


Tween algorithm and buffering effects example code implemented in JavaScript_javascript skills
The example in this article describes the Tween algorithm and buffering effects implemented in JavaScript. Share it with everyone for your reference, the details are as follows:
Here is a demonstration of the Tween algorithm and JavaScript code for buffering special effects. You can use it to create many animation effects such as easing and spring. How to use the Tween algorithm of flash to make the Tween algorithm of js and use it to do some simple things. As for the easing effect, you will understand it after reading this code.
The screenshot of the running effect is as follows:
The online demo address is as follows:
http://demo.jb51.net/js/2015/js-tween-run-style-codes/
The specific code is as follows:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=gb2312" /> <title>Tween</title> </head> <body> <div style="padding-left:50px;"> <div style="position:relative; border:1px solid #000000; width:550px; height:50px;"> <div id="idMove" style="width:50px; height:50px; background:#930; position:absolute;"></div> </div> <div style="position:relative;width:550px;height:200px; margin-top:50px;"> <div id="idChart" style="border:1px solid #000;height:200px;"> </div> <div id="idLine" style="position:absolute;top:0;left:0;height:200px;width:1px;background:#000;"></div> </div> </div> <div> <p> Tween类型: <br /> <label> <input name="vTween" type="radio" value="Linear" checked="checked" /> Linear </label> <label> <input name="vTween" type="radio" value="Quad" /> Quadratic </label> <label> <input name="vTween" type="radio" value="Cubic" /> Cubic </label> <label> <input name="vTween" type="radio" value="Quart" /> Quartic </label> <label> <input name="vTween" type="radio" value="Quint" /> Quintic </label> <label> <input name="vTween" type="radio" value="Sine" /> Sinusoidal </label> <br /> <label> <input name="vTween" type="radio" value="Expo" /> Exponential </label> <label> <input name="vTween" type="radio" value="Circ" /> Circular </label> <label> <input name="vTween" type="radio" value="Elastic" /> Elastic </label> <label> <input name="vTween" type="radio" value="Back" /> Back </label> <label> <input name="vTween" type="radio" value="Bounce" /> Bounce </label> </p> <p> ease类型: <br /> <label> <input name="vEase" type="radio" value="easeIn" checked="checked" /> easeIn </label> <label> <input name="vEase" type="radio" value="easeOut" /> easeOut </label> <label> <input name="vEase" type="radio" value="easeInOut" /> easeInOut </label> </p> <input id="idSpeed" type="button" value="减慢速度" /> <input id="idRun" type="button" value=" Run " /> </div> <script> var Tween = { Linear: function(t,b,c,d){ return c*t/d + b; }, Quad: { easeIn: function(t,b,c,d){ return c*(t/=d)*t + b; }, easeOut: function(t,b,c,d){ return -c *(t/=d)*(t-2) + b; }, easeInOut: function(t,b,c,d){ if ((t/=d/2) < 1) return c/2*t*t + b; return -c/2 * ((--t)*(t-2) - 1) + b; } }, Cubic: { easeIn: function(t,b,c,d){ return c*(t/=d)*t*t + b; }, easeOut: function(t,b,c,d){ return c*((t=t/d-1)*t*t + 1) + b; }, easeInOut: function(t,b,c,d){ if ((t/=d/2) < 1) return c/2*t*t*t + b; return c/2*((t-=2)*t*t + 2) + b; } }, Quart: { easeIn: function(t,b,c,d){ return c*(t/=d)*t*t*t + b; }, easeOut: function(t,b,c,d){ return -c * ((t=t/d-1)*t*t*t - 1) + b; }, easeInOut: function(t,b,c,d){ if ((t/=d/2) < 1) return c/2*t*t*t*t + b; return -c/2 * ((t-=2)*t*t*t - 2) + b; } }, Quint: { easeIn: function(t,b,c,d){ return c*(t/=d)*t*t*t*t + b; }, easeOut: function(t,b,c,d){ return c*((t=t/d-1)*t*t*t*t + 1) + b; }, easeInOut: function(t,b,c,d){ if ((t/=d/2) < 1) return c/2*t*t*t*t*t + b; return c/2*((t-=2)*t*t*t*t + 2) + b; } }, Sine: { easeIn: function(t,b,c,d){ return -c * Math.cos(t/d * (Math.PI/2)) + c + b; }, easeOut: function(t,b,c,d){ return c * Math.sin(t/d * (Math.PI/2)) + b; }, easeInOut: function(t,b,c,d){ return -c/2 * (Math.cos(Math.PI*t/d) - 1) + b; } }, Expo: { easeIn: function(t,b,c,d){ return (t==0) ? b : c * Math.pow(2, 10 * (t/d - 1)) + b; }, easeOut: function(t,b,c,d){ return (t==d) ? b+c : c * (-Math.pow(2, -10 * t/d) + 1) + b; }, easeInOut: function(t,b,c,d){ if (t==0) return b; if (t==d) return b+c; if ((t/=d/2) < 1) return c/2 * Math.pow(2, 10 * (t - 1)) + b; return c/2 * (-Math.pow(2, -10 * --t) + 2) + b; } }, Circ: { easeIn: function(t,b,c,d){ return -c * (Math.sqrt(1 - (t/=d)*t) - 1) + b; }, easeOut: function(t,b,c,d){ return c * Math.sqrt(1 - (t=t/d-1)*t) + b; }, easeInOut: function(t,b,c,d){ if ((t/=d/2) < 1) return -c/2 * (Math.sqrt(1 - t*t) - 1) + b; return c/2 * (Math.sqrt(1 - (t-=2)*t) + 1) + b; } }, Elastic: { easeIn: function(t,b,c,d,a,p){ if (t==0) return b; if ((t/=d)==1) return b+c; if (!p) p=d*.3; if (!a || a < Math.abs(c)) { a=c; var s=p/4; } else var s = p/(2*Math.PI) * Math.asin (c/a); return -(a*Math.pow(2,10*(t-=1)) * Math.sin( (t*d-s)*(2*Math.PI)/p )) + b; }, easeOut: function(t,b,c,d,a,p){ if (t==0) return b; if ((t/=d)==1) return b+c; if (!p) p=d*.3; if (!a || a < Math.abs(c)) { a=c; var s=p/4; } else var s = p/(2*Math.PI) * Math.asin (c/a); return (a*Math.pow(2,-10*t) * Math.sin( (t*d-s)*(2*Math.PI)/p ) + c + b); }, easeInOut: function(t,b,c,d,a,p){ if (t==0) return b; if ((t/=d/2)==2) return b+c; if (!p) p=d*(.3*1.5); if (!a || a < Math.abs(c)) { a=c; var s=p/4; } else var s = p/(2*Math.PI) * Math.asin (c/a); if (t < 1) return -.5*(a*Math.pow(2,10*(t-=1)) * Math.sin( (t*d-s)*(2*Math.PI)/p )) + b; return a*Math.pow(2,-10*(t-=1)) * Math.sin( (t*d-s)*(2*Math.PI)/p )*.5 + c + b; } }, Back: { easeIn: function(t,b,c,d,s){ if (s == undefined) s = 1.70158; return c*(t/=d)*t*((s+1)*t - s) + b; }, easeOut: function(t,b,c,d,s){ if (s == undefined) s = 1.70158; return c*((t=t/d-1)*t*((s+1)*t + s) + 1) + b; }, easeInOut: function(t,b,c,d,s){ if (s == undefined) s = 1.70158; if ((t/=d/2) < 1) return c/2*(t*t*(((s*=(1.525))+1)*t - s)) + b; return c/2*((t-=2)*t*(((s*=(1.525))+1)*t + s) + 