Detailed explanation of Laravel's method of handling session (session)
In web applications, it is necessary to identify users across requests and save data for each user, and for this, frameworks like Laravel provide a mechanism called sessions. This article will introduce to you how Laravel handles sessions.
Sessions can store data (keys and values), Laravel provides various backend sessions, which can be set in config/session.php.
File sessions by default save sessions in files in the storage/framework/sessions/ directory. In a production environment we will consider using database sessions, redis sessions, etc., but in a development environment it is enough to use the default file session.
php Chinese website learning topic: php session (including pictures, texts, videos, cases)
How to use sessions in Laravel
There are two main ways to operate sessions with Laravel.
One is through the Request instance passed to the operation.
Use Illuminate\Session\Store instance.
// 从会话中获取指定的数据 //在没有存在键的情况下,将返回的默认值指定为第二参数 $value = $request->session()->get('key’); $value = $request->session()->get('key', 'default’); $value = $request->session()->get('key', function () { return 'default'; }); // 获取会话中的所有数据 $data = $request->session()->all(); // 检查指定的数据是否存在于会话中 if ($request->session()->exists('key')) { // 存在 } if ($request->session()->has('key')) { // null不存在 } // 将数据保存到会话 $request->session()->put('key', 'value'); $request->session()->put(['key1' => 'value1', 'key2' => ‘value2']); // 从会话取得指定的数据后,删除该数据 $value = $request->session()->pull('key', 'default’); // 从会话中删除指定的数据 $request->session()->forget('key'); // 从会话中删除所有数据 $request->session()->flush();
Another way is to use the global helper function session().
Use Illuminate\Session\SessionManager instance.
// 从会话中获取指定的数据 // 在没有存在键的情况下,将返回的默认值指定为第二参数 $value = session('key’); $value = session('key', 'default'); $value = session('key', function () { return 'default'; }); $value = session()->get(‘key'); $value = session()->get('key', 'default'); $value = session()->get('key', function () { return 'default'; }); // 取得会话中的全部数据 $data = session()->all(); // 检查指定的数据是否存在于会话中 if (session()->exists('key')) { // 存在 } if (session()->has('key')) { // null不存在 } // 保存数据到会话 session(['key1' => 'value1', 'key2' => ‘value2']); session()->put(['key1' => 'value1', 'key2' => 'value2']); // 从会话取得指定的数据后,删除该数据 $value = session()->pull('key', 'default’); // 从会话中删除指定的数据 session()->forget('key'); // 从会话中删除所有数据 session()->flush();
Let’s look at A specific example of using session in Laravel
A very simple example
We define the following route.
routes/web.php Route::get('/put-data', function () { session()->put(['email' => 'user@example.com']); return session()->get('email'); }); Route::get(‘/list-data', function () { return session()->all(); });
First, when accessing /put-data in the browser, the first path is executed and you can confirm that the data has been saved in the session.
user@example.com
Next, when accessing /list-data in the browser, the second path will be executed, and you can confirm whether the previously saved data has been retained
{"email":"user@example.com","_previous":{"url":"http:\/\/localhost:8000\/put-data"},"_flash":{"old":[],"new":[]},"_token":"UYcsteOQAj58e9Aay5uNc3V4F0fSpi9VfEBlKhTZ"}
Of course there are other data, But these are automatically saved data, Laravel itself also uses sessions.
