What are static objects and methods in java
What is a static variable
Members modified by static in Java are called static members or class members. It belongs to the entire class, not to an object, that is, it is shared by all objects of the class. Static members can be accessed directly using the class name, or they can be accessed using the object name.
Use static to modify variables, methods and code blocks.
public class Test1 { // static修饰的变量为静态变量,所有类的对象共享hobby static String hobby = "Tedu"; public static void main(String[] args) { // 静态变量可以直接使用类名来访问,无需创建对象 System.out.println("通过类名访问hobby:" + Test1.hobby); // 创建类的对象 Test1 t1 = new Test1(); // 使用对象名访问静态变量 System.out.println("使用对象名访hobby:" + t1.hobby); // 使用对象名的形式修改静态变量的值 t1.hobby = "cjj"; // 再次使用类名访问静态白变量,值已经被修改 System.out.println("通过类名访问hobby:" + Test1.hobby); } }
Running result:
通过类名访问hobby:Tedu 使用对象名访hobby:Tedu 通过类名访问hobby:cjj
Note: Static members belong to the entire class. When the system uses the class for the first time, it will allocate memory space until the Resources will be recycled only after the class is unloaded!
What is a static method
Like static variables, we can also use static modified methods, called static methods or class methods. In fact, the main method we have been writing before is a static method.
public class Test01 { //使用static关键字声明静态方法 public static void print() { System.out.println("欢迎你:Cjj!"); } public static void main(String[] args) { //直接使用类名调用静态方法 Test01.print(); //也可以通过对象名调用,当然更推荐使用类名调用 Test01 t1 = new Test01(); t1.print(); } }
Running result:
欢迎你:Cjj! 欢迎你:Cjj!
Note:
1. Static methods can directly call static members of the same class, but cannot directly call non-static members. static members. For example:
If you want to call non-static variables in a static method, you can create an object of the class and then access the non-static variables through the object. For example:
2. In ordinary member methods, you can directly access non-static variables and static variables of the same type, such as:
3. Non-static methods cannot be directly called in static methods. Non-static methods need to be accessed through objects. Such as:
public class Test01 { String name = "Cjj"; //静态成员变量 static String hobby = "study"; //非静态成员变量 //普通成员方法 public void print1() { System.out.println("普通成员方法~~~"); } //静态成员方法 public static void print2() { System.out.println("静态成员方法~~~"); } public static void main(String[] args) { //普通成员方法必须通过对象来调用 Test01 t1 = new Test01(); t1.print1(); //可以直接调用静态方法 print2(); } }
Recommended tutorial: java introductory tutorial
The above is the detailed content of What are static objects and methods in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
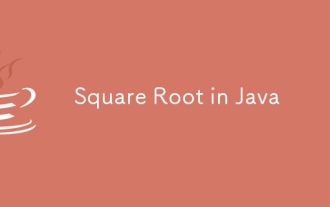
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
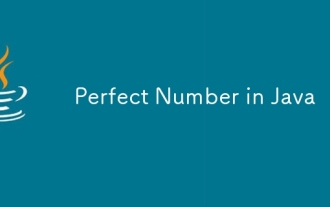
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
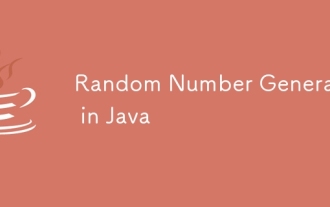
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
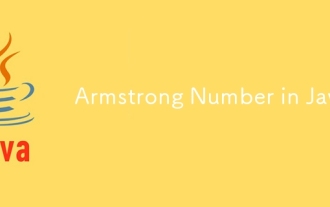
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
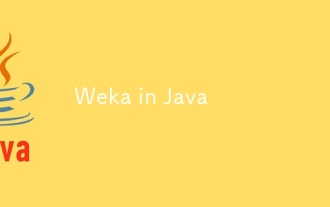
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
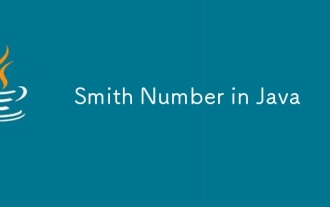
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
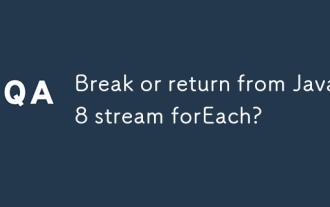
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
