How to merge elements of sublists in Python?
This article mainly introduces you to the implementation method of merging the elements of sublists in Python. For example, given two lists containing sublists, the task is to merge the elements of the sublists of the two lists in a single list. (Related recommendations: "Python Tutorial")
Below we will explain how to merge sublist elements in Python with specific code examples.
Example:
输入: list1 = [[1, 20, 30], [40, 29, 72], [119, 123, 115]] list2 = [[29, 57, 64, 22], [33, 66, 88, 15], [121, 100, 15, 117]] 输出: [[1, 20, 30, 29, 57, 64, 22], [40, 29, 72, 33, 66, 88, 15], [119, 123, 115, 121, 100, 15, 117]]
Method 1: Use Map lambda
# 初始化第一个列表 list1 = [[1, 20, 30], [40, 29, 72], [119, 123, 115]] # 初始化第二个列表 list2 = [[29, 57, 64, 22], [33, 66, 88, 15], [121, 100, 15, 117]] #使用map + lambda合并列表 Output = list(map(lambda x, y:x + y, list1, list2)) # 打印输出 print(Output)
Output:
[[1, 20, 30, 29, 57, 64, 22], [40, 29, 72, 33, 66, 88, 15], [119, 123, 115, 121, 100, 15, 117]]
Method 2: Use Zip()
list1 = [[1, 20, 30], [40, 29, 72], [119, 123, 115]] list2 = [[29, 57, 64, 22], [33, 66, 88, 15], [121, 100, 15, 117]] Output = [x + y for x, y in zip(list1, list2)] print(Output)
Output:
[[1, 20, 30, 29, 57, 64, 22], [40, 29, 72, 33, 66, 88, 15], [119, 123, 115, 121, 100, 15, 117]]
Method 3: Use starmap() and concat()
from operator import concat from itertools import starmap list1 = [[1, 20, 30], [40, 29, 72], [119, 123, 115]] list2 = [[29, 57, 64, 22], [33, 66, 88, 15], [121, 100, 15, 117]] Output = list(starmap(concat, zip(list1, list2))) print(Output)
Output:
[[1, 20, 30, 29, 57, 64, 22], [40, 29, 72, 33, 66, 88, 15], [119, 123, 115, 121, 100, 15, 117]]
This article is an introduction to the method of merging elements of sublists in Python. I hope it will be helpful to friends in need!
The above is the detailed content of How to merge elements of sublists in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


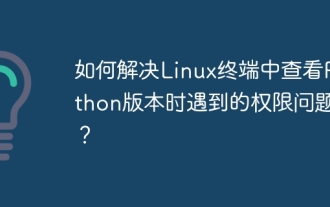
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
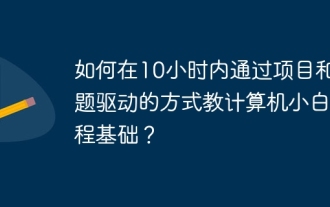
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
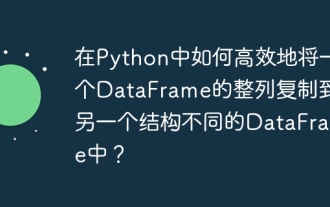
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
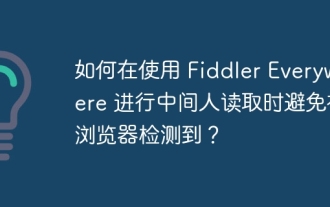
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
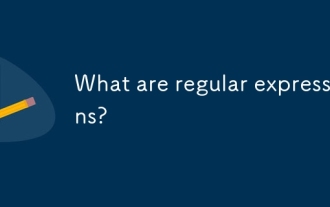
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
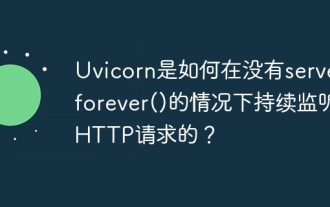
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
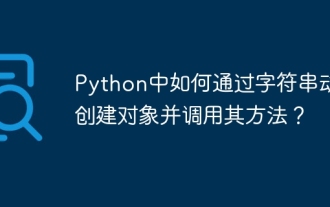
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
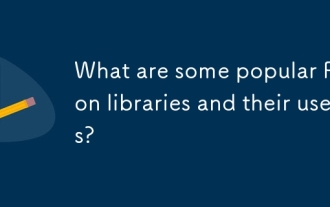
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
