Introduction to the definition and use of Java annotations (code examples)
This article brings you the definition and use of Java annotations (code examples). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Java annotations are used a lot in actual projects, especially after using Spring.
This article will introduce the syntax of Java annotations and examples of using annotations in Spring.Syntax of annotations
Examples of annotations
Take the @Test annotation in Junit as an example
@Target(ElementType.METHOD) @Retention(RetentionPolicy.RUNTIME) public @interface Test { long timeout() default 0L; }
You can see the @Test
annotation There are two annotations @Target()
and @Retention()
.
This kind of annotation that annotates annotations is called meta-annotation.
It has the same meaning as the data that declares the data, which is called metadata. The format of the annotation after
is
修饰符 @interface 注解名 { 注解元素的声明1 注解元素的声明2 }
The element of the annotation has two forms
type elementName(); type elementName() default value; // 带默认值
Common meta-annotations
@Target
Annotation
@Target
annotation is used to limit which items the annotation can be applied to. Annotations without @Target
can be applied to on any item.
You can see all items accepted by @Target
- # in the
- java.lang.annotation.ElementType
class ##TYPE
Use
#FIELD
on [Class, Interface, Annotation] Use
## on [Field, Enumeration Constant]- #METHOD
Use
## on [Method] Use - ## on [Parameter]
Use on [Constructor]#CONSTRUCTOR
-
##LOCAL_VARIABLE
Use on [Local Variable] ANNOTATION_TYPE
Useon [Annotation] Use
on [Package]TYPE_PARAMETER
Introduced in Java 1.8 on [type parameters]
- ##TYPE_USE
on [any declared type Where] using Java 1.8, the
- #@Test
annotation is only allowed to be used on methods.
<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">@Target(ElementType.METHOD) public @interface Test { ... }</pre><div class="contentsignin">Copy after login</div></div>
If you want to support multiple items, pass in multiple values.@Target({ElementType.TYPE, ElementType.METHOD}) public @interface MyAnnotation { ... }
Copy after loginIn addition, meta-annotations are also annotations and conform to the syntax of annotations, such as
annotations. @Target(ElementType.ANNOTATION_TYPE)
@Target
annotation can only be used on annotations.@Documented @Retention(RetentionPolicy.RUNTIME) @Target(ElementType.ANNOTATION_TYPE) public @interface Target { ElementType[] value(); }
@RetentionAnnotation
@Retention
Specifies how long the annotation should be retained. The default is RetentionPolicy.CLASS. You can see all items in
java.lang.annotation.RetentionPolicy
SOURCE
is not included in the class file
- CLASS
Contained in the class file, the virtual machine is not loaded
- RUNTIME
Contained in the class file, loaded by the virtual machine, you can use the reflection API to get the
- @Test
annotation will be loaded into the virtual machine, you can get it through the code
@Documented<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">@Retention(RetentionPolicy.RUNTIME) public @interface Test { ... }</pre><div class="contentsignin">Copy after login</div></div>
Annotation
is mainly used for archiving tool identification. Annotated elements can be documented using Javadoc
or similar tools.
@Inherited
Annotation
Added the annotation of the @Inherited
annotation, the subclasses of the annotated class will also have this annotation
Annotation<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
@Inherited
public @interface MyAnnotation { ... }</pre><div class="contentsignin">Copy after login</div></div>
Parent class
@MyAnnotation class Parent { ... }
SubclassChild
will add
to
ParentInherited
class Child extends Parent { ... }
@Repeatable
AnnotationsThe annotations introduced in Java 1.8 mark the annotations as reusable.
