String identity judgment in java
1. Cause: String identity judgment
//String is reference type String str1 = new String("hello"); String str2 = new String("hello"); System.out.println(str1==str2); // false System.out.println(str1.equals(str2)); // true
Online learning video sharing:java在线learning
2. Relational operator “==”
Points: "==" can only process the identity judgment of the corresponding value of the basic data type, and is not applicable to the reference data type (the value of which is stored in the address)
public class Main { public static void main(String[] args) { int n=3; int m=3; // true System.out.println(n==m); String str = new String("hello"); String str1 = new String("hello"); String str2 = new String("hello"); // false System.out.println(str1==str2); str1 = str; str2 = str; // true System.out.println(str1==str2); } }
n==mThe result is true, this is easy to understand. The values stored in variable n and variable m are both 3, which must be equal. And why are the results of the two comparisons of str1 and str2 different? To understand this, you only need to understand the difference between basic data type variables and non-basic data type variables.
8 basic data types in Java
Floating point type: float, double; Integer type: byte, short, int, long; Character type: char; Boolean type: boolean.
For these eight basic data types of variables, the variables directly store the "value", so when comparing using the relational operator ==, what is compared is the "value" itself. It should be noted that floating point and integer types are signed types, while char is an unsigned type.
For variables of non-basic data types (reference types), such as String type , a reference type variable stores not the "value" itself, but the address in memory of the object associated with it. For example, str1 is not the directly stored string "hello", but the address corresponding to the object.
So when you use == to compare str1 and str2 for the first time, the result is false. Because they point to different objects respectively, that is to say, the memory addresses where they are actually stored are different. In the second comparison, str1 and str2 point to the object pointed to by str at the same time, so the result is undoubtedly true (the address is the same).
3. Object method "equals()"
The equals method is a method in the base class Object, so all classes that inherit from Object will have this method . In the Object class, the equals method is used to compare whether the references of two objects are equal, that is, whether they point to the same object.
public class Main { public static void main(String[] args) { String str1 = new String("hello"); String str2 = new String("hello"); // true System.out.println(str1.equals(str2)); } }
The String class overrides the equals method to compare the pointed strings Whether the strings stored in the object are equal. Some other classes, such as Double, Date, Integer, etc., have overridden the equals method to compare whether the contents stored in the pointed objects are equal.
Summary:
For ==: If it acts on a variable of a basic data type, directly compare whether its stored "value" is equal; if it acts on a reference type variables, the address of the object pointed to is compared
For the equals method: If the equals method is not overridden, the address of the object pointed to by the reference type variable is compared; such as String, If classes such as Date override the equals method, the contents of the pointed objects are compared (the equals method cannot act on variables of basic data types).
For more related articles and tutorials, please visit: Getting Started with Java
The above is the detailed content of String identity judgment in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


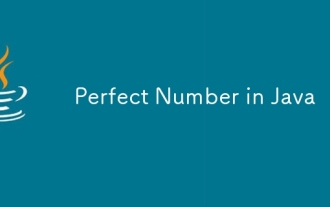
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
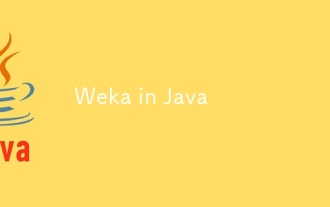
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
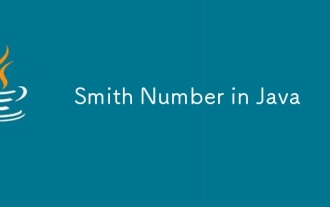
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
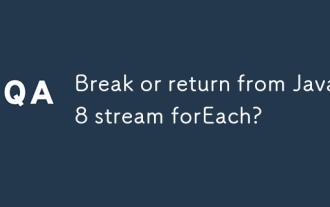
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
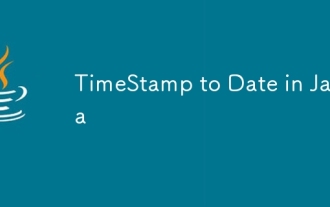
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
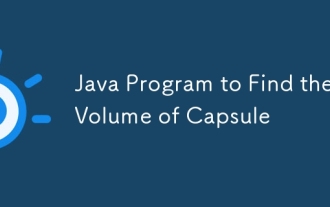
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
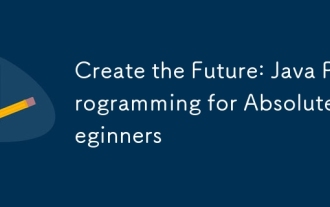
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
