


A brief discussion on object-oriented programming in python (code examples)
This article brings you a brief discussion of object-oriented programming in python (code examples). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
1. The first case---create a class
#__author:"吉" #date: 2018/10/27 0027 #function: # 设计类: ''' 类名:首字母大写,见名思意 属性:驼峰原则 行为:见名思意,驼峰法 说明:类不占空间,实例化对象占用空间! ''' # 格式,object是父类,超类 ''' 类名(object): 属性 行为 ''' class Peoson(object): name = 'zhanglei' age = 24 weight = 70 def run(self): print("跑!") def eat(self): print('吃')
2. Instantiate objects using classes
#__author:"吉" #date: 2018/10/27 0027 #function: # 设计类: ''' 类名:首字母大写,见名思意 属性:驼峰原则 行为:见名思意,驼峰法 说明:类不占空间,实例化对象占用空间! ''' # 格式,object是父类,超类 ''' 类名(object): 属性 行为 ''' class Peoson(object): name = 'zhanglei' age = 24 weight = 70 def run(self): print("跑!") def eat(self): print('吃') def changeName(self,name): self.name = name # 实例化对象 ''' 格式:对象名= 类名(参数列表信息) ''' peoson1 = Peoson() print(peoson1.name,peoson1.age,peoson1.weight) print(peoson1.eat()) print(peoson1.run()) # 原理 ''' 变量是在栈区,对象是在堆区。 '''
3. Accessing the properties and methods of objects
#__author:"吉" #date: 2018/10/27 0027 #function: # 设计类: ''' 类名:首字母大写,见名思意 属性:驼峰原则 行为:见名思意,驼峰法 说明:类不占空间,实例化对象占用空间! ''' # 格式,object是父类,超类 ''' 类名(object): 属性 行为 ''' class Peoson(object): name = 'zhanglei' age = 24 weight = 70 def run(self): print("跑!") def eat(self): print('吃') def changeName(self,name): self.name = name # 实例化对象 ''' 格式:对象名= 类名(参数列表信息) ''' peoson1 = Peoson() # 访问属性 ''' 变量是在栈区,对象是在堆区。 ''' print(peoson1.name,peoson1.age,peoson1.weight) peoson1.name = 'jiji' peoson1.age = 33 peoson1.weight = 90 print(peoson1.name,peoson1.age,peoson1.weight) peoson1.changeName('lala') print(peoson1.name,peoson1.age,peoson1.weight)
4. Constructor
#__author:"吉勇佳" #date: 2018/10/27 0027 #function: ''' 构造函数:__init__() 是在创建类的时候自动调用,不写出这个 构造函数的话,默认是一个空的构造函数什么页不执行。 ''' class Peoson(object): def __init__(self,name,age,height,weight): self.name = name self.age = age self.height = height self.weight = weight def run(self): print("跑!") def eat(self): print('吃') def changeName(self,name): self.name = name # 实例化对象 p1 = Peoson("jiyongjia",24,177,78) print(p1.name,p1.age,p1.height,p1.weight) p1.changeName('zhanglei') print(p1.name,p1.age,p1.height,p1.weight) # self 原理 ''' 1、哪个对象调用,self就代表那个对象。 '''
5. Use of self.__class__() to create instances and destructors
class Peoson(object): def __init__(self,name,age,height,weight): self.name = name self.age = age self.height = height self.weight = weight # 这里是析构函数 def __del__(self): print("我是析构函数") def run(self): print("跑!") def eat(self): print('吃') def changeName(self,name): self.name = name # 创建对象函数 self.__class__ 是代表类名的 def createObj(self,name): p=self.__class__(name,24,56,89) print(p.name,p.age,p.weight,p.height) # 即 执行p1的一个方法即可创建新的对象。 p1 = Peoson("丽丽",33,53,222) print(p1.name,p1.age,p1.height,p1.weight) p1.createObj("狗熊") ''' 输出:丽丽 33 53 222 狗熊 24 89 56 我是析构函数 我是析构函数 ''' print(p1.name)
6. Comparison of __str__() and __repr__()
''' 重写 __str__() str方法是给用户用的,用于返回用户需要的结果信息、 __repr__() 如果换成__repr__()是得到与str相同的结果。是在黑屏终端直接敲对象名再回车的方法 注意:在没有str方法但是有repr的时候,repr 就相当于str,只是repr用于黑屏终端 ''' class Peoson(object): def __init__(self,name,age,height,weight): self.name = name self.age = age self.height = height self.weight = weight # 这里是析构函数 def __del__(self): print("我是析构函数") def run(self): print("跑!") def eat(self): print('吃') def changeName(self,name): self.name = name # 创建对象函数 self.__class__ 是代表类名的 def createObj(self,name): p=self.__class__(name,24,56,89) print(p.name,p.age,p.weight,p.height) # 如果换成__repr__()是得到相同的结果。是在黑屏终端直接敲对象名再回车的方法 def __str__(self): pass # return "这里是str" # return self.name #返回多个值的话用如下 return "%s-%d-%d" % (self.name,self.age,self.weight) # 如果要打印出所有的属性信息呢? p1 = Peoson("嘉嘉",44,222,336) # 不写def __str__()方法的时候,打印出的是该对象的地址信息 print(p1) ''' 输出:<__main__.Peoson object at 0x0000000002564978> ''' # 写了__str__()函数是 打印出的自己需要返回的信息数据,此时p1等价于p1.__str__() print(p1)
7. Exercise-Object-oriented homework:
The gun design bullet design is missing one bullet at a time. If there is no bullet, it will prompt that you cannot shoot.
