ThinkPHP: The third of three powerful tools for models (getter)
Define the getter
The function of the getter is to automatically process the (original) data of the model object . A getter corresponds to a special method of the model (the method must be of public type). The method naming convention is:
getFieldNameAttr
FieldName is the camel case conversion of the data table field Or a field that does not exist in your data table (pay attention to understanding the following sentence). The following is a typical getter definition:
<?php namespace app\index\model; use think\Model; class User extends Model { public function getUserTypeAttr($value, $data) { $type = [0 => '普通', 1 => 'VIP', 2 => '黄金', 3 => '白金', 4 => '钻石']; return $type[$value]; } }
You need to define a corresponding getter for each data field that requires output conversion processing. But the field name of the getter does not have to be consistent with the field name of the data table. For example, if I want to define a getter named getTypeAttr for the user_type field, it is also allowed, but it should be noted that the first one passed into the getter at this time The parameter must have no value (because there is no corresponding data table field data), and you can only get the data you need through the second parameter.
<?php namespace app\index\model; use think\Model; class User extends Model { public function getTypeAttr($value, $data) { $type = [0 => '普通', 1 => 'VIP', 2 => '黄金', 3 => '白金', 4 => '钻石']; return $type[$data['user_type']]; } }
Of course, in a more rigorous case, you also need to determine whether $data['user_type'] exists, which will be skipped for now.
Note that the data data of the second parameter may itself have been processed by the getter (if you define the relevant getter).
Why do we need to define a getter that is inconsistent with the datagram field? The most obvious benefit is the ability to differentiate between different fields to obtain raw data and processed data. In fact, there are many reasons for you to define some field getters that do not exist in the data table. This is precisely the charm of getters.
It can be seen that the definition of the getter itself is not difficult. The key lies in the acquisition logic in the method, which is the most important thing to pay attention to in practical applications.
Call the getter
After defining the getter, it will be automatically triggered in the following situations:
·The data object value operation of the model (such as $model->field_name);
·The serialized output operation of the model (such as $model-> toArray() or toJson());
##·Explicitly call the getAttr method (for example $model->getAttr('field_name'));
The first two are actually implemented by calling the last one. The most important thing is to understand the first one. When we obtain the value of a model object, we generally use the following method:$user = User::get(1); echo $user->name; echo $user->user_type;
·Step 1 - If the query result contains the field data, retrieve the original data, otherwise go to step 2;
·Step 2 - Check whether the getter (including dynamic getter) of this field is defined. If so, call the getter to return the result. If not, go to step 3;
·Step 3 - Check whether the field type conversion is defined, if so, perform conversion processing and return the result, if not, proceed to step 4;
·Step 4 - If it is a system time field, the time will be formatted automatically and the result will be returned, otherwise go to step 5;
·Step 5 - If the field data is not included in the first step of the check, check whether there is an associated attribute definition, and if so, obtain the data through the associated relationship and Returns the result, otherwise throws a property undefined exception.
For the detailed code of the above five steps, if you are interested, you can directly refer to the getAttr method code of think\model\concern\Attribute.To put it simply, when you get $user->user_type, you will check whether the relevant getter is defined, regardless of whether the user_type field is a real data table field. But in many cases, you will not get the model data one by one, but return the entire model data to the client or template.
public function index() { $user = User::get(1); return json($user); }
public function index() { $user = User::get(1); return json($user->append(['type'])); }
public function index() { $users = User::all(); return json($users->append(['type'])); }
In addition to the append method, we also support using the hidden method to temporarily hide some data.
