How to call map function in python3
How to call the map function in python3?
The map function calling syntax in python3:
map(function, iterable, ...)
The python source code is explained as follows:
map(func, *iterables) --> map object Make an iterator that computes the function using arguments from each of the iterables. Stops when the shortest iterable is exhausted.
Simply put,
map() receives A function f and an iterable object (here understood as a list), and by applying the function f to each element of the list in turn, a new list is obtained and returned.
For example, for list [1, 2, 3, 4, 5, 6, 7, 8, 9]
If you want to square each element of the list, you can use map() function:
Therefore, we only need to pass in the function f(x)=x*x, and then we can use the map() function to complete this calculation:
def f(x): return x*x print(list(map(f, [1, 2, 3, 4, 5, 6, 7, 8, 9])))
Output result:
[1, 4, 9, 10, 25, 36, 49, 64, 81]
Use with anonymous functions:
data = list(range(10)) print(list(map(lambda x: x * x, data))) [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Note: The map() function does not change the original list, but returns a new list.
Using the map() function, you can convert a list into another list by passing in the conversion function.
Since the elements contained in the list can be of any type, map() can not only handle lists containing only numerical values, in fact it can handle lists containing any type, as long as the incoming function f can handle it this data type.
Task
Assuming that the English name entered by the user is not standardized and does not follow the rules of capitalizing the first letter and lowercase subsequent letters, please use the map() function to convert a list (including several non-standard names) English name) into a list containing standardized English names:
def f(s): return s[0:1].upper() + s[1:].lower() list_ = ['lll', 'lKK', 'wXy'] a = map(f, list_) print(a) print(list(a))
Running results:
<map object at 0x000001AD0A334908> ['Lll', 'Lkk', 'Wxy']
Related recommendations: "Python Tutorial"
The above is the detailed content of How to call map function in python3. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


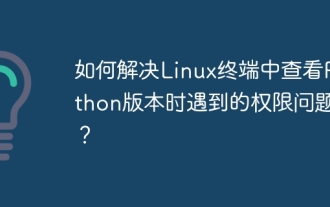
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
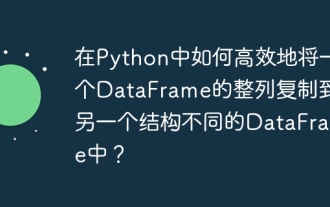
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
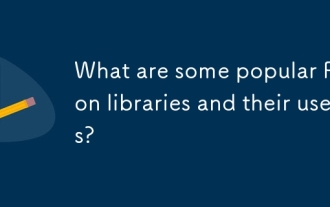
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
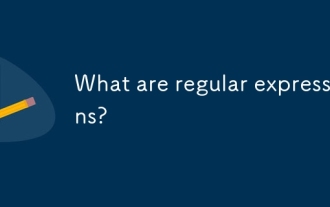
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
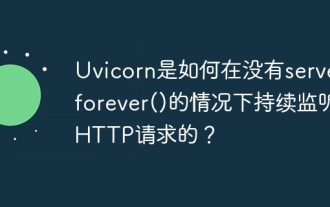
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
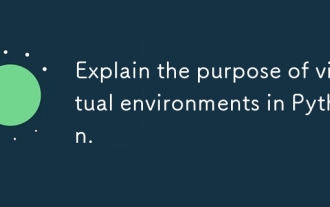
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.
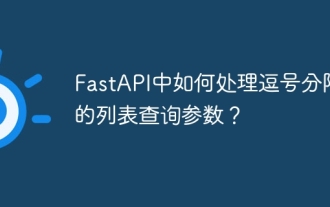
Fastapi ...
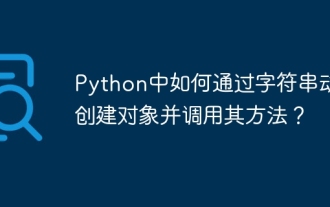
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
