[PHP] Principle and implementation code of heap sorting
The main content of this article is to use PHP to implement heap sorting, which has certain reference value. Interested friends can learn about it.
1. Heap (binary heap): It can be regarded as a complete binary tree. Except for the bottom layer, every level is full, which allows the heap to use arrays. To represent, each node corresponds to an element in the array
2. Given the subscript of a node, the subscript of the parent node and the child node can be calculated Standard; parent(i)=floor(i/2) left(i)=2i right=2i 1
3. Maximum heap and minimum heap, maximum heap: the root node is the maximum value, minimum heap: the root node is the minimum value
4. Heap sorting is to take out the maximum number at the top of the maximum heap, continue to adjust the remaining heap to the maximum heap, and take out the maximum number at the top of the heap again until the remaining Numbers have only one end
5. Maximum heap adjustment (maintain the maximum heap, the child node is always smaller than the parent node); create a maximum heap (adjust an array into an array of the maximum heap); Heap sort (create maximum heap, exchange, maintain maximum heap)
maxHeapify (array,index,heapSize) //最大堆调整 iMax,iLeft,iRight while true iMax=index;iLeft=2*index+1;iRight=2*index+2 如果根结点小于左右子树里结点值,就交换一下这两个值 利用第三方变量,交换下两个值 buildMaxHeap(array) //创建最大堆,把一个数组调整成最大堆的数组 iParent=floor((size-1)/2) for i=iParent;i>=0;i-- maxHeapify (array,i,size) sort(arr) buildMaxHeap(array, heapSize);//创建最大堆 for (int i = heapSize - 1; i > 0; i--) { swap(array, 0, i); //交换第一个和最后一个 maxHeapify(array, 0, i);//维护最大堆,size小了一个
//交换元素 function swap(&$arr,$a,$b){ $temp=$arr[$a]; $arr[$a]=$arr[$b]; $arr[$b]=$temp; } //排序的入口函数 function heapSort(&$arr){ $heapSize=count($arr); buildMaxHeap($arr, $heapSize);//创建最大堆 for ($i = $heapSize - 1; $i > 0; $i--) { swap($arr,0,$i); //交换第一个和最后一个 maxHeapify($arr, 0, $i);//维护最大堆,size小了一个 } } //创建最大堆的函数 function buildMaxHeap(&$arr, $heapSize){ $iParent=floor(($heapSize-1)/2);//根据最后一个元素的索引值计算该结点根结点的索引是哪个 for($i=$iParent;$i>=0;$i--){//这个循环是循环的所有根结点 maxHeapify($arr,$i,$heapSize);//维护最大堆 } } //维护最大堆 function maxHeapify(&$arr,$index,$heapSize){ $iMax=0;$iLeft=0;$iRight=0; while(true){ $iMax=$index; $iLeft=2*$iMax+1; $iRight=2*$iMax+2; if($iLeft<$heapSize && $arr[$iLeft]>$arr[$iMax]){ $iMax=$iLeft; } if($iRight<$heapSize && $arr[$iRight]>$arr[$iMax]){ $iMax=$iRight; } if($iMax!=$index){ swap($arr,$index,$iMax); $index=$iMax; }else{ break; } } } $arr=array(2,1,3,5,9,6); heapSort($arr); var_dump($arr);
Related tutorials: PHP video tutorial
The above is the detailed content of [PHP] Principle and implementation code of heap sorting. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


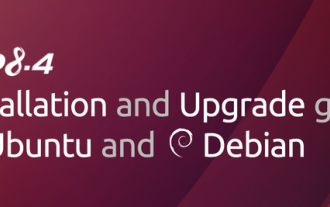
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
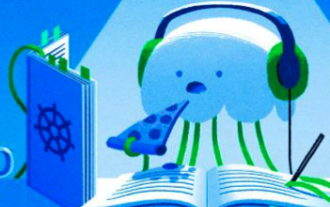
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
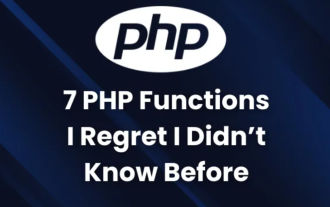
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
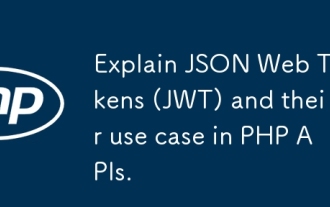
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
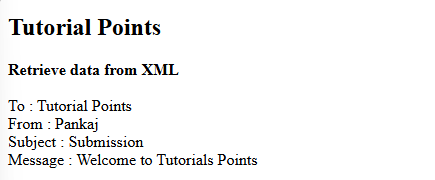
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
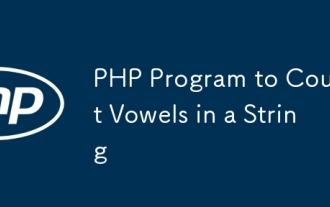
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
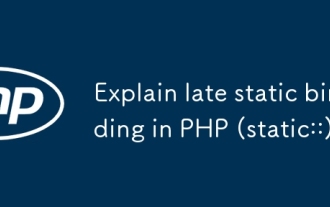
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
