Introduction to using NPM
NPM is a package management tool installed along with NodeJS. It can solve many problems in NodeJS code deployment. Common usage scenarios include the following:
Allow users to log in from the NPM server Download third-party packages written by others and use them locally.
Allows users to download and install command line programs written by others from the NPM server for local use.
Allows users to upload packages or command line programs they write to the NPM server for others to use.
Since the new version of nodejs has integrated npm, npm has also been installed before. You can also test whether the installation is successful by entering "npm -v". The command is as follows. If the version prompt appears, it means the installation is successful:
$ npm -v 2.3.0
If you are installing an old version of npm, you can easily upgrade it through the npm command. The command is as follows:
$ sudo npm install npm -g /usr/local/bin/npm -> /usr/local/lib/node_modules/npm/bin/npm-cli.js npm@2.14.2 /usr/local/lib/node_modules/npm
If it is Window The system can use the following command:
npm install npm -g
Use the Taobao mirror command:
npm install -g cnpm --registry=https://registry.npm.taobao.orgCopy after login
Use the npm command to install the module
npm The syntax format for installing Node.js modules is as follows:
$ npm install <Module Name>
In the following example, we use the npm command to install the commonly used Node.js web framework module express:
$ npm install express
After installation, the express package is placed in the node_modules directory under the project directory, so you only need to use require('express') in the code without specifying the third-party package path.
var express = require('express');
Global installation and local installation
npm package installation is divided into local installation (local) and global installation (global) There are two types. Judging from the command line, the difference is only whether there is -g. For example,
npm install express # 本地安装 npm install express -g # 全局安装
If the following error occurs:
npm err! Error: connect ECONNREFUSED 127.0.0.1:8087
The solution is:
$ npm config set proxy null
Local installation
1. Place the installation package under ./node_modules (the directory where you run the npm command). If there is no node_modules directory, it will be executed currently. The node_modules directory is generated under the directory of the npm command.
2. Locally installed packages can be introduced through require().
Global installation
1. Place the installation package under /usr/local or the installation directory of your node .
2. Can be used directly in the command line.
If you want to have the functionality of both, you need to install it in two places or use npm link.
Next we use the global method to install express
$ npm install express -g
The installation process outputs the following content. The first line outputs the module version number and installation location.
express@4.13.3 node_modules/express ├── escape-html@1.0.2 ├── range-parser@1.0.2 ├── merge-descriptors@1.0.0 ├── array-flatten@1.1.1 ├── cookie@0.1.3 ├── utils-merge@1.0.0 ├── parseurl@1.3.0 ├── cookie-signature@1.0.6 ├── methods@1.1.1 ├── fresh@0.3.0 ├── vary@1.0.1 ├── path-to-regexp@0.1.7 ├── content-type@1.0.1 ├── etag@1.7.0 ├── serve-static@1.10.0 ├── content-disposition@0.5.0 ├── depd@1.0.1 ├── qs@4.0.0 ├── finalhandler@0.4.0 (unpipe@1.0.0) ├── on-finished@2.3.0 (ee-first@1.1.1) ├── proxy-addr@1.0.8 (forwarded@0.1.0, ipaddr.js@1.0.1) ├── debug@2.2.0 (ms@0.7.1) ├── type-is@1.6.8 (media-typer@0.3.0, mime-types@2.1.6) ├── accepts@1.2.12 (negotiator@0.5.3, mime-types@2.1.6) └── send@0.13.0 (destroy@1.0.3, statuses@1.2.1, ms@0.7.1, mime@1.3.4, http-errors@1.3.1)
View installation information
You can use the following command to view all globally installed modules:
$ npm list -g ├─┬ cnpm@4.3.2 │ ├── auto-correct@1.0.0 │ ├── bagpipe@0.3.5 │ ├── colors@1.1.2 │ ├─┬ commander@2.9.0 │ │ └── graceful-readlink@1.0.1 │ ├─┬ cross-spawn@0.2.9 │ │ └── lru-cache@2.7.3 ……
