Follow me to learn javascript implicit cast_javascript skills
JavaScript data types are divided into six types, namely null, undefined, boolean, string, number, object.
Object is a reference type, and the other five are basic types or primitive types. We can use the typeof method to print out which type something belongs to. To compare variables of different types, you must first convert the type, which is called type conversion. Type conversion is also called implicit conversion. Implicit conversions usually occur with the operators addition, subtraction, multiplication, division, equals, and less than, greater than, etc. .
typeof '11' //string typeof(11) //number '11' < 4 //false
1. Basic type conversion
Let’s talk about addition, subtraction, multiplication and division first:
1. Add numbers to a string, and the numbers will be converted into strings.
2. Subtract a string from a number, and convert the string into a number . If the string is not a pure number, it will be converted to NaN. The same goes for string minus numbers. Subtracting two strings also converts them into numbers first.
3. The same applies to conversions of multiplication, division, greater than, less than and subtraction.
//隐式转换 + - * == / // + 10 + '20' //'2010' // - 10 - '20' //-10 10 - 'one' //NaN 10 - '100a' //NaN // * 10*'20' //200 '10'*'20' //200 // / 20/'10' //2 '20'/'10' //2 '20'/'one' //NaN
4. The order of addition operations is sensitive
Mixed expressions like this are sometimes confusing because JavaScript is sensitive to the order of operations. For example, expression: 1 2 "3"; //"33"
Since the addition operation is left associative (i.e. left associative law), it is equivalent to the following expression: (1 2) "3"; //"33"
In contrast, the expression: 1 "2" 3; //"123" evaluates to the string "123". The left associative law is equivalent to wrapping the addition operation on the left side of the expression in parentheses.
5. Let’s take a look at another group ==
1).undefined equals null
2). When comparing strings and numbers, convert strings to numbers
3). When the number is a Boolean comparison, Boolean is converted to a number
4). When comparing strings and Boolean, convert the two into numbers
// == undefined == null; //true '0' == 0; //true,字符串转数字 0 == false; //true,布尔转数字 '0' == false; //true,两者转数字 null == false; //false undefined == false; //false
7 false values: false, 0, -0, "", NaN, null and undefined, all other values are truth
6. NaN, not a number
NaN is a special value indicating that the result of certain arithmetic operations (such as finding the square root of a negative number) is not a number. The methods parseInt() and parseFloat() return this value when the specified string cannot be parsed. For some functions that normally return valid numbers, you can also use this method and use Number.NaN to indicate its error conditions.
Math.sqrt(-2) Math.log(-1) 0/0 parseFloat('foo')
For many JavaScript beginners, the first trap is that the result returned when calling typeof is usually something you don’t expect:
console.log(typeof NaN); // 'Number'
In this case, NaN does not mean a number, its type is number. Do you understand?
Because typeof returns a string, there are six types: "number", "string", "boolean", "object", "function", "undefined
Keep calm because there’s a lot of chaos down there. Let's compare two NaNs:
var x = Math.sqrt(-2); var y = Math.log(-1); console.log(x == y); // false
Maybe this is because we are not using strict equivalence (===) operation? Apparently not.
var x = Math.sqrt(-2); var y = Math.log(-1); console.log(x === y); // false
What about comparing two NaNs directly?
console.log(NaN === NaN); // false
Because there are many ways to represent a non-number, it still makes sense that a non-number will not be equal to another non-number that is NaN.
But of course, the solution is now available. Let’s get to know the global function isNaN:
console.log(isNaN(NaN)); // true
Alas, but isNaN() also has many flaws of its own:
console.log(isNaN('hello')); // true console.log(isNaN(['x'])); // true console.log(isNaN({})); // true
This leads to many different solutions. One takes advantage of the non-reflective nature of NaN (e.g., look at Kit Cambridge's notes)
var My = { isNaN: function (x) { return x !== x; } }
Fortunately, in the upcoming ECMAScript 6, there is a Number.isNaN() method that provides reliable NaN value detection.