Note 1
public @interface MyAnnotations { MyAnnotation[] value(); }
Note 2<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">@Repeatable(MyAnnotations.class)
public @interface MyAnnotation {
int value();
}</pre><div class="contentsignin">Copy after login</div></div>
Used when using
@Repeatable()
@MyAnnotation(1) @MyAnnotation(2) @MyAnnotation(3) public class MyTest { ... }
Not used## When using #@Repeatable()
,@MyAnnotation
remove@Repeatablemeta-annotation
@MyAnnotations({ @MyAnnotation(1), @MyAnnotation(2), @MyAnnotation(3)}) public class MyTest { ... }
@ComponentScan annotation of
Spring
also uses this meta-annotation. Types of elements
Supported element types8 basic data types (
byte
short
,char
,- int
- ,
long
##Class,
float,
double,
boolean)
String
-
enum Annotation type
Array (all arrays of the above types)
Example-
Enumeration class
public enum Status { GOOD, BAD }
Copy after loginNote 1 -
@Target(ElementType.ANNOTATION_TYPE) public @interface MyAnnotation1 { int val(); }
Copy after loginNote 2
@Target(ElementType.TYPE) public @interface MyAnnotation2 { boolean boo() default false; Class<?> cla() default Void.class; Status enu() default Status.GOOD; MyAnnotation1 anno() default @MyAnnotation1(val = 1); String[] arr(); }
Copy after loginWhen used, elements without default values must pass values
@MyAnnotation2( cla = String.class, enu = Status.BAD, anno = @MyAnnotation1(val = 2), arr = {"a", "b"}) public class MyTest { ... }
Built-in annotations
@OverrideAnnotations
tells the compiler that this is a method that overrides the parent class. If the parent class deletes this method, the subclass will report an error.
@Deprecated
Annotation
indicates that the annotated element has been deprecated.
@SuppressWarningsAnnotation
Tells the compiler to ignore warnings.
@FunctionalInterfaceAnnotations
Annotations introduced in Java 1.8. This annotation forces the compiler javac
to check whether an interface conforms to the functional interface standard.
特别的注解
有两种比较特别的注解
标记注解 : 注解中没有任何元素,使用时直接是
@XxxAnnotation
, 不需要加括号单值注解 : 注解只有一个元素,且名字为
value
,使用时直接传值,不需要指定元素名@XxxAnnotation(100)
利用反射获取注解
Java
的AnnotatedElement
接口中有getAnnotation()
等获取注解的方法。
而Method
,Field
,Class
,Package
等类均实现了这个接口,因此均有获取注解的能力。
例子
注解
@Retention(RetentionPolicy.RUNTIME) @Target({ElementType.TYPE, ElementType.FIELD, ElementType.METHOD}) public @interface MyAnno { String value(); }
被注解的元素
@MyAnno("class") public class MyClass { @MyAnno("feild") private String str; @MyAnno("method") public void method() { } }
获取注解
public class Test { public static void main(String[] args) throws Exception { MyClass obj = new MyClass(); Class<?> clazz = obj.getClass(); // 获取对象上的注解 MyAnno anno = clazz.getAnnotation(MyAnno.class); System.out.println(anno.value()); // 获取属性上的注解 Field field = clazz.getDeclaredField("str"); anno = field.getAnnotation(MyAnno.class); System.out.println(anno.value()); // 获取方法上的注解 Method method = clazz.getMethod("method"); anno = method.getAnnotation(MyAnno.class); System.out.println(anno.value()); } }
在Spring
中使用自定义注解
注解本身不会有任何的作用,需要有其他代码或工具的支持才有用。
需求
设想现有这样的需求,程序需要接收不同的命令CMD
,
然后根据命令调用不同的处理类Handler
。
很容易就会想到用Map
来存储命令和处理类的映射关系。
由于项目可能是多个成员共同开发,不同成员实现各自负责的命令的处理逻辑。
