Introduction to the typing module in Python (code example)
Python is a weakly typed language. Many times we may not know the function parameter type or return value type, which may lead to some types not specifying methods. The typing module can solve this problem very well.
The addition of this module will not affect the running of the program, and no formal errors will be reported, only reminders.The typing module can only be used in python3.5 or above. Pycharm currently supports typing check
1. The role of the typing module
1. Type Check to prevent parameter and return value type inconsistencies from occurring during runtime.
2. As an attachment to the development document, it is convenient for users to pass in and return parameter types when calling.
2. Commonly used methods of typing module
Look at the example code first:
from typing import List,Tuple,Dict def add(a:int,string:str,f:float,b:bool)->Tuple[List,Tuple,Dict,bool]: list1=list(range(a)) tup=(string,string,string) d={"a":f} bl=b return list1,tup,d,bl if __name__ == '__main__': print(add(5,'mark',183.1,False))
Run result:
([0, 1, 2, 3, 4], ('mark', 'mark', 'mark'), {'a': 183.1}, False)
Explanation:
When passing in parameters, declare the type of the parameter in the form of "parameter name: type";
The return result is passed in "-> Declare the result type in the form of "result type"
. If the parameter type is incorrect when calling, pycharm will remind you, but it will not affect the running of the program.
For lists such as lists, you can also specify something more specific, such as "->List[str]", which specifies that a list is returned and the elements are strings.
Now modify the above code, you can see that the pycharm background turns yellow, which is the error type reminder:
3. Commonly used types of typing
int, long, float: integer, long integer, floating point type
bool,str: Boolean type, string type
List, Tuple, Dict, Set: list, tuple, dictionary, set
Iterable, Iterator: Iterator, iterator type
Generator: Generator type
four , typing supports possible multiple types
Since Python inherently supports polymorphism, there may be multiple elements in the iterator.
Code example:
from typing import List, Tuple, Dict def add(a: int, string: str, f: float, b: bool or str) -> Tuple[List, Tuple, Dict, str or bool]: list1 = list(range(a)) tup = (string, string, string) d = {"a": f} bl = b return list1, tup, d, bl if __name__ == '__main__': print(add(5, 'mark', 183.1, False)) print(add(5, 'mark', 183.1, 'False'))
Running result (no different from not using typing):
([0, 1, 2, 3, 4], ('mark', 'mark', 'mark'), {'a': 183.1}, False) ([0, 1, 2, 3, 4], ('mark', 'mark', 'mark'), {'a': 183.1}, 'False')
The above is the detailed content of Introduction to the typing module in Python (code example). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


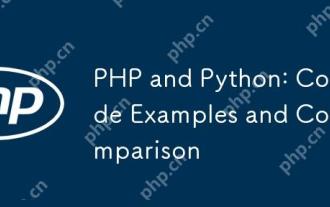
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
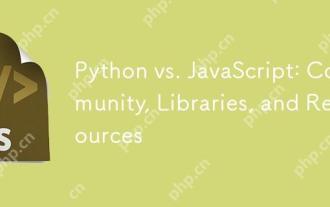
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
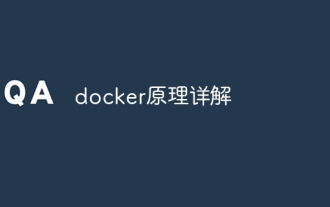
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
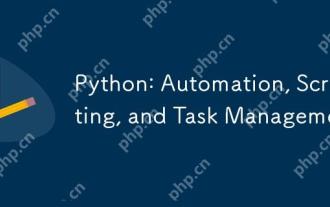
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
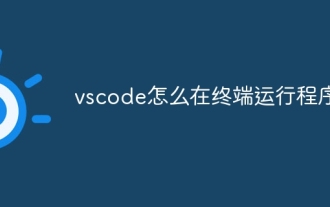
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
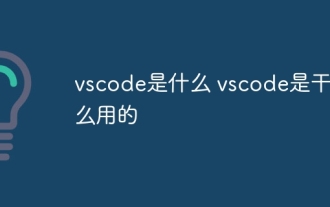
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
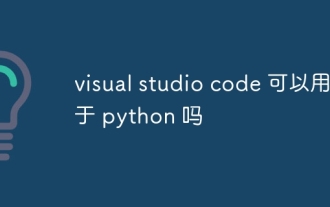
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
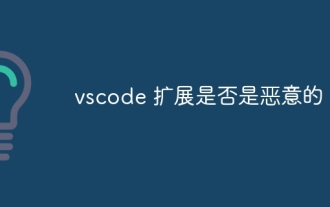
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