If you want to view the version of a module No., you can use the following command:
$ npm list grunt projectName@projectVersion /path/to/project/folder └── grunt@0.4.1
Use package.json
package.json is located in the directory of the module, for Define the properties of the package. Next, let’s take a look at the package.json file of the express package, located at node_modules/express/package.json. Content:
{ "name": "express", "description": "Fast, unopinionated, minimalist web framework", "version": "4.13.3", "author": { "name": "TJ Holowaychuk", "email": "tj@vision-media.ca" }, "contributors": [ { "name": "Aaron Heckmann", "email": "aaron.heckmann+github@gmail.com" }, { "name": "Ciaran Jessup", "email": "ciaranj@gmail.com" }, { "name": "Douglas Christopher Wilson", "email": "doug@somethingdoug.com" }, { "name": "Guillermo Rauch", "email": "rauchg@gmail.com" }, { "name": "Jonathan Ong", "email": "me@jongleberry.com" }, { "name": "Roman Shtylman", "email": "shtylman+expressjs@gmail.com" }, { "name": "Young Jae Sim", "email": "hanul@hanul.me" } ], "license": "MIT", "repository": { "type": "git", "url": "git+https://github.com/strongloop/express.git" }, "homepage": "http://expressjs.com/", "keywords": [ "express", "framework", "sinatra", "web", "rest", "restful", "router", "app", "api" ], "dependencies": { "accepts": "~1.2.12", "array-flatten": "1.1.1", "content-disposition": "0.5.0", "content-type": "~1.0.1", "cookie": "0.1.3", "cookie-signature": "1.0.6", "debug": "~2.2.0", "depd": "~1.0.1", "escape-html": "1.0.2", "etag": "~1.7.0", "finalhandler": "0.4.0", "fresh": "0.3.0", "merge-descriptors": "1.0.0", "methods": "~1.1.1", "on-finished": "~2.3.0", "parseurl": "~1.3.0", "path-to-regexp": "0.1.7", "proxy-addr": "~1.0.8", "qs": "4.0.0", "range-parser": "~1.0.2", "send": "0.13.0", "serve-static": "~1.10.0", "type-is": "~1.6.6", "utils-merge": "1.0.0", "vary": "~1.0.1" }, "devDependencies": { "after": "0.8.1", "ejs": "2.3.3", "istanbul": "0.3.17", "marked": "0.3.5", "mocha": "2.2.5", "should": "7.0.2", "supertest": "1.0.1", "body-parser": "~1.13.3", "connect-redis": "~2.4.1", "cookie-parser": "~1.3.5", "cookie-session": "~1.2.0", "express-session": "~1.11.3", "jade": "~1.11.0", "method-override": "~2.3.5", "morgan": "~1.6.1", "multiparty": "~4.1.2", "vhost": "~3.0.1" }, "engines": { "node": ">= 0.10.0" }, "files": [ "LICENSE", "History.md", "Readme.md", "index.js", "lib/" ], "scripts": { "test": "mocha --require test/support/env --reporter spec --bail --check-leaks test/ test/acceptance/", "test-ci": "istanbul cover node_modules/mocha/bin/_mocha --report lcovonly -- --require test/support/env --reporter spec --check-leaks test/ test/acceptance/", "test-cov": "istanbul cover node_modules/mocha/bin/_mocha -- --require test/support/env --reporter dot --check-leaks test/ test/acceptance/", "test-tap": "mocha --require test/support/env --reporter tap --check-leaks test/ test/acceptance/" }, "gitHead": "ef7ad681b245fba023843ce94f6bcb8e275bbb8e", "bugs": { "url": "https://github.com/strongloop/express/issues" }, "_id": "express@4.13.3", "_shasum": "ddb2f1fb4502bf33598d2b032b037960ca6c80a3", "_from": "express@*", "_npmVersion": "1.4.28", "_npmUser": { "name": "dougwilson", "email": "doug@somethingdoug.com" }, "maintainers": [ { "name": "tjholowaychuk", "email": "tj@vision-media.ca" }, { "name": "jongleberry", "email": "jonathanrichardong@gmail.com" }, { "name": "dougwilson", "email": "doug@somethingdoug.com" }, { "name": "rfeng", "email": "enjoyjava@gmail.com" }, { "name": "aredridel", "email": "aredridel@dinhe.net" }, { "name": "strongloop", "email": "callback@strongloop.com" }, { "name": "defunctzombie", "email": "shtylman@gmail.com" } ], "dist": { "shasum": "ddb2f1fb4502bf33598d2b032b037960ca6c80a3", "tarball": "http://registry.npmjs.org/express/-/express-4.13.3.tgz" }, "directories": {}, "_resolved": "https://registry.npmjs.org/express/-/express-4.13.3.tgz", "readme": "ERROR: No README data found!" }
Package.json Attribute Description
name - package name.