The above is the detailed content of Detailed explanation of Laravel's method of handling session (session). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
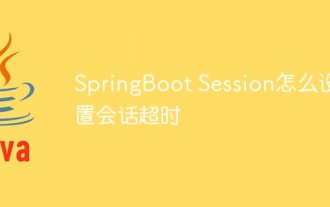
The problem was found in the springboot project production session-out timeout. The problem is described below: In the test environment, the session-out was configured by changing the application.yaml. After setting different times to verify that the session-out configuration took effect, the expiration time was directly set to 8 hours for release. Arrived in production environment. However, I received feedback from customers at noon that the project expiration time was set to be short. If no operation is performed for half an hour, the session will expire and require repeated logins. Solve the problem of handling the development environment: the springboot project has built-in Tomcat, so the session-out configured in application.yaml in the project is effective. Production environment: Production environment release is
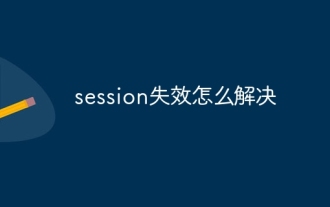
Session failure is usually caused by the session lifetime expiration or server shutdown. The solutions: 1. Extend the lifetime of the session; 2. Use persistent storage; 3. Use cookies; 4. Update the session asynchronously; 5. Use session management middleware.
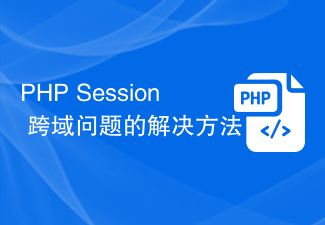
Solution to the cross-domain problem of PHPSession In the development of front-end and back-end separation, cross-domain requests have become the norm. When dealing with cross-domain issues, we usually involve the use and management of sessions. However, due to browser origin policy restrictions, sessions cannot be shared by default across domains. In order to solve this problem, we need to use some techniques and methods to achieve cross-domain sharing of sessions. 1. The most common use of cookies to share sessions across domains
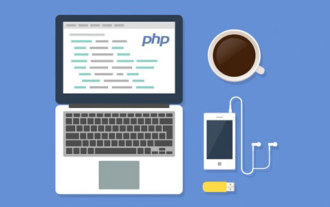
Solution to the problem that the php session disappears after refreshing: 1. Open the session through "session_start();"; 2. Write all public configurations in a php file; 3. The variable name cannot be the same as the array subscript; 4. In Just check the storage path of the session data in phpinfo and check whether the sessio in the file directory is saved successfully.
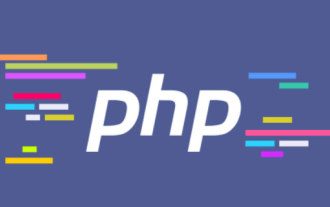
The default expiration time of session PHP is 1440 seconds, which is 24 minutes, which means that if the client does not refresh for more than 24 minutes, the current session will expire; if the user closes the browser, the session will end and the Session will no longer exist.
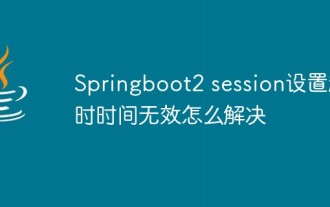
Problem: Today, we encountered a setting timeout problem in our project, and changes to SpringBoot2’s application.properties never took effect. Solution: The server.* properties are used to control the embedded container used by SpringBoot. SpringBoot will create an instance of the servlet container using one of the ServletWebServerFactory instances. These classes use server.* properties to configure the controlled servlet container (tomcat, jetty, etc.). When the application is deployed as a war file to a Tomcat instance, the server.* properties do not apply. They do not apply,

When you are using a PHP session (Session), sometimes you will find that the Session can be read normally in one file, but cannot be read in another file. This may confuse you since session data is supposed to be shared across the entire application. This article will explain how to correctly read and write PHP session data in multiple files.
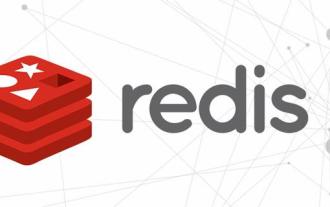
1. Implementing SMS login based on session 1.1 SMS login flow chart 1.2 Implementing sending SMS verification code Front-end request description: Description of request method POST request path /user/code request parameter phone (phone number) return value No back-end interface implementation: @Slf4j@ ServicepublicclassUserServiceImplextendsServiceImplimplementsIUserService{@OverridepublicResultsendCode(Stringphone,HttpSessionsession){//1. Verify mobile phone number if
