Why can't static variables be serialized?
Static members belong to the class level, so they cannot be serialized. What cannot be serialized here means that the static member field is not included in the serialization information.
Here is an example that demonstrates why static variables cannot be serialized:
Class Student1
package test; import java.io.Serializable; public class Student1 implements Serializable{ private static final long serialVersionUID = 1L; private String name; private transient String password; private static int count = 0; public Student1(String name,String password){ System.out.println("调用Student的带参构造方法 "); this.name = name; this.password = password; count++; } public String toString(){ return "人数:" + count + "姓名:" + name + "密码:" + password; } }
Class ObjectSerTest1
package test; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class ObjectSerTest1 { public static void main(String args[]){ try{ FileOutputStream fos = new FileOutputStream("test.obj"); ObjectOutputStream oos = new ObjectOutputStream(fos); Student1 s1 = new Student1("张三","123456"); Student1 s2 = new Student1("王五","56"); oos.writeObject(s1); oos.writeObject(s2); oos.close(); FileInputStream fis = new FileInputStream("test.obj"); ObjectInputStream ois = new ObjectInputStream(fis); Student1 s3 = (Student1) ois.readObject(); Student1 s4 = (Student1) ois.readObject(); System.out.println(s3); System.out.println(s4); ois.close(); } catch (IOException e) { e.printStackTrace(); } catch (ClassNotFoundException e1) { e1.printStackTrace(); } } }
Run result:
Call Student’s constructor with parameters
Call Student’s constructor with parameters
Number of people: 2 Name: Zhang San’s password: null
Number of people: 2 Name: Wang Wu Password: null
Class Test1
package test; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class Test1{ public static void main(String args[]){ try { FileInputStream fis = new FileInputStream("test.obj"); ObjectInputStream ois = new ObjectInputStream(fis); Student1 s3 = (Student1) ois.readObject(); Student1 s4 = (Student1) ois.readObject(); System.out.println(s3); System.out.println(s4); ois.close(); } catch (IOException e) { e.printStackTrace(); } catch (ClassNotFoundException e1) { e1.printStackTrace(); } } }
Run result:
Number of people: 0 Name: Zhang San Password: null
Number of people: 0 Name: Wang Wu Password: null
Summary:
Class ObjectSerTest1 The running result shows that count=2, which seems to be serialized, but the running result of class Test1 shows that count=0 has not been serialized.
"Serialization saves the state of the object, and static variables are the state of the class, so serialization does not save static variables.
The meaning of "cannot be serialized" here is serialization The information does not include this static member field
ObjectSerTest1. The test is successful because they are all on the same machine (and the same process), because the jvm has already loaded count, so what you get is the loaded count, if you transfer it to another machine or you close the program and rewrite it and write a program to read test.obj, at this time, because other machines or new processes reload the count, the count information is the initial value. Information. "----- From the reference webpage
Rewrite class Test1 reads test.obj and the result displayed is the initial information of count, which also verifies the above paragraph.
Finally, static and transient modified properties of Java objects cannot be serialized.
The above is the detailed content of Why can't static variables be serialized?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
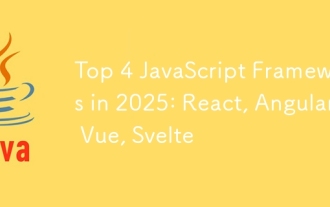
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
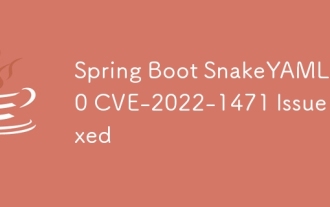
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
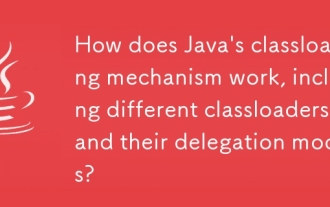
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
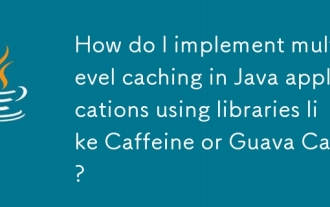
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
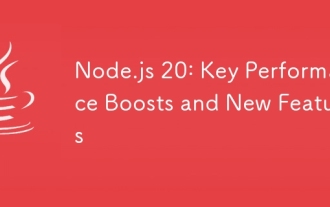
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
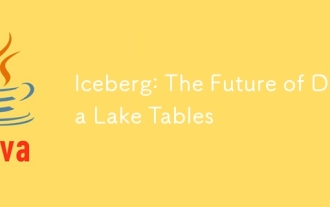
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
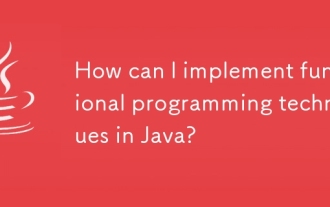
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
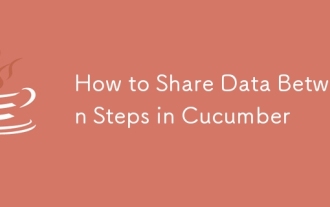
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
