Detailed explanation of usage of position: sticky
Position: sticky in CSS allows us to build some very concise interactions in very few lines of code. It's useful anytime the user wants a UI element to stay in view while scrolling, but not become sticky until the element reaches a specific distance from the top/bottom/left/right side of the scroll viewport.
How can we set a position: sticky?
position: sticky is perfect for iOS style list headers. Scroll the content and watch the title after it hits 0px from the top edge.
.heading{ background: #ccc; height: 50px; line-height: 50px; margin-top: 10px; font-size: 30px; padding-left: 10px; position: -webkit-sticky; position: sticky; top: 0px; }
You want the list title to remain visible if the user scrolls down. If you scroll up, you also want the "Add Item" footer to be visible. Try it! Scroll up and down and watch how the elements stick after reaching the edge of the viewport:
header{ background: #ccc; font-size: 20px; color: #282a37; padding: 10px; position: -webkit-sticky; position: sticky; top: 0; } footer{ background: #ccc; padding: 10px; color: #ae81fe; position: relative; position: -webkit-sticky; position: sticky; } footer{ bottom: 0; } list{ border: 1px solid #ccc; border-radius: 5px; width: 200px; margin-left: 20px; background: #282a37; } item{ padding: 10px; color: #fff; display: block; } body{ padding-top: 20px; display: flex; align-items: flex-start; } .abs{ position: absolute; right: 0; top: 10px; }
You can also stick items to the left or right edge. This is a side image viewer with a rotated text description of the image. Scroll it sideways and watch as the description is docked to the left, in view, until a new description pushes it out of the way.
div[description]{ max-width: 40px; height: 300px; position: -webkit-sticky; position: sticky; left: 0; /* become sticky once touching left edge */ } sidescroller{ display: flex; align-items: center; overflow-x: auto; overflow-y: hidden; background: #000; } div[wrapper]{ flex: 0 0 40px; max-width: 40px; height: 300px; position: -webkit-sticky; position: sticky; left: 0; white-space: nowrap; color: #fff; } div[item]{ display: flex; } div[description] span{ display: inline-block; background: rgba(0,0,0,.5); width: 300px; height: 40px; transform: rotate(-90deg) translateX(-300px); transform-origin: left top 0; padding-top: 11px; text-align: center; text-transform: uppercase; color: #fff; font-size: 14px; } img{ max-height: 300px; }
You can even specify a negative number when an element becomes sticky when some or all of it scrolls out of view! This might be useful, for example, if the sidebar menu becomes sticky when scrolled out of view, leaving a small button that when clicked can jump back to the sidebar:
.sidebar{ background: purple; width: 200px; height: 300px; padding: 20px; flex-shrink: 0; overflow: visible; position: -webkit-sticky; position: sticky; left: -200px; } .sidebar .handle{ height: 30px; width: 30px; position: absolute; right: -30px; top: 0; background: purple; color: #fff; font-weight: bold; font-size: 20px; padding-left: 8px; cursor: pointer; } p{ padding: 20px; } .site{ display: flex; }
Use position: sticky is very simple.
1. Declare the element as sticky position:sticky (plus any required browser prefix position: -webkit-sticky)
2. Specify the edge of the item to be "sticked" (Top|Right|Bottom|Left).
3. Enter the distance from the edge, and the stickiness will be activated when it is reached.
For example, say you want the title to become sticky when it is 20px from the top of the scroll area:
.header{ position: -webkit-sticky; position: sticky; top: 20px; }
Or scroll to the left edge of the menu out of view like the example above:
.menu{ width: 200px; position: -webkit-sticky; position: sticky; left: -200px; }
Notes on position: sticky
Sibling elements
If sibling (adjacent) elements are set to position: sticky, they behaves slightly differently than elements in nested items. Sticky sibling elements are not moved for new elements. Instead, they overlap in place:
Sometimes you may want this behavior, but if you do set a background color, otherwise the user will see all Projects are packed into the same small space all at once and it looks like a mess.
On the other hand, if you nest a sticky element into a parent element, like we did in the SsiDelLoad example, then the sticky element will start to move as soon as another sticky element starts touching it , which is a good practice and works a bit like IMO:
Overflow (overflow)
Don’t use overflow: auto |scroll|hidden on the parent element of a position:sticky element, which completely breaks stickiness, but overflow: visible does.