2) + b; } }, Bounce: { easeIn: function(t,b,c,d){ return c - Tween.Bounce.easeOut(d-t, 0, c, d) + b; }, easeOut: function(t,b,c,d){ if ((t/=d) < (1/2.75)) { return c*(7.5625*t*t) + b; } else if (t < (2/2.75)) { return c*(7.5625*(t-=(1.5/2.75))*t + .75) + b; } else if (t < (2.5/2.75)) { return c*(7.5625*(t-=(2.25/2.75))*t + .9375) + b; } else { return c*(7.5625*(t-=(2.625/2.75))*t + .984375) + b; } }, easeInOut: function(t,b,c,d){ if (t < d/2) return Tween.Bounce.easeIn(t*2, 0, c, d) * .5 + b; else return Tween.Bounce.easeOut(t*2-d, 0, c, d) * .5 + c*.5 + b; } } } var $ = function (id) { return "string" == typeof id ? document.getElementById(id) : id; }; var Each = function(list, fun){ for (var i = 0, len = list.length; i < len; i++) { fun(list[i], i); } }; var fun, iChart = 550, iDuration = 100; function Move(){ var oM = $("idMove").style, oL = $("idLine").style, t=0, c=500, d=iDuration; oM.left=oL.left="0px"; clearTimeout(Move._t); function _run(){ if(t<d){ t++; oM.left = Math.ceil(fun(t,0,c,d)) + "px"; oL.left = Math.ceil(Tween.Linear(t,0,iChart,d)) + "px"; Move._t = setTimeout(_run, 10); }else{ oM.left = c + "px"; oL.left = iChart + "px"; } } _run(); } function Chart(){ var a = []; for (var i = 0; i < iChart; i++) { a.push('<div style="background-color:#f60;font-size:0;width:3px;height:3px;position:absolute;left:' + (i-1) + 'px;top:' + (Math.ceil(fun(i,200,-200,iChart))) + 'px;"><\/div>'); } $("idChart").innerHTML = a.join(""); } var arrTween = document.getElementsByName("vTween"); var arrEase = document.getElementsByName("vEase"); Each(arrTween, function(o){ o.onclick = function(){ SetFun(); Chart(); } }) Each(arrEase, function(o){ o.onclick = function(){ SetFun(); Chart(); } }) function SetFun(){ var sTween, sEase; Each(arrTween, function(o){ if(o.checked){ sTween = o.value; } }) Each(arrEase, function(o){ if(o.checked){ sEase = o.value; } }) fun = sTween == "Linear" ? Tween.Linear : Tween[sTween][sEase]; } $("idRun").onclick = function(){ SetFun(); Chart(); Move(); } $("idSpeed").onclick = function(){ if(iDuration == 100){ iDuration = 500; this.value = "加快速度"; }else{ iDuration = 100; this.value = "减慢速度"; } } </script> </body> </html>
I hope this article will be helpful to everyone in JavaScript programming.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
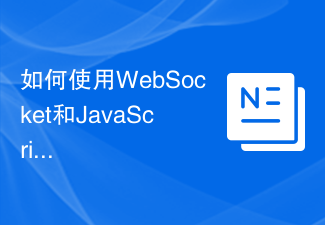
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
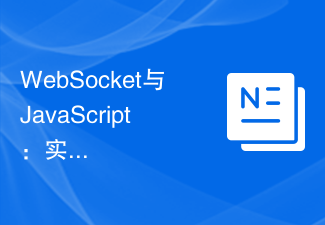
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
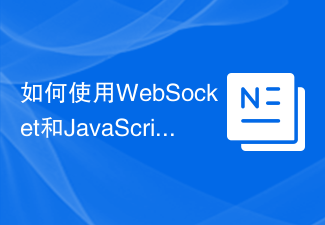
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
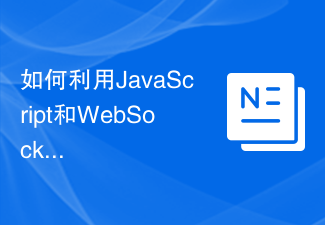
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
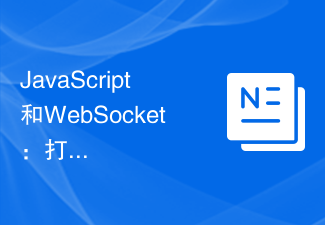
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
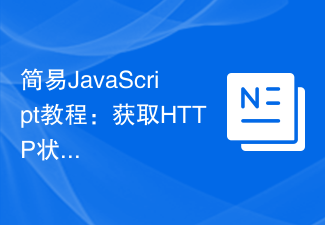
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
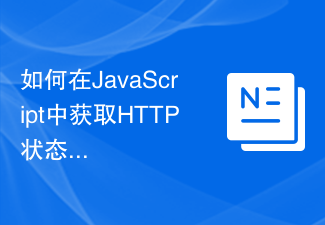
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
