How to merge two lists in python
The method of merging lists in python is as follows:
Method 1
The most original and stupidest method is to Take out all the elements from a list and put them into a new list. The sample code is as follows:
list1 = [1,2,3] list2 = [4,5,6] list_new = [] for item in list1: list_new.append(item) for item in list2: list_new.append(item) print(list_new)
Method 2
A built-in function zip() in python is used here. Its function can be seen from the name. To come out is to package several originally unrelated contents together. Look at the code:
a = [1,2,3] b = [4,5,6] c = zip(a,b) //c = [(1,4),(2,5),(3,6)] list_new = [row[i] for i in range(len(0)) for row in c]
This is python2 syntax
Method three
Use merge directly
a = [1,2,3] b = [4,5,6] c = a + b
Method 4
Use the append method
a = [1,2,3] b = [4,5,6] a.append(b)
Note: The append() method adds the b list as a whole to the a list, and the output result is
[1, 2, 3, [4, 5, 6]]
The above is the detailed content of How to merge two lists in python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


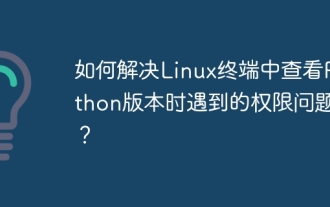
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
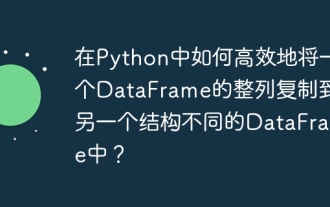
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
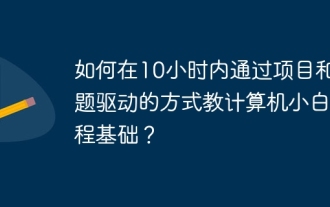
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
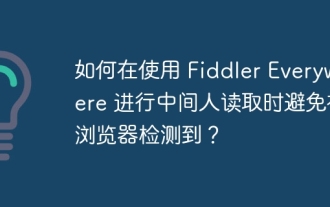
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
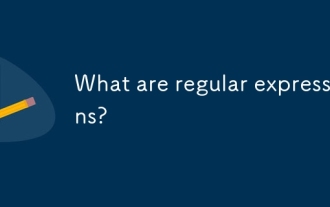
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
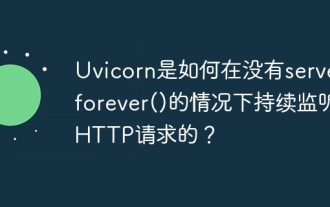
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
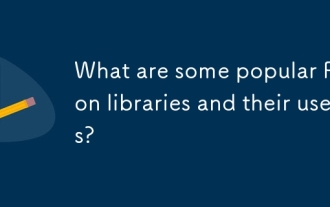
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
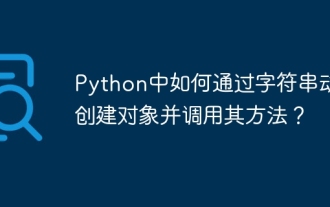
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