Absolute positioning (position:absolute)
If you want to use position:absolute elements inside a sticky element, you must be careful. If your application is running in an older browser that does not support position:sticky, the sticky element will not behave like a relatively positioned element. So an absolutely positioned element will skip it and look up the DOM tree until it finds the next non-static element (absolute|relative fixed position), html will default to the element if not found. In other words, your absolutely positioned element will look different on screen than you expect. If you are building on some older browsers, one might think that the solution to this situation is to set both relative and sticky positioning:
.footerwithAbsolutePositionedeChildren{ position: relative;/*don't forgot this*/ } /*NOTE: @supports has to be at the root,not nested*/ @supports(position:sticky){ .footerwithAbsolutePositionedeChildren{ position: sticky; bottom: 20px;/now this won't mess with position in non-sticky browsers*/ } }
Why not use JavaScript?
You can definitely achieve this using JS. But this will involve a scroll event listener, which is still a very expensive thing to add to your application. Scrolling is one of the most common actions performed by users, and executing JavaScript during these events makes it difficult to maintain stable 60 FPS (frames per second) scrolling. The UI is out of sync with the user's mouse/finger/stylus. This is called rolling jank. There is a special kind of event listener called a passive event listener, which lets the browser know that your events will not stop scrolling, so the browser can better optimize these events. But they're not supported yet in IE or Edge, you'll need the JS fallback anyway.
Additionally, since position:sticky you are not writing to the DOM during scrolling, you are not causing any forced layout and layout recalculation. So the browser is able to move this operation to the GPU and you can scroll very smoothly even when the sticky element is playing. It's especially smooth in mobile Sarari.
Also, writing two lines of declarative CSS is easier than using the JS alternative.
Can I use it now?
position:sticky is supported by many browsers, but not yet in Edge. IE doesn't matter here unless you have contractual obligations in Enterprise Town. If you must have this behavior, there are a lot of polyfills out there, but they all use JavaScript, so you'll get the performance mentioned above. A better option is to design your app so that sticky locations are a slick addition, but the app still doesn't have its functionality.
The above is the detailed content of Detailed explanation of usage of position: sticky. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


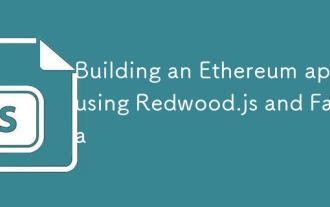
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
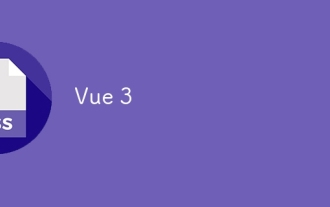
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
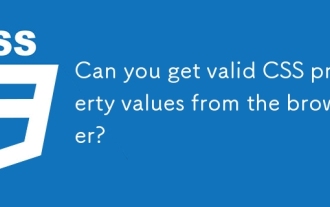
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
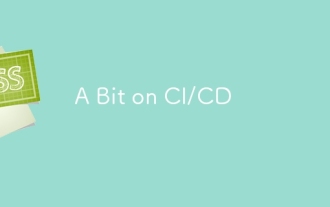
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
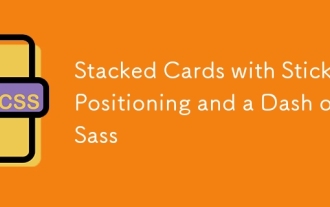
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
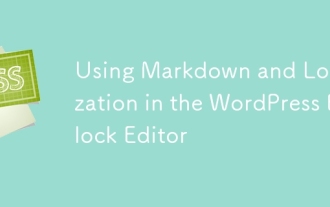
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
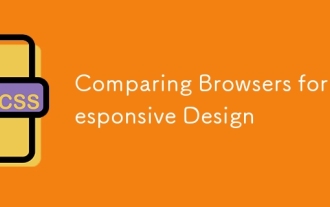
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
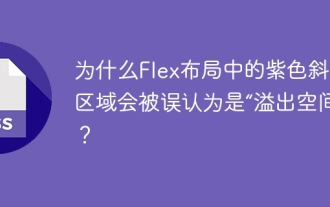
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
