A brief introduction to InvocationHandler of Java dynamic proxy
This article brings you a brief introduction to InvocationHandler of Java dynamic proxy. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
There are very in-depth articles on the Internet about Java's dynamic proxy, Proxy and InvocationHandler concepts. In fact, these concepts are not that complicated. Now we understand what InvocationHandler is through the simplest example. It is worth mentioning that InvocationHandler is widely used in Spring framework implementation, which means that if we understand InvocationHandler thoroughly, we will lay a solid foundation for future Spring source code learning.
Develop an interface that contains two methods that can greet "hello" or "goodbye" to the specified person.
public interface IHello { void sayHello(String name); void sayGoogBye(String name); }
Create a simple class to implement this IHello interface.
public class Helloimplements implements IHello { @Override public void sayHello(String name) { System.out.println("Hello " + name); } @Override public void sayGoogBye(String name) { System.out.println(name+" GoodBye!"); } }
Consuming this implementation class is nothing special so far.
Now suppose we receive this requirement: The boss requires that every time the implementation class greets someone, the details of the greeting must be recorded in a log file. For the sake of simplicity, we print the following line of statement before greeting to simulate the logging action.
System.out.println("问候之前的日志记录...");
You may say, this is not simple? Directly modify the corresponding method of Helloimplements and insert this line of log into the corresponding method.
However, the boss’s request is that you are not allowed to modify the original Helloimplements class. In real scenarios, Helloimplements may be provided by a third-party jar package, and we have no way to modify the code.
You may say that we can use the proxy pattern in the design pattern, that is, create a new Java class as a proxy class, and also implement IHello interface and then pass an instance of the Helloimplements class into the proxy class. Although we are required not to modify the code of Helloimplements, we can write the logging code in the proxy class. The complete code is as follows:
public class StaticProxy implements IHello { private IHello iHello; public void setImpl(IHello impl){ this.iHello = impl; } @Override public void sayHello(String name) { System.out.println("问候之前的日志记录..."); iHello.sayHello(name); } @Override public void sayGoogBye(String name) { System.out.println("问候之前的日志记录..."); iHello.sayGoogBye(name); } static public void main(String[] arg) { Helloimplements hello = new Helloimplements(); StaticProxy proxy = new StaticProxy(); proxy.setImpl(hello); proxy.sayHello("Jerry"); } }
This approach can achieve the requirements:
Let’s look at how to use InvocationHandler to achieve the same effect. .
InvocationHandler是一个JDK提供的标准接口。看下面的代码: import java.lang.reflect.InvocationHandler; import java.lang.reflect.Method; import java.lang.reflect.Proxy; public class DynaProxyHello implements InvocationHandler { private Object delegate; public Object bind(Object delegate) { this.delegate = delegate; return Proxy.newProxyInstance( this.delegate.getClass().getClassLoader(), this.delegate .getClass().getInterfaces(), this); } public Object invoke(Object proxy, Method method, Object[] args) throws Throwable { Object result = null; try { System.out.println("问候之前的日志记录..."); // JVM通过这条语句执行原来的方法(反射机制) result = method.invoke(this.delegate, args); } catch (Exception e) { e.printStackTrace(); } return result; }
The bind method in the above code is very similar to the setImpl method of my previous proxy class StaticProxy, but the input parameter type of this bind method is more universal. The logging code is written in the method invoke.
See how to use it:
static public void main(String[] arg) { DynaProxyHello helloproxy = new DynaProxyHello(); Helloimplements hello = new Helloimplements(); IHello ihello = (IHello) helloproxy.bind(hello); ihello.sayHello("Jerry"); }
The execution effect is exactly the same as that of StaticProxy solution.
Let’s debug it first. When the bind method is executed, the method Proxy.newProxyInstance is called, and the instance of the Helloimplements class is passed in.
We observe the ihello variable returned by the statement IHello ihello = (IHello) helloproxy.bind(hello) in the debugger. Although its static type is IHello, please note that when observing its actual type in the debugger, it is not an instance of Helloimplements, but one processed by the JVM, including the line of log we handwritten in the invoke method. Document the code. The ihello type is $Proxy0.
When the sayHello method of this variable processed by the JVM is called, the JVM automatically transfers the call to DynaProxyHello.invoke:
So, in the invoke method, our handwritten logging code is executed, and then the original sayHello code is executed through Java reflection.
Some friends may ask, your InvocationHandler seems more complicated than the static proxy StaticProxy? what is the benefit?
Assume that the boss's needs have changed again, and different logging strategies should be used in the methods of calling greetings and saying goodbye.
Let’s see how to use InvocationHandler to implement it elegantly:
I hope this example can give everyone an understanding of Java’s dynamic proxy InvocationHandler. Get the most basic understanding.
The above is the detailed content of A brief introduction to InvocationHandler of Java dynamic proxy. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


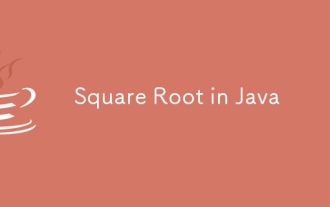
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
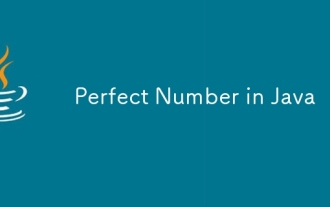
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
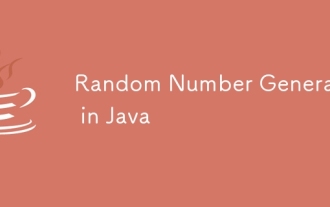
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
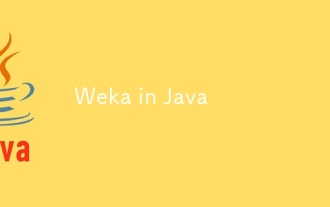
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
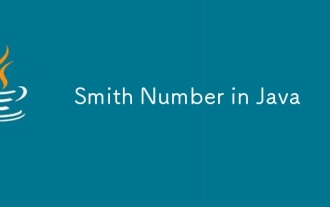
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
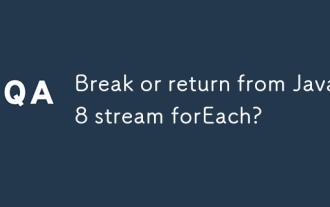
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
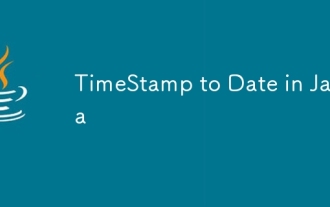
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
