What method is used to generate unique random numbers in java
Method 1: Call the random() method in the Math class below java.lang to generate random numbers
Create a new file with the suffix name java, and the file name is MyRandom, write the following code in this class:
public class MyRandom { public static void main(String[] args) { int radom = (int)(Math.random()*10); System.out.println(radom); } }
Among them, Math.random() generates a random decimal between 0 and 1.
Generate an integer between 0 and 9: (int)(Math.random()*10)
Generate an integer between 1 and 10 Then you can write: (int)(Math.random()*10 1)
and so on: to generate a number between 0~n, you should write: Math.random()*n
Get a random positive integer with a specified length:
public static int buildRandom(int length) { int num = 1; double random = Math.random(); if (random < 0.1) { random = random + 0.1; } for (int i = 0; i < length; i++) { num = num * 10; } return (int) ((random * num)); }
Method 2: Call the Random class below in java.util. Examples of this class are used To generate a pseudo-random number stream and generate a random integer, call the nextInt() method of the class. Before using the Random class, import java.util.Random
under the package. The code is as follows:
import java.util.Random; public class MyRandom { public static void main(String[] args) { Random rand = new Random(); int rInt = rand.nextInt(10); System.out.println(rInt); } }
WhereRandom rand = new Random()
is to create a new random number generator; rand.nextInt(int n)
is taken from the sequence of this random number generator , an int value uniformly distributed between 0 (inclusive) and the specified value n (exclusive).
In Java, in the specified integer range class, the loop generates different random numbers.
Take the example of generating 6 different random integers within 20 digits as follows:
public class MyRandom { public static void main(String[] args) { int n = 20; Random rand = new Random(); boolean[] bool = new boolean[n]; int randInt = 0; for(int i = 0; i < 6 ; i++) { do { randInt = rand.nextInt(n); }while(bool[randInt]); bool[randInt] = true; System.out.println(randInt); } } }
Among them, a Boolean variable array is used to store whether the number is generated. After generation, the number becomes true as the corresponding Boolean value in the table below the Boolean array. The next time the number is generated, it will enter the do...while loop again to generate numbers until a number that has not been generated before is generated.
Recommended tutorial: Getting started with java development
The above is the detailed content of What method is used to generate unique random numbers in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


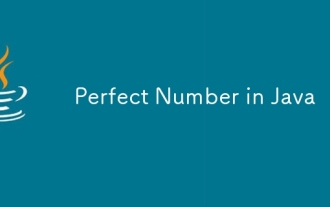
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
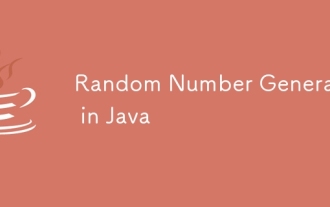
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
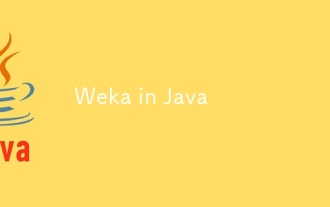
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
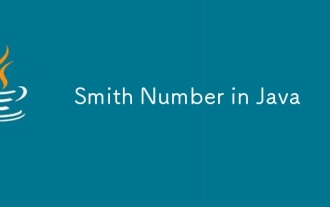
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
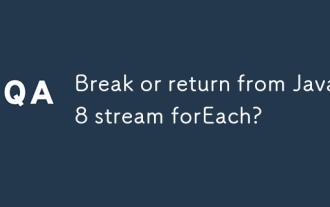
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
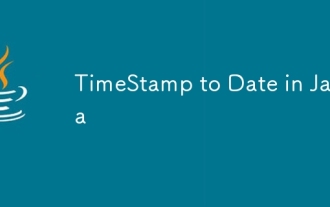
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
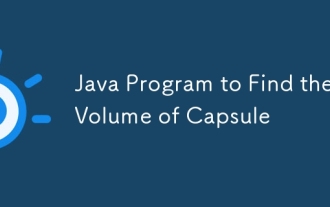
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
