ThinkPHP: Correct use of Db classes and models
It is found that many developers do not understand the correct usage posture of Db and model when using ThinkPHP5.*, especially in version 5.1. If you do not use the following correct posture, there are It is very likely that you will step into a trap.
The correct posture of Db
The following is the officially recommended usage of the Db class (that is, static method calls every time)
// 查询单个数据 Db::name('user')->where('id', 1)->find(); // 查询多个数据 Db::name('user')->where('id', '>', 1)->select(); // 写入新的数据 Db::name('user')->insert(['name' => '张三']); // 更新数据 Db::name('user')->where('id', 1)->update(['name' => '李四']); // 删除数据 Db::name('user')->delete(1);
Many developers To simplify the code, readers like to use the following code.
However, never use the code below in 5.1!
// 错误的用法 $user = Db::name('user'); // 查询单个数据 $user->where('id', 1)->find(); // 查询多个数据 $user->where('id', '>', 1)->select(); // 写入新的数据 $user->insert(['name' => '张三']); // 更新数据 $user->update(['name' => '李四']); // 删除数据 $user->delete(1);
Even using the helper function is still not recommended!
// 仍然是错误的用法 // 查询单个数据 db('user')->where('id', 1)->find(); // 查询多个数据 db('user')->where('id', '>', 1)->select(); // 写入新的数据 db('user')->insert(['name' => '张三']); // 更新数据 db('user')->update(['name' => '李四']); // 删除数据 db('user')->delete(1);
Many developers may wonder why it is wrong usage? The results I used are obviously fine, right? This just means that you haven’t stepped into the trap yet.
The real reason is that version 5.1 will not clear the previous query conditions after each query (5.0 will clear them every time), so the following usage is valid.
$user = Db::name('user'); // 查询分数大于80分的用户总数 $count = $user->where('score', '>', 80)->count(); // 查询分数大于80分的用户数据 $user->select();
You should understand after seeing this that when you use the same database query object instance, the query conditions will always be retained (that is, it will cause subsequent query conditions to be confused), and if you Multiple operations using helper functions or manual instantiation will be the same object instance, unless you manually clear it as below.
$user = Db::name('user'); // 查询分数大于80分的用户总数 $count = $user->where('score', '>', 80)->count(); // 清除查询条件(但不包括排序或者字段等信息) $user->removeOption('where'); // 查询所有用户数据 并按分数倒序排列 $user->order('score', 'desc')->select(); // 清除所有查询条件 $user->removeOption(); // 查询分数等于100的用户 $user->where('score', 100)->select();
Best practice: Use a new Db static query every time
The correct posture of the model
The design of the model In fact, just like Db, there is basically no need to manually instantiate it.
// 写入新的数据 $user = User::create(['name' => '张三']); // 更新数据 $user->update(['name' => '李四']); // 查询单个数据 $user = User::get(1); // 删除当前模型数据 $user->delete();
In the above code, we do not use any instantiation code, but use static method operations. The instantiation of the model is automatically completed by the system when querying or writing data. If you instantiate the model manually, it will cause the cost of repeated instantiation of the model.
Not recommended usage:
$user = new User; // 写入新的数据 $user->name = '张三'; $user->save();
$user = new User; $user->find(1); echo $user->name;
Recommended usage:
// 写入新的数据 User::create(['name' => '张三']); $user = User::get(1); echo $user->name;
So, please do not instantiate the model manually, and it is not recommended to use the model helper function.
Best practice: use static methods for both model query and creation
Now, do you understand the correct posture for using Db classes and models?
PHP Chinese website has a large number of free ThinkPHP introductory tutorials, everyone is welcome to learn!
This article is reproduced from: https://blog.thinkphp.cn/810719
The above is the detailed content of ThinkPHP: Correct use of Db classes and models. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


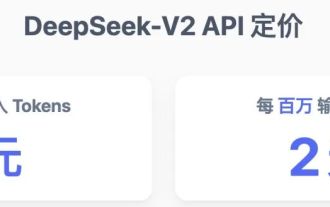
Imagine an artificial intelligence model that not only has the ability to surpass traditional computing, but also achieves more efficient performance at a lower cost. This is not science fiction, DeepSeek-V2[1], the world’s most powerful open source MoE model is here. DeepSeek-V2 is a powerful mixture of experts (MoE) language model with the characteristics of economical training and efficient inference. It consists of 236B parameters, 21B of which are used to activate each marker. Compared with DeepSeek67B, DeepSeek-V2 has stronger performance, while saving 42.5% of training costs, reducing KV cache by 93.3%, and increasing the maximum generation throughput to 5.76 times. DeepSeek is a company exploring general artificial intelligence
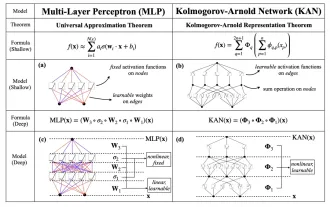