In other words, true will only be returned if the argument is a true NaN
console.log(Number.isNaN(NaN)); // true console.log(Number.isNaN(Math.sqrt(-2))); // true console.log(Number.isNaN('hello')); // false console.log(Number.isNaN(['x'])); // false console.log(Number.isNaN({})); // false
二、引用类型的转换
基本类型间的比较相对简单。引用类型和基本类型的比较就相对复杂一些,先要把引用类型转成基本类型,再按上述的方法比较。
1.引用类型转布尔全是true。
比如空数组,只要是对象就是引用类型,所以[]为true。引用类型转数字或者字符串就要用valueOf()或者toString();对象本身就继承了valuOf()和toString(),还可以自定义valueOf()和toString()。根据不同的对象用继承的valueOf()转成字符串,数字或本身,而对象用toString就一定转为字符串。一般对象默认调用valueOf()。
1).对象转数字时,调用valueOf();
2).对象转字符串时,调用toString();
先看看下面的例子:
0 == []; // true, 0 == [].valueOf(); ---> 0 == 0; '0' == []; // false, '0' == [].toString(); ---> '0' == ''; 2 == ['2']; // true, 2 == ['2'].valueOf(); ---> 2 == '2' ---> 2 == 2; '2' == [2]; // true, '2' == [2].toString(); ---> '2' =='2'; [] == ![]; //true, [].valueOf() == !Boolean([]) -> 0 == false ---> 0 == 0;
对象转成数字时,调用valueOf(),在这之前先调用的是toString();所以我猜valueOf方法是这样的。So上面的例子 0 == []要改成下面更合理。无论如何,[]最后是转成0的。
var valueOf = function (){ var str = this.toString(); //先调用toString(),转成字符串 //... } 0 == []; // true, 0 == [].valueOf(); -> 0 == '0' -> 0 == 0;
自定义的valueOf()和toString();
- 自定义的valueOf()和toString()都存在,会默认调用valueOf();
- 如果只有toString(),则调用toString();
var a = [1]; a.valueOf = function (){ return 1;} a.toString = function (){ return '1';} a + 1; // 2, valueOf()先调用
去掉valueOf()就会调用toString()。
var a = [1]; a.valueOf = function (){ return 1;} a.toString = function (){ return '1';} a + 1; // 2, 先调用valueOf() //去掉valueOf delete a.valueOf; a + 1; // '11', 调用toString()
如果返回其它会怎么样呢?
var a = [1]; a.valueOf = function (){return ;} a.toString = function (){return 1 ;}; 1 - a; //NaN
其它对象 调用valueOf()转成不同的类型:
var a = {}; a.valueOf(); //Object {} var a = []; a.valueOf(); //[] 自己本身 var a = new Date(); a.valueOf(); //1423812036234 数字 var a = new RegExp(); a.valueOf(); // /(?:)/ 正则对象
引用类型之间的比较是内存地址的比较,不需要进行隐式转换,这里不多说。
[] == [] //false 地址不一样
var a = [];
b = a;
b == a //true
2.显式转换
显式转换比较简单,可以直接用类当作方法直接转换。
Number([]); //0
String([]); //”
Boolean([]); //true
还有更简单的转换方法。
3 + ” // 字符串'3'
+'3' // 数字3
!!'3' // true
以上就是本文的全部内容,详细介绍了javascript的隐式强制转换,希望对大家的学习有所帮助。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
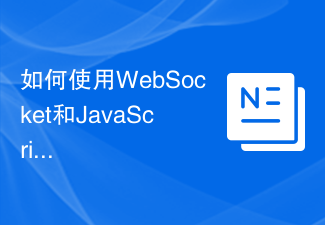
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
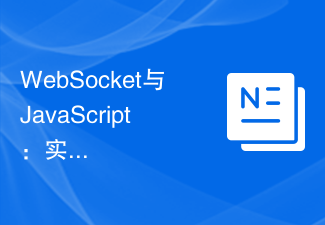
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
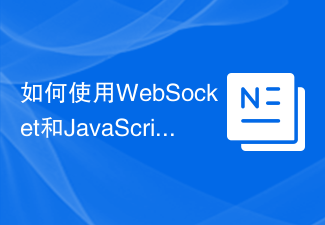
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
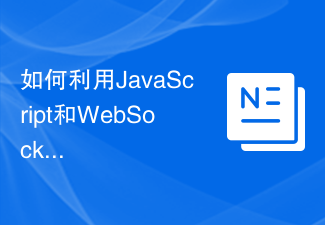
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
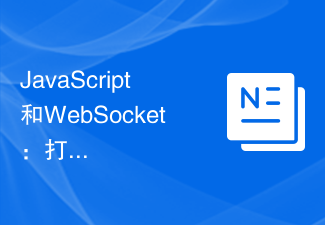
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
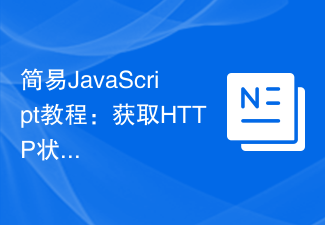
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
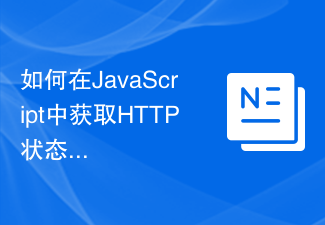
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
