Learn javascript global variables with me_javascript skills
1. Use global objects as little as possible
The problem with global variables is that these global variables are shared by all the code in your JavaScript application and the web page. They live in the same global namespace, so when two different parts of the program define the same name but have different functions When using global variables, naming conflicts are inevitable.
It is also common for web pages to contain code that was not written by the developer of the page, for example:
- Third-party JavaScript library
- Advertiser’s script code
- Third-party user tracking and analysis script code
- Different types of widgets, flags and buttons
For example, the third-party script defines a global variable called result; then, also defines a global variable named result in your function. The result is that the later variables overwrite the previous ones, and the third-party script suddenly burps!
Because if you accidentally modify the global variable somewhere in the code, it will cause errors in other modules that rely on the global variable. Moreover, the cause of the error is difficult to debug and find.
Furthermore, the window object must be used to run the web page, and the browser engine has to traverse the properties of the window again, resulting in reduced performance.
- Global variables are the link between different modules. Modules can only access the functions provided by each other through global variables
- Never use global variables when you can use local variables
var i,n,sum//globals function averageScore(players){ sum =0; for(i = 1, i = player.length; i<n; i++){ sum += score(players[i]); } return sum/n; }
Keep these variables local and only use them as part of the code that needs to use them.
function averageScore(players){ var i,n,sum; sum =0; for(i = 1, i = player.length; i<n; i++){ sum += score(players[i]); } return sum/n; }
In the browser, the this keyword will point to the global window object
JavaScript's global namespace is also exposed as a global object accessible in the program's global scope, which serves as the initial value of the this keyword. In web browsers, global objects are bound to the global window variable. Adding or modifying a global variable automatically updates the global object.
this.foo; //undefined foo ="global foo"; //"global foo" this.foo; //"global foo"
Similarly, updating the global object will automatically update the global namespace:
var foo ="global foo"; this.foo; //"global foo" this.foo ="changed"; foo; //"changed"
Two ways to change the global object, declaring it through the var keyword and setting attributes on the global object (through the this keyword)
Use global objects to perform feature detection (Feature Detection) for the current running environment. For example, ES5 provides a JSON object to operate JSON data, then you can use if (this.JSON) to determine whether the current running environment supports it. JSON
if(!this.JSON){ this.JSON ={ parse:..., stringify:... } }
2. How to avoid global variables
Method 1: Create only one global variable.
MYAPP.stooge = { "first-name": "Joe", "last-name": "Howard" }; MYAPP.flight = { airline: "Oceanic", number: 815, departure: { IATA: "SYD", time: "2004-09-22 14:55", city: "Sydney" }, arrival: { IATA: "LAX", time: "2004-09-23 10:42", city: "Los Angeles" } };
Method 2: Use module mode
var serial_maker = function ( ) { // Produce an object that produces unique strings. A // unique string is made up of two parts: a prefix // and a sequence number. The object comes with // methods for setting the prefix and sequence // number, and a gensym method that produces unique // strings. var prefix = ''; var seq = 0; return { set_prefix: function (p) { prefix = String(p); }, set_seq: function (s) { seq = s; }, gensym: function ( ) { var result = prefix + seq; seq += 1; return result; } }; }( ); var seqer = serial_maker( ); seqer.set_prefix = 'Q'; seqer.set_seq = 1000; var unique = seqer.gensym( ); // unique is "Q1000"
The so-called module mode is to create a function, which includes private variables and a privileged object. The content of the privileged object is that the private variables can be accessed using closures. function, and finally returns the privileged object.
First of all, method two can not only be used as a global variable, but can also be used to declare global variables locally. Because even if you modify seqer somewhere unknown, an error will be reported immediately because it is an object, not a string.
Method 3: Zero global variables
Zero global variables are actually a local variable processing method adopted to adapt to a small section of closed code, and are only suitable for use in some special scenarios. The most common ones are completely independent scripts that are not accessed by other scripts.
To use zero global variables, you need to use an immediate execution function. The usage is as follows.
