A detailed introduction to String, a common class in Java
Overview
java.lang.String
class represents a string. All string literals (such as "abc") in Java programs can be regarded as instances that implement this class.
String includes methods for checking individual strings, such as for comparing strings, Searches for a string, extracts substrings, and creates a copy of the string with all characters translated to uppercase or lowercase.
Features
1. String is unchanged: the value of the string cannot be changed after creation
String s1 = "abc"; s1 += "d"; System.out.println(s1); // "abcd" // 内存中有"abc","abcd"两个对象,s1从指向"abc",改变指向,指向了"abcd"。
2 .Because String objects are immutable, they can be shared
String s1 = "abc"; String s2 = "abc"; // 内存中只有一个"abc"对象被创建,同时被s1和s2共享。
3."abc" is equivalent to char[] data = {'a','b','c'}
Online learning video sharing: java video
Usage steps
View class
java.lang.String
This class does not need to be imported.
View constructor method
public String():
Initialize the newly created String object so that it represents an empty character sequence.
public String(char[] value)
: Construct a new String from the character array in the current parameter.
public String(byte[] bytes)
: Constructs a new String by decoding the byte array in the current argument using the platform's default character set.
Construction example, the code is as follows:
Common methods
Methods for judging functions
public boolean equals (Object anObject)
: Compare this string with the specified object.
public boolean equalsIgnoreCase (String anotherString)
: Compare this string with the specified object, ignoring case
Method demonstration, the code is as follows:
Object means "object" and is also a reference type. As a parameter type, it means that any object can be passed into the method.
Methods to get the function
public int length ()
Returns the length of this string.
public String concat (String str)
: Concatenate the specified string to the end of the string.
public char charAt (int index)
: Returns the char value at the specified index.
public int indexOf (String str):
The index position of the first occurrence of this string
public String substring (int beginIndex)
: Return a substring, intercept the string from begin Index to the end of the string
public String substring (int beginIndex, int endIndex)
: Return a substring, from beginIndex to endIndex Intercept string. Contains beginIndex but does not include endIndex.
Method demonstration, the code is as follows:
public class String_Demo02 { public static void main(String[] args) { //创建字符串对象 String s = "helloworld"; // int length():获取字符串的长度,其实也就是字符个数 // System.out.println(s.length()); // System.out.println("‐‐‐‐‐‐‐‐"); // String concat (String str):将将指定的字符串连接到该字符串的末尾. // String s = "helloworld"; String s2 = s.concat("**hello itheima"); // char charAt(int index):获取指定索引处的字符 // System.out.println(s.charAt(0)); // System.out.println(s.charAt(1)); // System.out.println("‐‐‐‐‐‐‐‐"); // int indexOf(String str):获取str在字符串对象中第一次出现的索引,没有返回‐1 // System.out.println(s.indexOf("l")); // System.out.println(s.indexOf("owo")); // System.out.println(s.indexOf("ak")); System.out.println("‐‐‐‐‐‐‐‐"); // String substring(int start):从start开始截取字符串到字符串结尾 // System.out.println(s.substring(0)); // System.out.println(s.substring(5)); // System.out.println("‐‐‐‐‐‐‐‐"); // String substring(int start,int end):从start到end截取字符串。含start,不含end。 // System.out.println(s.substring(0, s.length())); // System.out.println(s.substring(3,8)); } }
public char[] toCharArray ()
: Convert this string to a new string array
public byte[] getBytes ()
: Use the platform's default character set to convert the String encoding into a new byte array
public String replace (CharSequence target, CharSequence replacement)
: Replace the string matching the target with the replacement string.
Method demonstration, the code is as follows:
public class String_Demo03 { public static void main(String[] args) { //创建字符串对象String s = "abcde"; // char[] toCharArray():把字符串转换为字符数组 // char[] chs = s.toCharArray(); for (int x = 0; x < chs.length; x++) { System.out.println(chs[x]); } System.out.println("‐‐‐‐‐‐‐‐‐‐‐"); // byte[] getBytes ():把字符串转换为字节数组 // byte[] bytes = s.getBytes(); for (int x = 0; x < bytes.length; x++) { System.out.println(bytes[x]); } System.out.println("‐‐‐‐‐‐‐‐‐‐‐"); // 替换字母it为大写IT // String replace = str.replace("it", "IT"); // System.out.println(replace); // ITcast ITheima System.out.println("‐‐‐‐‐‐‐‐‐‐‐"); } }
CharSequence is an interface and a reference type. As a parameter type, String objects can be passed into methods.
Method of splitting function
public String[] split(String regex)
Split this string according to the given regex ( rules) split into an array of strings.
Method demonstration, the code is as follows:
##String class exercises
Splicing strings
Define a method to splice arrays {1,2,3} into a string according to a specified format. The format is as follows:public class StringTest1 { public static void main(String[] args) { //定义一个int类型的数组 // int[] arr = {1, 2, 3}; //调用方法 String s = arrayToString(arr); //输出结果System.out.println("s:" + s); } /* *写方法实现把数组中的元素按照指定的格式拼接成一个字符串 *两个明确: *返回值类型:String *参数列表:int[] arr */ public static String arrayToString(int[] arr) { // 创建字符串s String s = new String("["); // 遍历数组,并拼接字符串 for (int x = 0; x < arr.length; x++) { if (x == arr.length - 1) { s = s.concat(arr[x] + "]"); } else { s = s.concat(arr[x] + "#"); } } return s; } }
Counting the number of characters
Enter a character on the keyboard and count the number of uppercase and lowercase letters and numeric characters in the string
The above is the detailed content of A detailed introduction to String, a common class in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


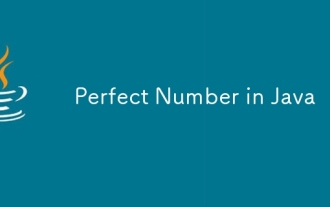
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
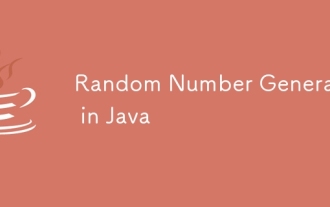
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
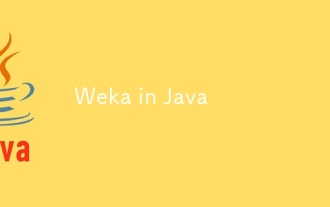
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
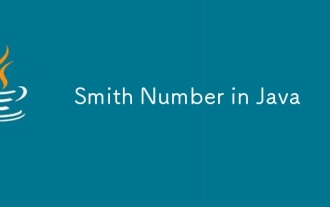
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
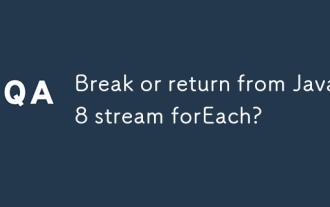
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
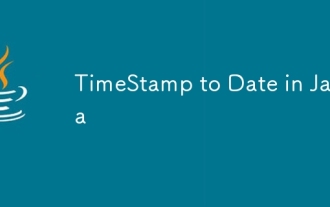
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
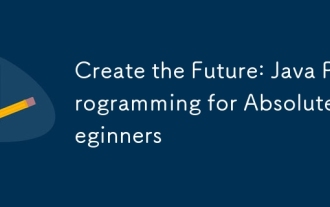
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