Get original data
有些情况下,除了要获取处理过的数据外,还需要获取原始数据以便应对不同的需求。
如果你的获取器都是用的区分于实际数据表字段的额外属性字段,那么这个问题本身已经解决了。所以我们主要讨论的是当你的获取器属性和数据表字段一致的情况下,该如何获取原始数据。
一个最简单的办法是使用getData方法:
$user = User::get(1); // 获取user_type获取器数据 echo $user->user_type; // 获取原始的user_type数据 echo $user->getData('user_type'); // 获取全部原始数据 dump($user->getData());
动态获取器
前面我们提到过动态获取器的概念,动态获取器就是不需要在模型类里面定义获取器方法,而是在查询的时候使用闭包来定义一个字段的获取器对数据进行统一的处理。
User::withAttr('name', function($value, $data) { return strtolower($value); })->select();
如果你需要定义多个动态获取器,多次调用withAttr方法就行。
动态获取器的意义除了可以不用在模型里面定义获取器方法之外,还可以起到覆盖已经定义的获取器的作用,并且动态获取器可以支持Db类操作,弥补了Db操作不能使用获取器的缺憾,具体就看自己的需求来选择了。
Db::name('user')->withAttr('name', function($value, $data) { return strtolower($value); })->select();
总结
无论是获取器,还是之前提的修改器、搜索器,其作用无非是把你的模型工作细化和拆分,这样代码和逻辑也会更清晰,可维护性也大大增强,至于性能,从来不是模型首先考虑的。
PHP中文网,有大量免费的ThinkPHP入门教程,欢迎大家学习!
本文转自:https://blog.thinkphp.cn/825350
The above is the detailed content of ThinkPHP: The third of three powerful tools for models (getter). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


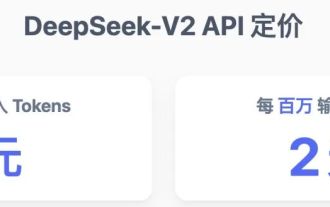
Imagine an artificial intelligence model that not only has the ability to surpass traditional computing, but also achieves more efficient performance at a lower cost. This is not science fiction, DeepSeek-V2[1], the world’s most powerful open source MoE model is here. DeepSeek-V2 is a powerful mixture of experts (MoE) language model with the characteristics of economical training and efficient inference. It consists of 236B parameters, 21B of which are used to activate each marker. Compared with DeepSeek67B, DeepSeek-V2 has stronger performance, while saving 42.5% of training costs, reducing KV cache by 93.3%, and increasing the maximum generation throughput to 5.76 times. DeepSeek is a company exploring general artificial intelligence
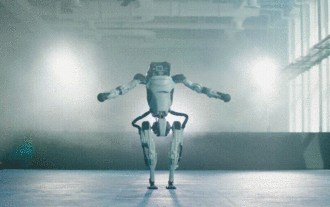
Boston Dynamics Atlas officially enters the era of electric robots! Yesterday, the hydraulic Atlas just "tearfully" withdrew from the stage of history. Today, Boston Dynamics announced that the electric Atlas is on the job. It seems that in the field of commercial humanoid robots, Boston Dynamics is determined to compete with Tesla. After the new video was released, it had already been viewed by more than one million people in just ten hours. The old people leave and new roles appear. This is a historical necessity. There is no doubt that this year is the explosive year of humanoid robots. Netizens commented: The advancement of robots has made this year's opening ceremony look like a human, and the degree of freedom is far greater than that of humans. But is this really not a horror movie? At the beginning of the video, Atlas is lying calmly on the ground, seemingly on his back. What follows is jaw-dropping
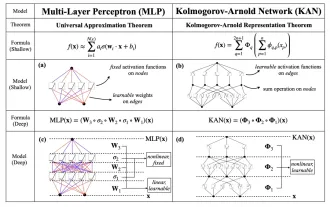
Earlier this month, researchers from MIT and other institutions proposed a very promising alternative to MLP - KAN. KAN outperforms MLP in terms of accuracy and interpretability. And it can outperform MLP running with a larger number of parameters with a very small number of parameters. For example, the authors stated that they used KAN to reproduce DeepMind's results with a smaller network and a higher degree of automation. Specifically, DeepMind's MLP has about 300,000 parameters, while KAN only has about 200 parameters. KAN has a strong mathematical foundation like MLP. MLP is based on the universal approximation theorem, while KAN is based on the Kolmogorov-Arnold representation theorem. As shown in the figure below, KAN has
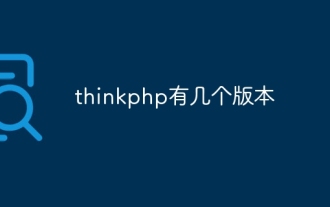
ThinkPHP has multiple versions designed for different PHP versions. Major versions include 3.2, 5.0, 5.1, and 6.0, while minor versions are used to fix bugs and provide new features. The latest stable version is ThinkPHP 6.0.16. When choosing a version, consider the PHP version, feature requirements, and community support. It is recommended to use the latest stable version for best performance and support.