version - The version number of the package.
description - Description of the package.
homepage - the official website url of the package.
author - The name of the author of the package.
contributors - Names of other contributors to the package.
dependencies - list of dependent packages. If the dependent package is not installed, npm will automatically install the dependent package in the node_module directory.
repository - The type of place where the package code is stored, it can be git or svn, git can be on Github.
main - The main field specifies the main entry file of the program, and require('moduleName') will load this file. The default value of this field is index.js under the module root directory.
keywords - keywords
##Uninstall module
We can use the following command to uninstall the Node.js module.$ npm uninstall express
$ npm ls
Update module
$ npm update express
##Search moduleUse the following to search for modules:
$ npm search express
Create moduleTo create a module, the package.json file is essential. We can use NPM to generate a package.json file, and the generated file contains the basic results.
$ npm init This utility will walk you through creating a package.json file. It only covers the most common items, and tries to guess sensible defaults. See `npm help json` for definitive documentation on these fields and exactly what they do. Use `npm install <pkg> --save` afterwards to install a package and save it as a dependency in the package.json file. Press ^C at any time to quit. name: (node_modules) runoob # 模块名 version: (1.0.0) description: Node.js 测试模块(www.runoob.com) # 描述 entry point: (index.js) test command: make test git repository: https://github.com/runoob/runoob.git # Github 地址 keywords: author: license: (ISC) About to write to ……/node_modules/package.json: # 生成地址 { "name": "runoob", "version": "1.0.0", "description": "Node.js 测试模块(www.runoob.com)", …… } Is this ok? (yes) yes
You need to enter the above information according to your own situation. After entering "yes" at the end, the package.json file will be generated.
Next we can use the following command to register a user in the npm resource library (use email to register):
$ npm adduser Username: mcmohd Password: Email: (this IS public) mcmohd@gmail.com
Next we use the following command to publish the module:
$ npm publish
如果你以上的步骤都操作正确,你就可以跟其他模块一样使用 npm 来安装。
版本号
使用NPM下载和发布代码时都会接触到版本号。NPM使用语义版本号来管理代码,这里简单介绍一下。
语义版本号分为X.Y.Z三位,分别代表主版本号、次版本号和补丁版本号。当代码变更时,版本号按以下原则更新。
如果只是修复bug,需要更新Z位。
如果是新增了功能,但是向下兼容,需要更新Y位。
如果有大变动,向下不兼容,需要更新X位。
版本号有了这个保证后,在申明第三方包依赖时,除了可依赖于一个固定版本号外,还可依赖于某个范围的版本号。例如"argv": "0.0.x"表示依赖于0.0.x系列的最新版argv。
NPM支持的所有版本号范围指定方式可以查看官方文档。
NPM 常用命令
除了本章介绍的部分外,NPM还提供了很多功能,package.json里也有很多其它有用的字段。
除了可以在【https://npmjs.org/doc/】查看官方文档外,这里再介绍一些NPM常用命令。
NPM提供了很多命令,例如install和publish,使用npm help可查看所有命令。
NPM提供了很多命令,例如install和publish,使用npm help可查看所有命令。
使用npm help
可查看某条命令的详细帮助,例如npm help install。 在package.json所在目录下使用npm install . -g可先在本地安装当前命令行程序,可用于发布前的本地测试。
使用npm update
可以把当前目录下node_modules子目录里边的对应模块更新至最新版本。 使用npm update
-g可以把全局安装的对应命令行程序更新至最新版。 使用npm cache clear可以清空NPM本地缓存,用于对付使用相同版本号发布新版本代码的人。
使用npm unpublish
@ 可以撤销发布自己发布过的某个版本代码。
使用淘宝 NPM 镜像
大家都知道国内直接使用 npm 的官方镜像是非常慢的,这里推荐使用淘宝 NPM 镜像。
淘宝 NPM 镜像是一个完整 npmjs.org 镜像,你可以用此代替官方版本(只读),同步频率目前为 10分钟 一次以保证尽量与官方服务同步。
你可以使用淘宝定制的 cnpm (gzip 压缩支持) 命令行工具代替默认的 npm:
$ npm install -g cnpm --registry=https://registry.npm.taobao.org
这样就可以使用 cnpm 命令来安装模块了:
$ cnpm install [name]
更多信息可以查阅:http://npm.taobao.org/。
The above is the detailed content of Introduction to using NPM. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


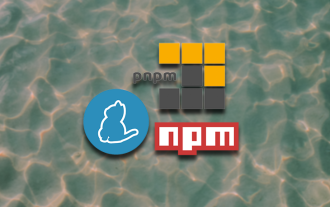
This article will take you through the three JavaScript package managers (npm, yarn, pnpm), compare these three package managers, and talk about the differences and relationships between npm, yarn, and pnpm. I hope it will be helpful to everyone. Please help, if you have any questions please point them out!