Earlier this month, researchers from MIT and other institutions proposed a very promising alternative to MLP - KAN. KAN outperforms MLP in terms of accuracy and interpretability. And it can outperform MLP running with a larger number of parameters with a very small number of parameters. For example, the authors stated that they used KAN to reproduce DeepMind's results with a smaller network and a higher degree of automation. Specifically, DeepMind's MLP has about 300,000 parameters, while KAN only has about 200 parameters. KAN has a strong mathematical foundation like MLP. MLP is based on the universal approximation theorem, while KAN is based on the Kolmogorov-Arnold representation theorem. As shown in the figure below, KAN has
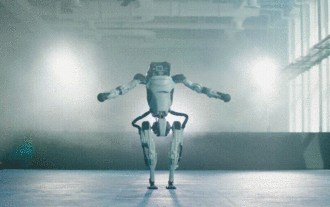
Boston Dynamics Atlas officially enters the era of electric robots! Yesterday, the hydraulic Atlas just "tearfully" withdrew from the stage of history. Today, Boston Dynamics announced that the electric Atlas is on the job. It seems that in the field of commercial humanoid robots, Boston Dynamics is determined to compete with Tesla. After the new video was released, it had already been viewed by more than one million people in just ten hours. The old people leave and new roles appear. This is a historical necessity. There is no doubt that this year is the explosive year of humanoid robots. Netizens commented: The advancement of robots has made this year's opening ceremony look like a human, and the degree of freedom is far greater than that of humans. But is this really not a horror movie? At the beginning of the video, Atlas is lying calmly on the ground, seemingly on his back. What follows is jaw-dropping
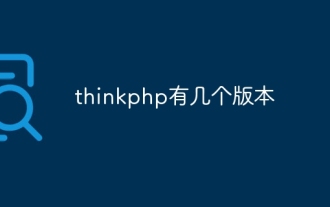
ThinkPHP has multiple versions designed for different PHP versions. Major versions include 3.2, 5.0, 5.1, and 6.0, while minor versions are used to fix bugs and provide new features. The latest stable version is ThinkPHP 6.0.16. When choosing a version, consider the PHP version, feature requirements, and community support. It is recommended to use the latest stable version for best performance and support.
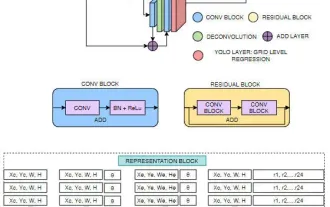
Target detection is a relatively mature problem in autonomous driving systems, among which pedestrian detection is one of the earliest algorithms to be deployed. Very comprehensive research has been carried out in most papers. However, distance perception using fisheye cameras for surround view is relatively less studied. Due to large radial distortion, standard bounding box representation is difficult to implement in fisheye cameras. To alleviate the above description, we explore extended bounding box, ellipse, and general polygon designs into polar/angular representations and define an instance segmentation mIOU metric to analyze these representations. The proposed model fisheyeDetNet with polygonal shape outperforms other models and simultaneously achieves 49.5% mAP on the Valeo fisheye camera dataset for autonomous driving
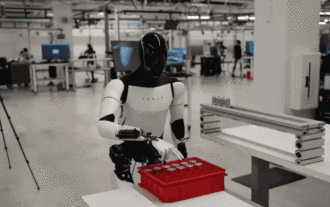
The latest video of Tesla's robot Optimus is released, and it can already work in the factory. At normal speed, it sorts batteries (Tesla's 4680 batteries) like this: The official also released what it looks like at 20x speed - on a small "workstation", picking and picking and picking: This time it is released One of the highlights of the video is that Optimus completes this work in the factory, completely autonomously, without human intervention throughout the process. And from the perspective of Optimus, it can also pick up and place the crooked battery, focusing on automatic error correction: Regarding Optimus's hand, NVIDIA scientist Jim Fan gave a high evaluation: Optimus's hand is the world's five-fingered robot. One of the most dexterous. Its hands are not only tactile
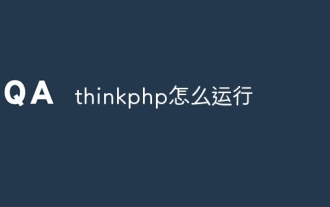
Steps to run ThinkPHP Framework locally: Download and unzip ThinkPHP Framework to a local directory. Create a virtual host (optional) pointing to the ThinkPHP root directory. Configure database connection parameters. Start the web server. Initialize the ThinkPHP application. Access the ThinkPHP application URL and run it.
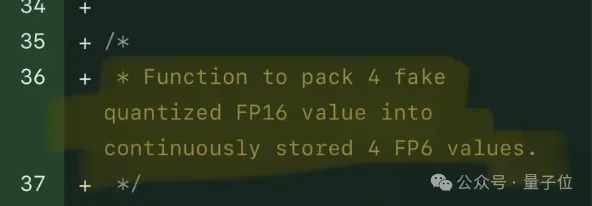
FP8 and lower floating point quantification precision are no longer the "patent" of H100! Lao Huang wanted everyone to use INT8/INT4, and the Microsoft DeepSpeed team started running FP6 on A100 without official support from NVIDIA. Test results show that the new method TC-FPx's FP6 quantization on A100 is close to or occasionally faster than INT4, and has higher accuracy than the latter. On top of this, there is also end-to-end large model support, which has been open sourced and integrated into deep learning inference frameworks such as DeepSpeed. This result also has an immediate effect on accelerating large models - under this framework, using a single card to run Llama, the throughput is 2.65 times higher than that of dual cards. one
