Summary of usage of reference types in java
1. class as a member variable
When defining a class Role (game character), the code is as follows:
Use the int type to represent the character id and health value, and use the String type to represent the name. At this time, String itself is a reference type. Since it is used like a constant, its existence as a reference type is often ignored. If we continue to enrich the definition of this class and give the Role attributes such as equipment, how will we write it?
Free video tutorial recommendation: java free video tutorial
Define weapon class, which will increase attack capability:
Define wearing armor class, which will increase defense ability, that is, increase health value:
Define character class:
Test class:
public class Test { public static void main(String[] args) { // 创建Weapon 对象 Weapon wp = new Weapon("屠龙刀", 999999); // 创建Armour 对象 Armour ar = new Armour("麒麟甲", 10000); // 创建Role 对象 Role r = new Role(); // 设置武器属性 r.setWeapon(wp); // 设置盔甲属性 r.setArmour(ar); // 攻 击 r.attack(); // 穿戴盔甲 r.wear(); } } 输出结果: 使用屠龙刀,造成999999点伤害 穿上麒麟甲 ,生命值增加10000
Tip: When a class is used as a member variable, assigning a value to it is actually assigning it an object of the class.
2. Interface as a member variable
Interface is an encapsulation of methods, corresponding to the game, it can be regarded as an extended game Character's skills. Therefore, if we want to expand more powerful skills, we can add interfaces as member variables in Role to set different skills.
Define the interface:
Define the role class:
Define test class:
public class Test { public static void main(String[] args) { // 创建游戏角色 Role role = new Role(); // 设置角色法术技能 role.setFaShuSkill(new FaShuSkill() { @Override public void faShuAttack() { System.out.println("纵横天下"); } }); // 发动法术攻击 role.faShuSkillAttack(); // 更换技能 role.setFaShuSkill(new FaShuSkill() { @Override public void faShuAttack() { System.out.println("逆转乾坤"); } }); // 发动法术攻击 role.faShuSkillAttack(); } } 输出结果: 发动法术攻击:纵横天下攻击完毕 发动法术攻击:逆转乾坤攻击完毕
Tip: We use an interface as a member variable to change skills at any time. This design is more flexible and enhances the expansion of the program. sex. When an interface is used as a member variable, assigning a value to it is actually assigning it a subclass object of the interface.
3. Interface as method parameter and return value type
When the interface is used as a method parameter, what needs to be passed? When an interface is used as the return value type of a method, what needs to be returned? Yes, in fact, they are all subclass objects of it.
We are not unfamiliar with the ArrayList class. Looking at the API, we find that, in fact, it is the implementation class of the java.util.List
interface. So, when we see the List interface as a parameter or return value type, of course we can pass or return an ArrayList object.
Please observe the following method: Get all the even numbers in a set.
Define method:
public static List<Integer> getEvenNum(List<Integer> list) { // 创建保存偶数的集合 ArrayList<Integer> evenList = new ArrayList<>(); // 遍历集合list,判断元素为偶数,就添加到evenList中 for (int i = 0; i < list.size(); i++) { Integer integer = list.get(i); if (integer % 2 == 0) { evenList.add(integer); } } /* 返回偶数集合因为getEvenNum方法的返回值类型是List,而ArrayList是List的子类, 所以evenList可以返回 */ return evenList; }
Calling method:
## Tip: When the interface is used as a parameter, Pass its subclass object. When an interface is used as a return value type, its subclass object is returned.
Recommended java related articles and tutorials:The above is the detailed content of Summary of usage of reference types in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


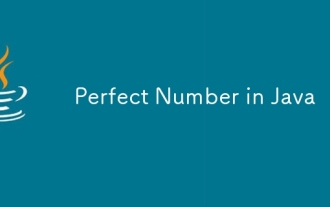
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
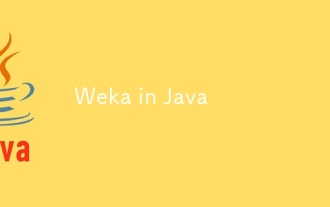
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
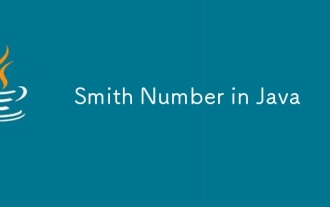
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
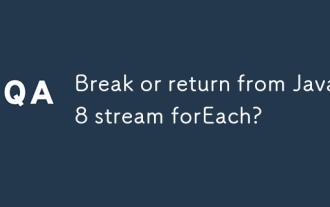
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
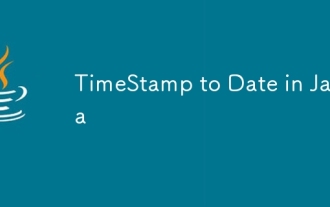
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
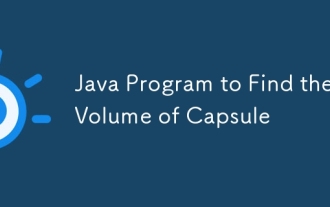
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
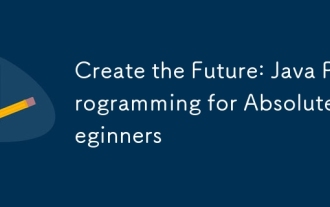
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
