How to conditionally query in yii framework
Conditional query
$customers = Customer::find()->where($cond)->all();
$cond is what we call conditions. The way of writing conditions also depends on the query data. There are differences, so how to write query conditions using yii2?
[[Simple condition]]
// SQL: (type = 1) AND (status = 2). $cond = ['type' => 1, 'status' => 2] // SQL:(id IN (1, 2, 3)) AND (status = 2) $cond = ['id' => [1, 2, 3], 'status' => 2] //SQL:status IS NULL $cond = ['status' => null]
[and]: Combine different conditions together, usage example:
//SQL:`id=1 AND id=2` $cond = ['and', 'id=1', 'id=2'] //SQL:`type=1 AND (id=1 OR id=2)` $cond = ['and', 'type=1', ['or', 'id=1', 'id=2']] //SQL:`type=1 AND (id=1 OR id=2)` //此写法'='可以换成其他操作符,例:in like != >=等 $cond = [ 'and', ['=', 'type', 1], [ 'or', ['=', 'id', '1'], ['=', 'id', '2'], ] ]
[[or]]:
/SQL:`(type IN (7, 8, 9) OR (id IN (1, 2, 3)))` $cond = ['or', ['type' => [7, 8, 9]], ['id' => [1, 2, 3]]
[[not]]:
//SQL:`NOT (attribute IS NULL)` $cond = ['not', ['attribute' => null]]
[[between]]: not between has the same usage
//SQL:`id BETWEEN 1 AND 10` $cond = ['between', 'id', 1, 10]
[[in]]: not in has the same usage
//SQL:`id IN (1, 2, 3)` $cond = ['in', 'id', [1, 2, 3]] or $cond = ['id'=>[1, 2, 3]]
//IN条件也适用于多字段 $cond = ['in', ['id', 'name'], [['id' => 1, 'name' => 'foo'], ['id' => 2, 'name' => 'bar']]] //也适用于内嵌sql语句 $cond = ['in', 'user_id', (new Query())->select('id')->from('users')->where(['active' => 1])]
[[like]]:
//SQL:`name LIKE '%tester%'` $cond = ['like', 'name', 'tester'] //SQL:`name LIKE '%test%' AND name LIKE '%sample%'` $cond = ['like', 'name', ['test', 'sample']] //SQL:`name LIKE '%tester'` $cond = ['like', 'name', '%tester', false]
[[exists]]: not exists usage is similar to
//SQL:EXISTS (SELECT "id" FROM "users" WHERE "active"=1) $cond = ['exists', (new Query())->select('id')->from('users')->where(['active' => 1])]
In addition, you can specify any operator as follows:
//SQL:`id >= 10` $cond = ['>=', 'id', 10] //SQL:`id != 10` $cond = ['!=', 'id', 10]
PHP Chinese website, There are a large number of free Yii introductory tutorials, everyone is welcome to learn!
The above is the detailed content of How to conditionally query in yii framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
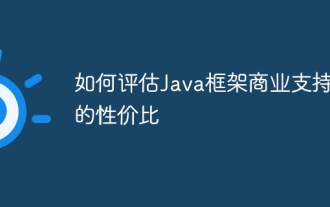
Evaluating the cost/performance of commercial support for a Java framework involves the following steps: Determine the required level of assurance and service level agreement (SLA) guarantees. The experience and expertise of the research support team. Consider additional services such as upgrades, troubleshooting, and performance optimization. Weigh business support costs against risk mitigation and increased efficiency.

The lightweight PHP framework improves application performance through small size and low resource consumption. Its features include: small size, fast startup, low memory usage, improved response speed and throughput, and reduced resource consumption. Practical case: SlimFramework creates REST API, only 500KB, high responsiveness and high throughput
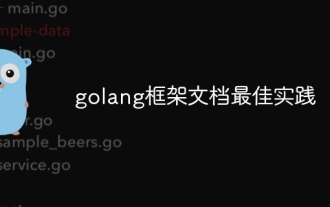
Writing clear and comprehensive documentation is crucial for the Golang framework. Best practices include following an established documentation style, such as Google's Go Coding Style Guide. Use a clear organizational structure, including headings, subheadings, and lists, and provide navigation. Provides comprehensive and accurate information, including getting started guides, API references, and concepts. Use code examples to illustrate concepts and usage. Keep documentation updated, track changes and document new features. Provide support and community resources such as GitHub issues and forums. Create practical examples, such as API documentation.
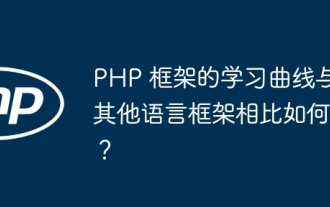
The learning curve of a PHP framework depends on language proficiency, framework complexity, documentation quality, and community support. The learning curve of PHP frameworks is higher when compared to Python frameworks and lower when compared to Ruby frameworks. Compared to Java frameworks, PHP frameworks have a moderate learning curve but a shorter time to get started.
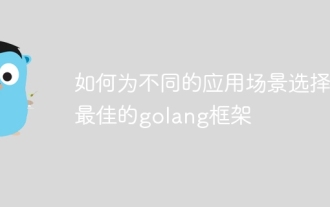
Choose the best Go framework based on application scenarios: consider application type, language features, performance requirements, and ecosystem. Common Go frameworks: Gin (Web application), Echo (Web service), Fiber (high throughput), gorm (ORM), fasthttp (speed). Practical case: building REST API (Fiber) and interacting with the database (gorm). Choose a framework: choose fasthttp for key performance, Gin/Echo for flexible web applications, and gorm for database interaction.
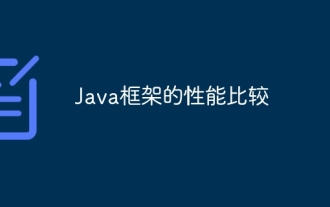
According to benchmarks, for small, high-performance applications, Quarkus (fast startup, low memory) or Micronaut (TechEmpower excellent) are ideal choices. SpringBoot is suitable for large, full-stack applications, but has slightly slower startup times and memory usage.
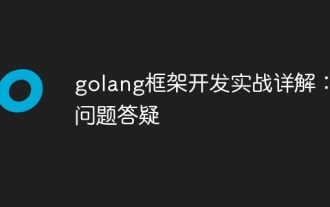
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
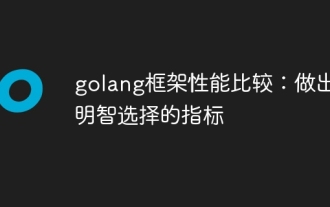
When choosing a Go framework, key performance indicators (KPIs) include: response time, throughput, concurrency, and resource usage. By benchmarking and comparing frameworks' KPIs, developers can make informed choices based on application needs, taking into account expected load, performance-critical sections, and resource constraints.
