


Problems and solutions encountered when using props to assign initial values to data in Vue
The content of this article is about the problems and solutions encountered when using props to assign initial values to data in Vue. It has certain reference value. Friends in need can refer to it. I hope it will be useful to you. helped.
I was working on a project for operational activities some time ago. After it was launched, the product feedback page had something wrong. During the investigation, I found that the problem was caused by the initial value of data in Vue, and the initial value of data Comes from props. For the convenience of description, the problem is abstracted as follows:
1. Phenomenon
Code:
nbsp;html> <meta> <title>用props初始化data中变量</title> <script></script> <div> <user-info></user-info> </div> <script> //全局组件 let userInfo = Vue.component('userInfo' ,{ name: 'user-info', props: { userData: Object }, data() { return { userName: this.userData.name } }, template: ` <div> <div>姓名:{{userName}} <div>性别:{{userData.gender}} <div>生日:{{userData.birthday}} ` }); //Vue实例 new Vue({ el: '#app', data: { user: { name: '', gender: '', birthday: '' } }, created(){ this.getUserData(); }, methods:{ getUserData(){ setTimeout(()=>{ this.user = { name: '于永雨', gender: '男', birthday: '1991-7' } }, 500) } }, components: { userInfo } }); </script>
Code interpretation:
There is an object in the root component data: user, which contains three attributes: name, gender, and birthday. The initial values are all empty strings.
Simulate asynchronous API requests. After 500 milliseconds, the user is reassigned, and the three attributes are no longer empty.
Declare a subcomponent userInfo, and there is an object userData in the props, which is used to receive the user of the parent component; There is a variable userName in data, the initial value comes from userData.name
Result:
page After initialization, the name, gender, and birthday are all displayed as empty. After 500 milliseconds, the gender and birthday display normal results, and only the name does not change.
Why is this so?
My initial idea: user.name is String, which belongs to the basic data type. Use it to assign values to userName in the child component data. It belongs to the basic data type assignment, so when user.name in the parent component changes , the userName in the subcomponent will not change accordingly.
Is that so? So I decided to change user.name to an object, assign the value by reference to the data type, and then observe whether it meets expectations. The code is as follows:
nbsp;html> <meta> <title>用props初始化data中变量-对象形式</title> <script></script> <div> <user-info></user-info> </div> <script> //全局组件 let userInfo = Vue.component('userInfo' ,{ name: 'user-info', props: { userData: Object }, data() { return { userName: this.userData.name } }, template: ` <div> <div>姓名:{{userName.text}} <div>性别:{{userData.gender}} <div>生日:{{userData.birthday}} ` }); //Vue实例 new Vue({ el: '#app', data: { user: { name: {text: ''}, gender: '', birthday: '' } }, created(){ this.getUserData(); }, methods:{ getUserData(){ setTimeout(()=>{ this.user = { name: {text: '于永雨'}, gender: '男', birthday: '1991-7' } }, 500) } }, components: { userInfo } }); </script>
Running result: The name still has no value, the same as the first result! ! !
2. Reason
So, what is the reason? I was puzzled. Later, when discussing with my friends, someone asked: Could it be because the data is deeply copied during initialization?
I think this explanation is more reliable, so I went to collect evidence. First, I went to the Vue official website to read the documents about data, among which:
When you see the word "recursively", you can basically conclude that the above inference is correct, because the core principle of deep copy is recursion.
It turns out that Vue will recursively traverse all properties of data during initialization, and use Object.defineProperty to convert all these properties into getters/setters for two-way binding. The official document clearly states in the Reactivity in Depth chapter:
also explains why Vue does not support IE8: IE8 Object.defineProperty is not supported.
3. Solution
Since data cannot be updated as props change due to deep copy of data, we naturally think of two monitoring functions in Vue. Functions: watch, computed.
Modify the code as follows and observe the results:
nbsp;html> <meta> <title>解决方案:watch、computed</title> <script></script> <div> <user-info></user-info> </div> <script> //全局组件 let userInfo = Vue.component('userInfo' ,{ name: 'user-info', props: { userData: Object }, data() { return { userName: this.userData.name } }, computed: { computedUserName(){ return this.userData.name } }, watch: { 'userData.name': function (val) {//监听props中的属性 this.userName = val; } }, template: ` <div> <div>姓名(watch):{{ userName }} <div>姓名(computed):{{ computedUserName }} <div>性别:{{ userData.gender }} <div>生日:{{ userData.birthday }} ` }); //Vue实例 new Vue({ el: '#app', data: { user: { name: '', gender: '', birthday: '' } }, created(){ this.getUserData(); }, methods:{ getUserData(){ setTimeout(()=>{ this.user = { name: '于永雨', gender: '男', birthday: '1991-7' } }, 500) } }, components: { userInfo } }); </script>
Running results
##Perfect ! ! !
4. Summary: Key points about props in VueAfterwards, I carefully read the documentation about props:Disadvantages: local variables do not follow Update
as props are updated (2) After converting the props value in computed, outputThe above is the detailed content of Problems and solutions encountered when using props to assign initial values to data in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


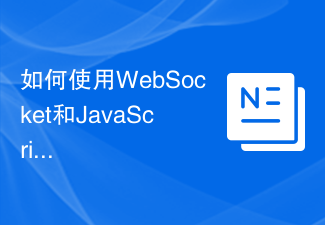
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
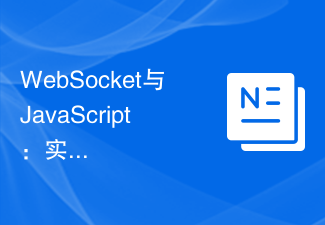
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
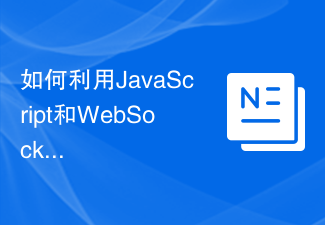
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
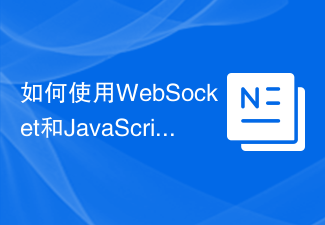
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
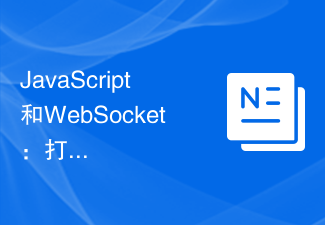
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
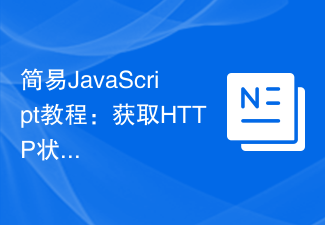
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
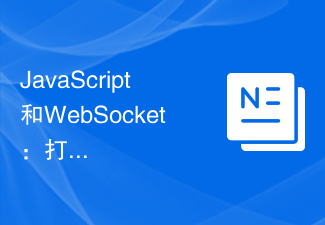
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