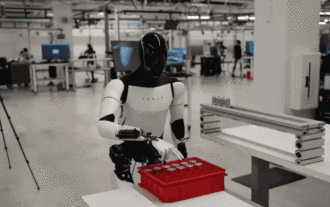
The latest video of Tesla's robot Optimus is released, and it can already work in the factory. At normal speed, it sorts batteries (Tesla's 4680 batteries) like this: The official also released what it looks like at 20x speed - on a small "workstation", picking and picking and picking: This time it is released One of the highlights of the video is that Optimus completes this work in the factory, completely autonomously, without human intervention throughout the process. And from the perspective of Optimus, it can also pick up and place the crooked battery, focusing on automatic error correction: Regarding Optimus's hand, NVIDIA scientist Jim Fan gave a high evaluation: Optimus's hand is the world's five-fingered robot. One of the most dexterous. Its hands are not only tactile
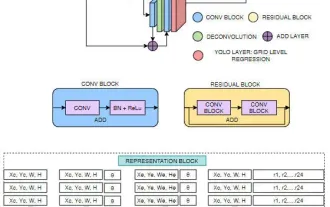
Target detection is a relatively mature problem in autonomous driving systems, among which pedestrian detection is one of the earliest algorithms to be deployed. Very comprehensive research has been carried out in most papers. However, distance perception using fisheye cameras for surround view is relatively less studied. Due to large radial distortion, standard bounding box representation is difficult to implement in fisheye cameras. To alleviate the above description, we explore extended bounding box, ellipse, and general polygon designs into polar/angular representations and define an instance segmentation mIOU metric to analyze these representations. The proposed model fisheyeDetNet with polygonal shape outperforms other models and simultaneously achieves 49.5% mAP on the Valeo fisheye camera dataset for autonomous driving
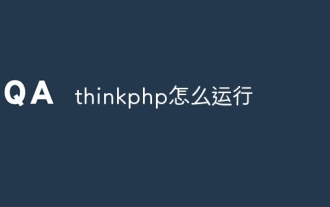
Steps to run ThinkPHP Framework locally: Download and unzip ThinkPHP Framework to a local directory. Create a virtual host (optional) pointing to the ThinkPHP root directory. Configure database connection parameters. Start the web server. Initialize the ThinkPHP application. Access the ThinkPHP application URL and run it.
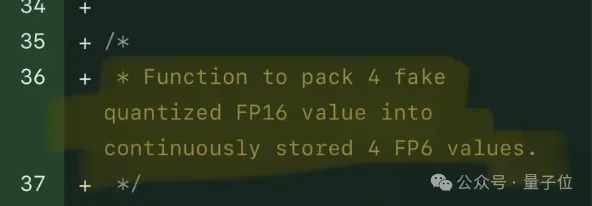
FP8 and lower floating point quantification precision are no longer the "patent" of H100! Lao Huang wanted everyone to use INT8/INT4, and the Microsoft DeepSpeed team started running FP6 on A100 without official support from NVIDIA. Test results show that the new method TC-FPx's FP6 quantization on A100 is close to or occasionally faster than INT4, and has higher accuracy than the latter. On top of this, there is also end-to-end large model support, which has been open sourced and integrated into deep learning inference frameworks such as DeepSpeed. This result also has an immediate effect on accelerating large models - under this framework, using a single card to run Llama, the throughput is 2.65 times higher than that of dual cards. one