#__author:"吉" #date: 2018/10/27 0027 #function: # 枪设计子弹 设计一次少一个子弹,没子弹了提示无法射击 class Gun(object): def __init__(self,name,bulletNum): self.name = name self.bulletNum = bulletNum def shot(self): self.bulletNum =self.bulletNum - 1 if self.bulletNum+1 != 0: print("此枪型号是:%s,此次射击成功!子弹减少1发,余量为:%d" %(self.name,self.bulletNum)) else: print("*************射击失败,子弹数为0.已经为您子弹加弹5发!请重新射击!**************") self.bulletNum = 5 gun = Gun("AK47",5) # 射击敌人 gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() gun.shot() ''' 输出: 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:4 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:3 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:2 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:1 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:0 *************射击失败,子弹数为0.已经为您子弹加弹5发!请重新射击!************** 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:4 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:3 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:2 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:1 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:0 *************射击失败,子弹数为0.已经为您子弹加弹5发!请重新射击!************** 此枪型号是:AK47,此次射击成功!子弹减少1发,余量为:4 '''
8. How to create private attributes, in The outside cannot be accessed or modified
#1 Specifies a private attribute that cannot be changed externally: that is, add two underscores in front of the attribute, such as __money
#2 In python, there is only a certain Properties with two underscores in front of them are called private properties, while __money__ is not called a special property.
#3 _money is not a private attribute, but when we see such a variable, we should treat it as private by convention. It is not private in nature, it is accessible.
# 指定私有属性,在外部无法更改:即在属性前加两个下划线 如__money class Person(object): def __init__(self,name,age,height,weight,money): self.name = name self.age = age self.height = height self.weight = weight self.__money= money def run(self): print("跑!") def eat(self): print('吃') def changeName(self,name): self.name = name # 创建对象函数 self.__class__ 是代表类名的 def createObj(self,name): p=self.__class__(name,24,56,89,10000) print(p.name,p.age,p.weight,p.height,p.__money) # 即 执行p1的一个方法即可创建新的对象。 p1 = Person("丽丽",33,53,222,20000) print(p1.name,p1.__money) #此处出错,在外部无法访问私有
If you want to access it from outside, you can put the private properties in the method and call the method to access the private properties. Such as:
def run(self): print("跑!") print("工资",self.__money)
Summary: When accessing or modifying private attributes, modification and access can be achieved through two fixed methods. At the same time, data can be filtered in the methods.
# 对于处理私有属性的方法---设置值的方法 def SetMoney(self,money): if money<0: print("输入的钱不能小于0") else: self.__money = money # 对于访问私有属性的方法 def GetMoney(self): return self.__money
# In fact, the reason why __money cannot be accessed is because python changed the private attribute to _Person__money,
# that is, it is still accessible. But you should follow the rules and not access it like this.
p1._Person__money = 70 print(p1.GetMoney())
As mentioned above, you can still modify the private attributes.
父类的文件信息: class People(object): def __init__(self,name,age): self.name=name self.age=age 子类中调用上边的父类 from people import People class Student(People): def __init__(self,name,age,faceValue): # 继承父类的__init__() super(Student,self).__init__(name,age) self.faceValue = faceValue stu = Student("玲",22,100) print(stu.name,stu.age,stu.faceValue) 输出 玲 22 100 # 总结: # 我们发现Student类中并无这两个属性信息,这两个属性是调用的父类的__init__中的属性。 # 总结: # 我们发现Student类中并无这两个属性信息,这两个属性是调用的父类的__init__中的属性。
The above is the detailed content of A brief discussion on object-oriented programming in python (code examples). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


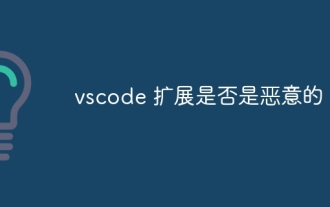
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
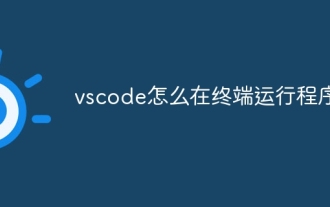
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
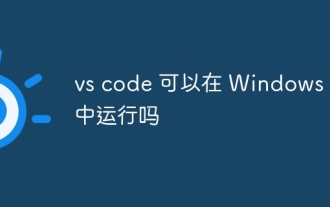
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
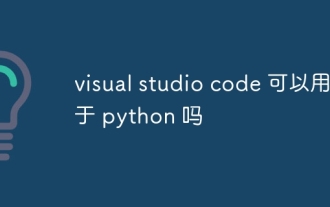
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
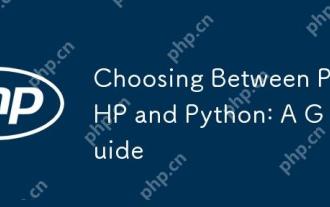
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
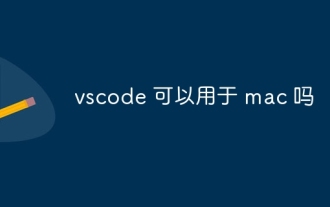
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
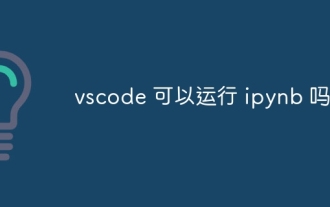
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
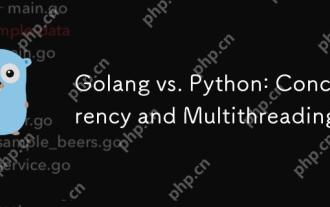
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
