How to read files in java
Methods for reading files in java: 1. Read by using the [java.io] method and read from a relative path relative to the current user directory; 2. By using the [java.lang.ClassLoader] method Read, read from a relative path relative to the classpath.
Methods to read files in java:
Two methods to read files in java: java.io And java.lang.ClassLoader
// java.io: File file = new File("..."); FileInputStream fis = new FileInputStream("..."); FileReader fr = new FileReader("..."); //ClassLoader: ClassLoader loader = XXXClass.class.getClassLoader(); ClassLoader loader2 = Thread.currentThread().getContextClassLoader(); URL url = loader.getResource("..."); File file = new File(url.getFile()); InputStream input = loader.getResourceAsStream("...");
1, classes in the java.io package always analyze relative path names based on the current user directory, which means that whether relative paths work well depends on to the value of user.dir. The system property user.dir is set when the JVM is started. It is usually the calling directory of the Java virtual machine, that is, the directory where the java command is executed.
For tomcat/jboss container, user.dir is the %home/bin%/ directory, because this directory is where we start the web container
When running the program in eclipse, eclipse will Set the value of user.dir to the root directory of the project
The user directory can be viewed using System.getProperty("user.dir")
So, use java.io to read the file , whether it is a relative path or an absolute path is not a good practice, don't use it if you can't use it (in JavaEE).
2. Use ClassLoader
Class.getResource() has 2 ways, absolute path and relative path. The absolute path starts with / and searches from the root directory of the classpath or jar package;
The relative path is relative to the directory where the current class is located, allowing you to use .. or . to locate files.
ClassLoader.getResource() can only use absolute paths and does not start with /.
These two methods of reading resource files will not depend on user.dir or the specific deployment environment. They are recommended practices (JavaEE)
How Select
java.io:
Read the relative path relative to the current user directory; focus on dealing with disk files or use in pure java projects.
Although the ClassLoader method is more versatile, if it is not a javaEE environment, it is unreasonable to locate the classpath path to read the file.
java.lang.ClassLoader:
Read relative path relative to classpath; it is recommended to use this method in the javaEE environment.
Usually, ClassLoader cannot read files that are too large. It is suitable for reading configuration files of web projects. If you need to read large files, you still need to use the IO package. You can first obtain the file through ClassLoader. The absolute path, and then passed to File or other objects, it would be better to use the objects in the io package to read.
For more java knowledge, please pay attention to java basic tutorial.
The above is the detailed content of How to read files in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


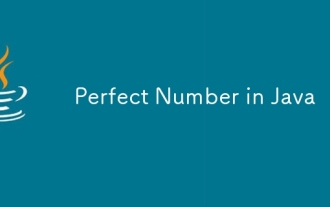
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
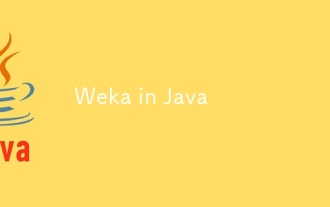
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
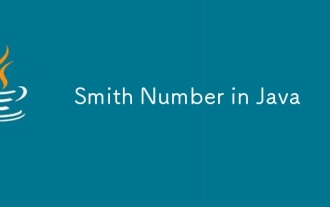
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
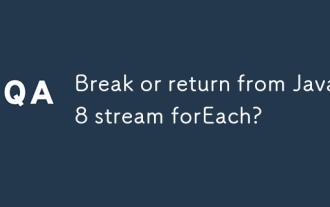
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
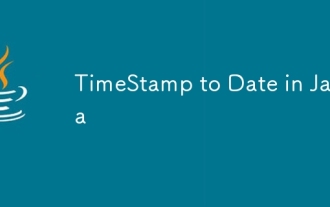
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
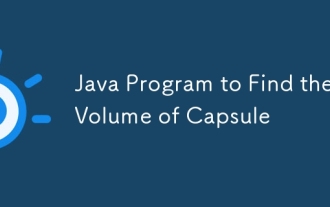
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
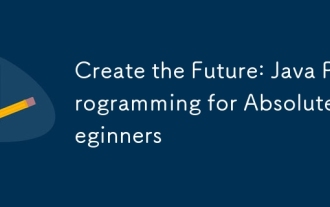
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