( function ( win ) { 'use strict' ; var doc = win.document ; //在此定义其他的变量并书写代码 } )
3. Unexpected global variables
Due to two characteristics of JavaScript, it is surprisingly easy to create global variables unconsciously. First, you can use variables without even declaring them; second, JavaScript has the concept of implicit globals, which means that any variable you don't declare becomes a global object property. Refer to the code below:
function sum(x, y) { // 不推荐写法: 隐式全局变量 result = x + y; return result; }
The result in this code is not declared. The code still works fine, but after calling the function you end up with an extra global namespace, which can be a source of problems.
The rule of thumb is to always use var to declare variables, as demonstrated by the improved sum() function:
function sum(x, y) { var result = x + y; return result; }
Another counter-example to creating implicit global variables is using task chains for partial var declarations. In the following snippet, a is a local variable but b is a global variable, which is probably not what you want:
// 反例,勿使用 function foo() { var a = b = 0; // ... }
此现象发生的原因在于这个从右到左的赋值,首先,是赋值表达式b = 0,此情况下b是未声明的。这个表达式的返回值是0,然后这个0就分配给了通过var定义的这个局部变量a。换句话说,就好比你输入了:
var a = (b = 0);
如果你已经准备好声明变量,使用链分配是比较好的做法,不会产生任何意料之外的全局变量,如:
function foo() { var a, b; // ... a = b = 0; // 两个均局部变量 }
然而,另外一个避免全局变量的原因是可移植性。如果你想你的代码在不同的环境下(主机下)运行,使用全局变量如履薄冰,因为你会无意中覆盖你最初环境下不存在的主机对象
总是记得通过var关键字来声明局部变量
使用lint工具来确保没有隐式声明的全局变量
以上就是对javascript的全局变量介绍,希望对大家的学习有所帮助。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
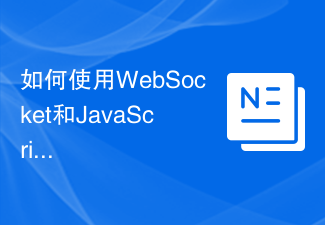
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
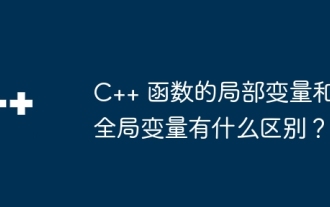
The difference between C++ local variables and global variables: Visibility: Local variables are limited to the defining function, while global variables are visible throughout the program. Memory allocation: local variables are allocated on the stack, while global variables are allocated in the global data area. Scope: Local variables are within a function, while global variables are throughout the program. Initialization: Local variables are initialized when a function is called, while global variables are initialized when the program starts. Recreation: Local variables are recreated on every function call, while global variables are created only when the program starts.
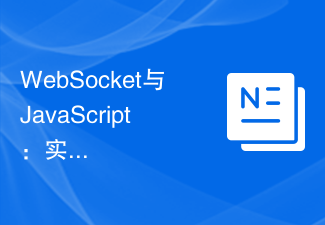
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
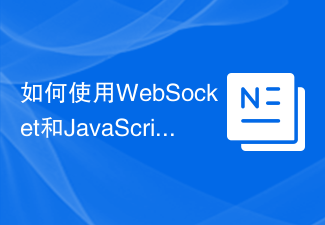
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
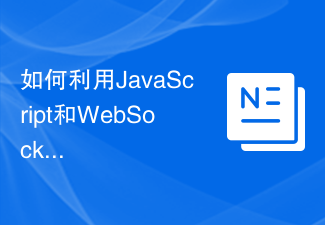
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
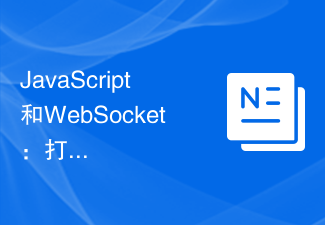
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
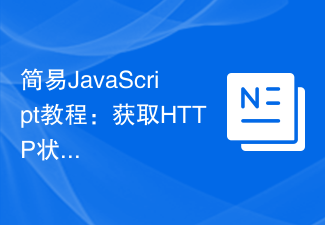
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