因此希望开发成员只关注Handler
的实现,不需要主动去Map
中注册CMD
和Handler
的映射。
最终效果
最终希望看到效果是这样的
@CmdMapping(Cmd.LOGIN) public class LoginHandler implements ICmdHandler { @Override public void handle() { System.out.println("handle login request"); } } @CmdMapping(Cmd.LOGOUT) public class LogoutHandler implements ICmdHandler { @Override public void handle() { System.out.println("handle logout request"); } }
开发人员增加自己的Handler
,只需要创建新的类并注上@CmdMapping(Cmd.Xxx)
即可。
具体做法
具体的实现是使用Spring
和一个自定义的注解
定义@CmdMapping
注解
@Documented @Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) @Component public @interface CmdMapping { int value(); }
@CmdMapping
中有一个int
类型的元素value
,用于指定CMD
。这里做成一个单值注解。
这里还加了Spring
的@Component
注解,因此注解了@CmdMapping
的类也会被Spring创建实例。
然后是CMD
接口,存储命令。
public interface Cmd { int REGISTER = 1; int LOGIN = 2; int LOGOUT = 3; }
之后是处理类接口,现实情况接口会复杂得多,这里简化了。
public interface ICmdHandler { void handle(); }
上边说过,注解本身是不起作用的,需要其他的支持。下边就是让注解生效的部分了。
使用时调用handle()
方法即可。
@Component public class HandlerDispatcherServlet implements InitializingBean, ApplicationContextAware { private ApplicationContext context; private Map<Integer, ICmdHandler> handlers = new HashMap<>(); public void handle(int cmd) { handlers.get(cmd).handle(); } public void afterPropertiesSet() { String[] beanNames = this.context.getBeanNamesForType(Object.class); for (String beanName : beanNames) { if (ScopedProxyUtils.isScopedTarget(beanName)) { continue; } Class<?> beanType = this.context.getType(beanName); if (beanType != null) { CmdMapping annotation = AnnotatedElementUtils.findMergedAnnotation( beanType, CmdMapping.class); if(annotation != null) { handlers.put(annotation.value(), (ICmdHandler) context.getBean(beanType)); } } } } public void setApplicationContext(ApplicationContext applicationContext) throws BeansException { this.context = applicationContext; } }
主要工作都是Spring
做,这里只是将实例化后的对象put
到Map
中。
测试代码
@ComponentScan("pers.custom.annotation") public class Main { public static void main(String[] args) { AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(Main.class); HandlerDispatcherServlet servlet = context.getBean(HandlerDispatcherServlet.class); servlet.handle(Cmd.REGISTER); servlet.handle(Cmd.LOGIN); servlet.handle(Cmd.LOGOUT); context.close(); } }
> 完整项目
总结
可以看到使用注解能够写出很灵活的代码,注解也特别适合做为使用框架的一种方式。
所以学会使用注解还是很有用的,毕竟这对于上手框架或实现自己的框架都是非常重要的知识。
The above is the detailed content of Introduction to the definition and use of Java annotations (code examples). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
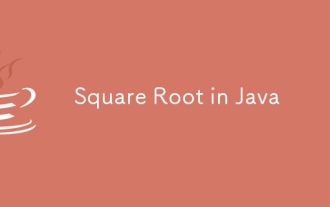
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
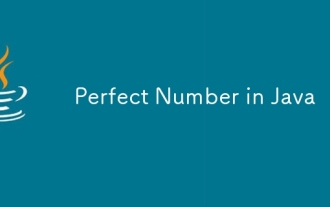
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
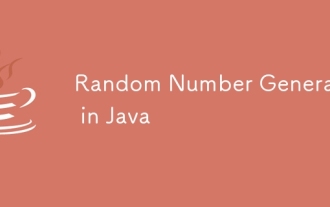
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
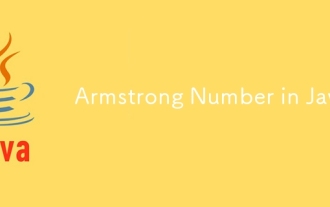
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
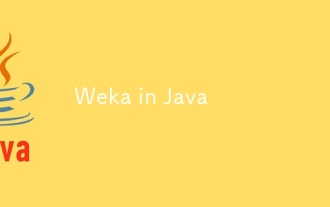
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
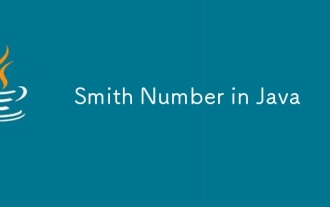
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
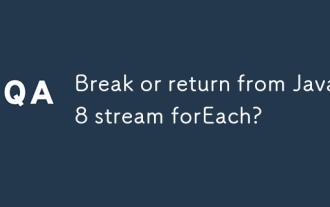
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