Solution to npm react installation error: 1. Open the "package.json" file in the project and find the dependencies object; 2. Move "react.json" to "devDependencies"; 3. Run "npm audit in the terminal --production" to fix the warning.
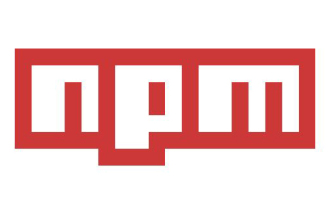
This article will give you a detailed explanation of the package.json and package-lock.json files. I hope it will be helpful to you!
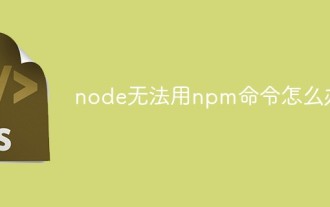
The reason why node cannot use the npm command is because the environment variables are not configured correctly. The solution is: 1. Open "System Properties"; 2. Find "Environment Variables" -> "System Variables", and then edit the environment variables; 3. Find the location of nodejs folder; 4. Click "OK".
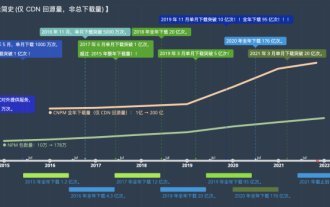
npm is the package management tool for the node.js library. Because the mirror address is abroad, the installation of the library will be slow. You can change the mirror address to a domestic address (Taobao mirror) to improve the speed of installing the library.
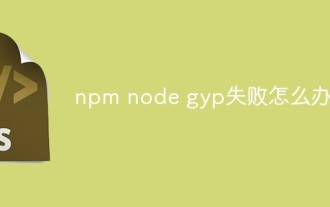
npm node gyp fails because "node-gyp.js" does not match the version of "Node.js". The solution is: 1. Clear the node cache through "npm cache clean -f"; 2. Through "npm install -g n" Install the n module; 3. Install the "node v12.21.0" version through the "n v12.21.0" command.
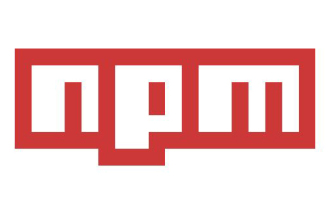
npm is the package management tool of the JavaScript world and is the default package management tool for the Node.js platform. Through npm, you can install, share, distribute code, and manage project dependencies. This article will take you through the principles of npm, I hope it will be helpful to you!
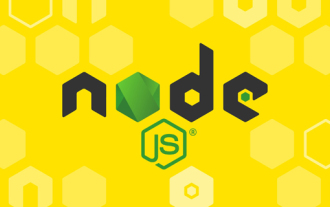
npm means "node package manager" in Chinese. It is the default package management tool of the Node.js platform. It will be installed together with Nodejs. npm manages third-party plug-ins corresponding to node.js; you can install, share, and distribute code through npm. , manage node project dependencies.
