition is not allowed """ def __init__(self, previous, next1, message): self.previous = previous self.next = next1 self.message = message try: raise InputError(2*2, 45) # 抛出异常 except InputError: # 处理异常 print('My exception occurred') # ---------------------------------------------------------------------# # 定义清理行为 # ---------------------------------------------------------------------# def divide(x, y): try: result = x / y except ZeroDivisionError: print("division by zero!") else: # 没有异常的时执行 print("result is", result) finally: # 无论在任何情况下都会执行的清理行为 print("executing finally clause") # 一个异常在 try 子句里(或者在 except 和 else 子句里)被抛出, # 而又没有任何的 except 把它截住,那么这个异常会在 finally 子句执行后再次被抛出 # divide("2", "1") #从测试代码
The above is the detailed content of Python error and exception handling. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
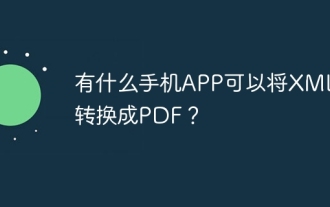
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
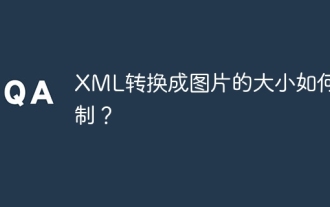
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
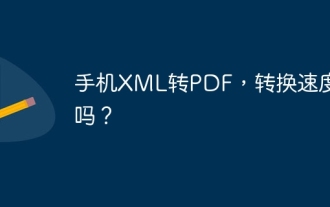
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
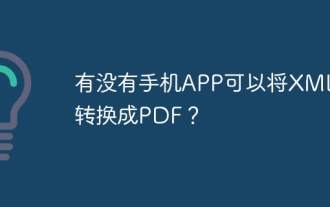
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
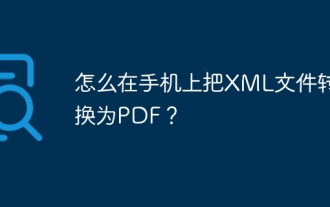
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
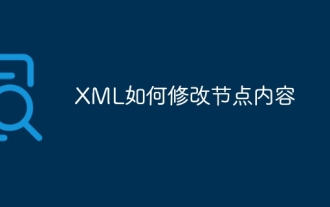
XML node content modification skills: 1. Use the ElementTree module to locate nodes (findall(), find()); 2. Modify text attributes; 3. Use XPath expressions to accurately locate them; 4. Consider encoding, namespace and exception handling; 5. Pay attention to performance optimization (avoid repeated traversals)
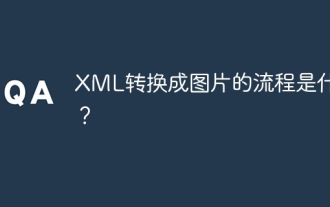
To convert XML images, you need to determine the XML data structure first, then select a suitable graphical library (such as Python's matplotlib) and method, select a visualization strategy based on the data structure, consider the data volume and image format, perform batch processing or use efficient libraries, and finally save it as PNG, JPEG, or SVG according to the needs.
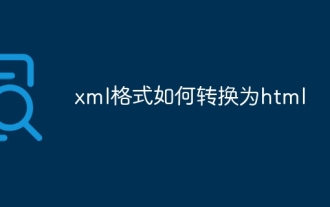
The correct way to convert XML to HTML is to extract XML structure data into a tree structure using a parser. Building an HTML structure based on the extracted data. Avoid inefficient and error-prone string operations.
