What does typedef mean in c language
Typedef is a keyword in C language. Its function is to define a new name for a data type. The data types here include internal data types [int, char, etc.] and custom data types [stuct, etc.] .
Typedef is a common syntax in C/C. The function of typedef can be summed up into four types:
1. Define a type Name
In conventional applications, if you want to define two character pointers, write the following code: char *a, b
(1) char* a,b;
(2) char c='m';
(3) a=&c;
(4) b=&c;
The above code is wrong, only a is a character pointer, and b is still a character variable. Macro definition through #define is still invalid, because macro definition is only character replacement.
The following is feasible:
(1)typedef char* PCHAR;
(2)PCHAR pa, pb;
2. Cross-platform transplantation
When writing programs, if you take into account platform porting factors, you need to abstract the differences in the hardware layer from the code, such as the space occupied by variables, end mode, etc.
Consider a floating-point variable. On different hardware platforms, the space it occupies may be different. In this case, you can use typedef to define it in a separate header file. This header file is purely abstract. Hardware related content:
(1) typedef float REAL;
(2) typedef short int INT16;
(3) typedef int INT32
(4)...
In this case, if you consider transplanting the program in the future, you only need to modify the header file.
3. Give aliases to complex declarations
Complex declarations are in the form: void (*b[10]) (void (*)());
Meaning : First, *b[10] is a pointer array, and the ten elements in it are all pointers. What kind of pointer is it? It is a function pointer with a null return type and null formal parameters.
This kind of complex declaration can be simplified with typedef:
First: declare the function pointer behind:
(1) typedef void (pFunParam *)();
Then declare the previous pointer array:
(1) typedef void (*pFunx)(pFunParam);
The simplified version of the original declaration:
pFunx b[10];
During the writing process of this document, I referred to the online blog typedef usage
which mentioned a complex statement:
(1) doube (*)() (*e)[9];
However, this statement does not pass when compiled under gcc. According to the author's original intention, it seems that it should be declared like this:
(1 )double (*(*e)[9])();
e is a pointer to a 9-dimensional array. The array is a function pointer. The formal parameter of the function pointer is empty and the return type is double.
In this case, such typedef should be used to simplify the declaration:
typedef (*(*ptr)[9])();
Recommended tutorial: "c language tutorial》
The above is the detailed content of What does typedef mean in c language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


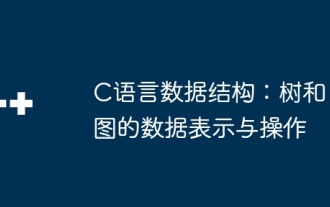
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
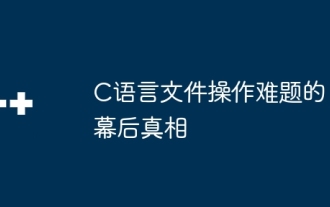
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
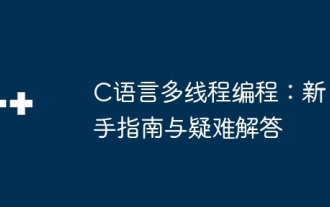
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
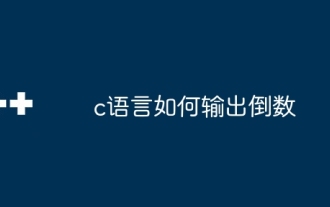
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
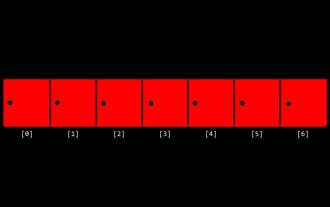
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
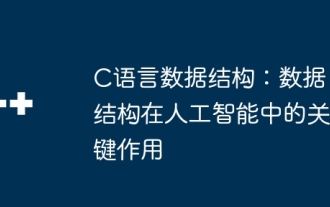
C Language Data Structure: Overview of the Key Role of Data Structure in Artificial Intelligence In the field of artificial intelligence, data structures are crucial to processing large amounts of data. Data structures provide an effective way to organize and manage data, optimize algorithms and improve program efficiency. Common data structures Commonly used data structures in C language include: arrays: a set of consecutively stored data items with the same type. Structure: A data type that organizes different types of data together and gives them a name. Linked List: A linear data structure in which data items are connected together by pointers. Stack: Data structure that follows the last-in first-out (LIFO) principle. Queue: Data structure that follows the first-in first-out (FIFO) principle. Practical case: Adjacent table in graph theory is artificial intelligence
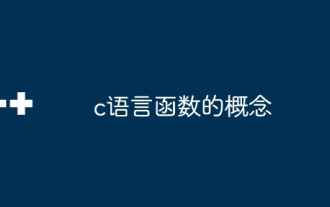
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
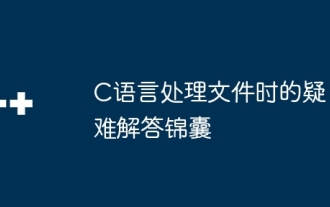
Troubleshooting Tips for C language processing files When processing files in C language, you may encounter various problems. The following are common problems and corresponding solutions: Problem 1: Cannot open the file code: FILE*fp=fopen("myfile.txt","r");if(fp==NULL){//File opening failed} Reason: File path error File does not exist without file read permission Solution: Check the file path to ensure that the file has check file permission problem 2: File reading failed code: charbuffer[100];size_tread_bytes=fread(buffer,1,siz
